Getting a List from Redis in Golang (Detailed Guide w/ Code Examples)
Use Case(s)
In Golang, you might use Redis for caching, session management, pub/sub systems, and more. One common requirement is to retrieve a list from Redis, which can be used as a queue or stack for task management.
Code Examples
Example 1: Using the go-redis
package. Firstly, you need to install the go-redis
package using go get github.com/go-redis/redis/v8
Then here is how you can get a list from Redis: CODE_BLOCK_PLACEHOLDER_0
This program connects to a local Redis instance, retrieves all elements (0 to -1
means all elements) from a list named mylist
, and prints them out.
Best Practices
- Always handle errors that can occur during the connection or retrieval process.
- If you have larger lists, consider fetching them in chunks rather than all at once to reduce memory usage.
- Be mindful of the index values; in Redis,
-1
indicates the end of the list.
Common Mistakes
- Ignoring errors or connection issues that may arise during development.
- Incorrectly assuming list indices, remember, Redis lists are zero-indexed.
FAQs
Q: How do I handle an empty list? A: If a list is empty, LRange
will return an empty slice with no error. You can check this by checking if the length of the returned slice is 0.
Q: Can I get a specific range of elements from a list in Redis using Golang? A: Yes, you can specify the start and stop parameters in the LRange
function to get a specific range of elements.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
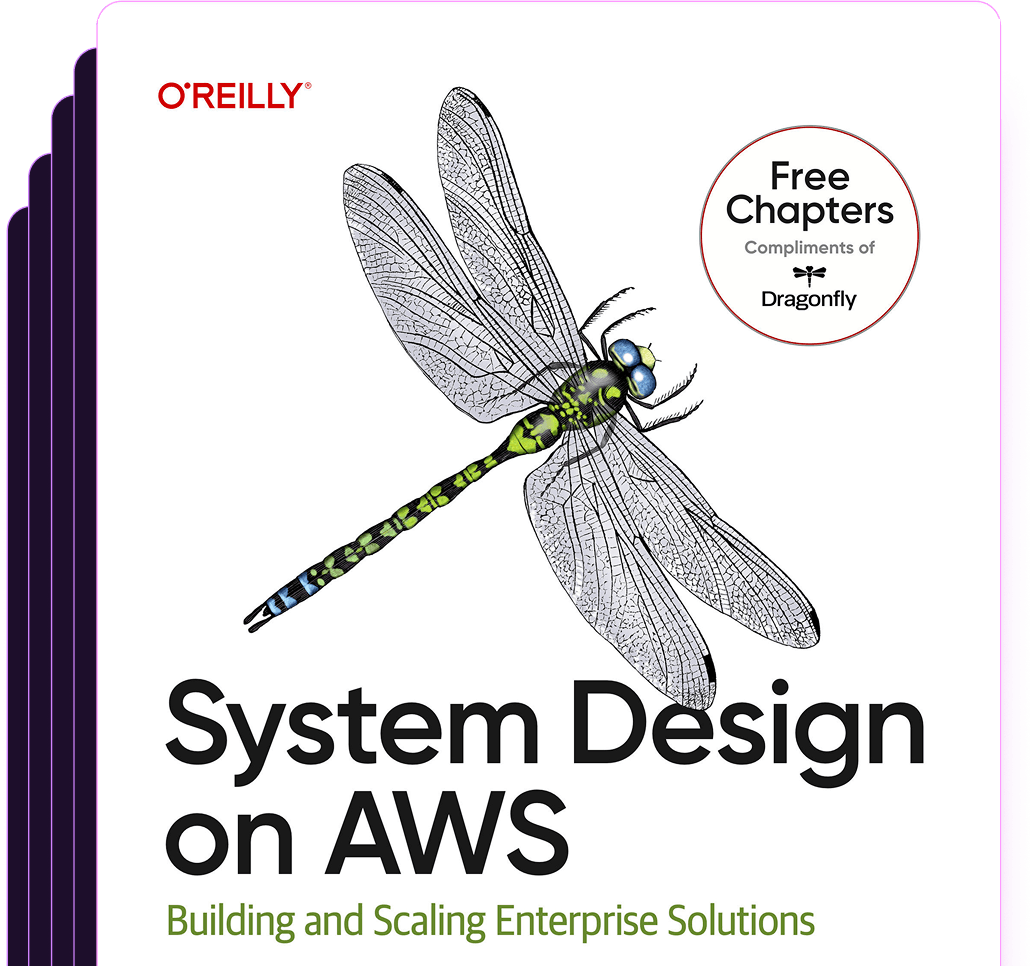
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost