Deleting a Redis Hash in Java (Detailed Guide w/ Code Examples)
Use Case(s)
Redis hashes are incredibly versatile and are commonly used to store objects. Deleting a Redis hash in Java is a common operation and can be needed for various reasons, such as dealing with obsolete data or managing storage.
Code Examples
Let's consider you're using Jedis, a popular Redis client for Java. Here's how you would typically delete a hash:
```
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args) {
// Connect to Redis server
Jedis jedis = new Jedis('localhost');
// Delete the entire hash
jedis.del('myhash');
}
}
```
In this example, 'myhash'
is the name of the hash we want to delete. The del
method deletes the entire hash along with all its fields and associated values.
Best Practices
- Always check that the hash you're trying to delete exists to avoid unnecessary operations.
- Consider using Redis transactions if you want to perform several operations atomically.
Common Mistakes
- Trying to delete a specific field using
del
. For deleting a specific field usehdel
instead.
FAQs
Q: What happens if I try to delete a non-existent hash?
A: The del
command will simply return 0 if the specified key doesn't exist.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
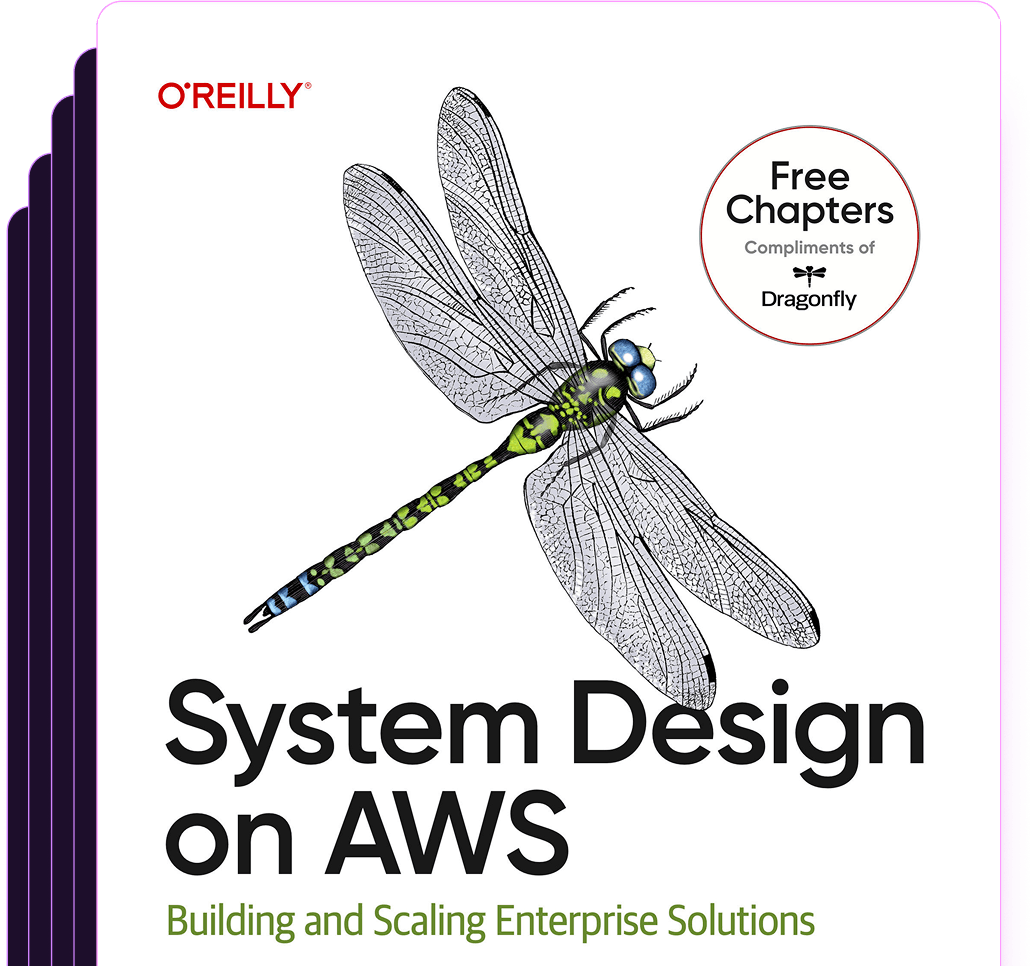
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost