Node Redis Delete All Keys (Detailed Guide w/ Code Examples)
Use Case(s)
In Node.js applications using Redis as a cache or session store, you may need to delete all keys in scenarios such as:
- Clearing the cache to refresh data.
- Deleting all sessions for system maintenance or security reasons.
Code Examples
- Using
flushdb
command:
const redis = require('redis');
const client = redis.createClient();
client.flushdb((err, succeeded) => {
console.log(succeeded); // will be true if success
});
flushdb
is a Redis command that deletes all keys from the current database. This method is handy when you want to clear your entire cache.
- Using
keys
anddel
commands:
const redis = require('redis');
const client = redis.createClient();
client.keys('*', (err, keys) => {
if (err) return console.log(err);
for(let i = 0; i < keys.length; i++) {
client.del(keys[i], function(err, reply) {
console.log(reply);
});
}
});
In this example, we first call the keys
command with the wildcard '*' to fetch all keys in the database. After that, we loop over the keys and delete each one using the del
command.
Best Practices
- Be cautious when using these commands, especially on production databases, as they will permanently delete all your keys.
- Avoid blocking Redis for a long time when deleting a large number of keys. Consider using the
UNLINK
command, which is a non-blocking alternative toDEL
.
Common Mistakes
- Not handling errors from
flushdb
,del
, orkeys
commands. - Using these commands on the wrong database or the production environment by mistake.
FAQs
Q: Can I delete keys matching a specific pattern? A: Yes, in the second code example, replace '' in the keys
method with your pattern. For example, 'session:' will match all keys that start with 'session:'.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
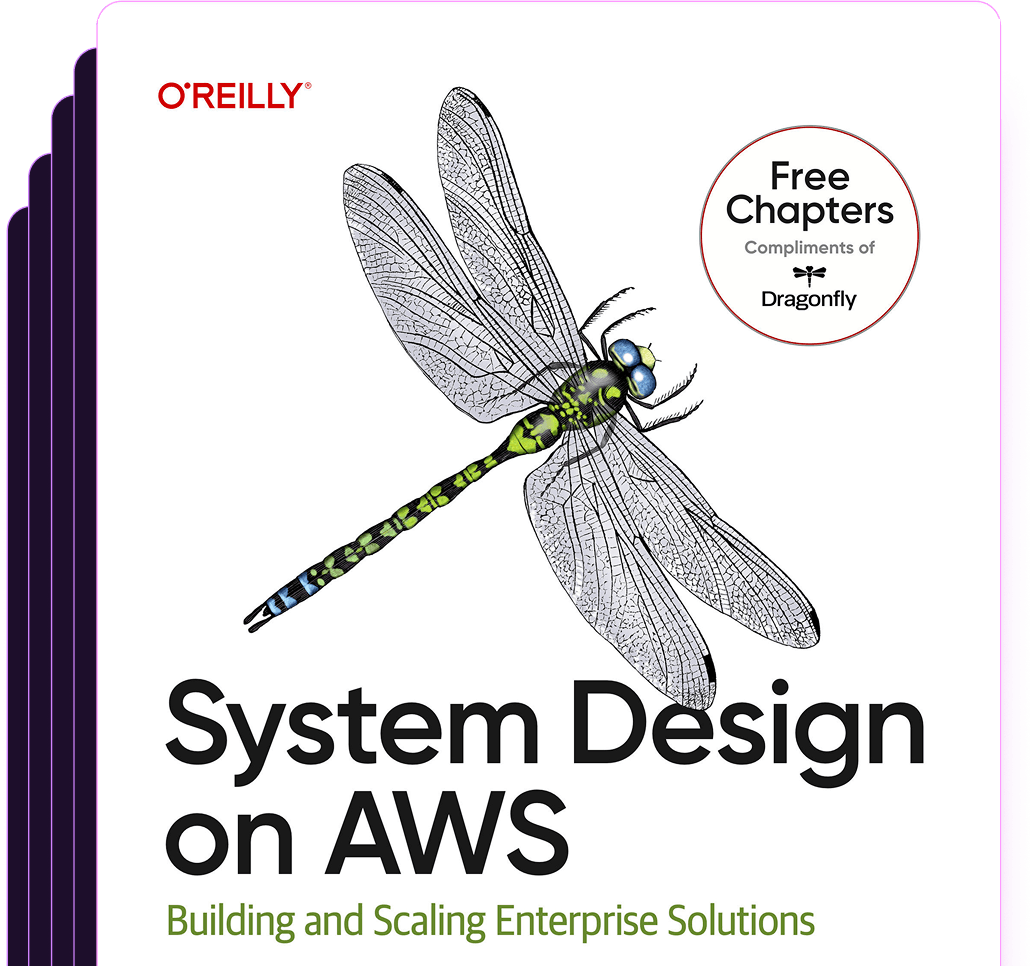
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost