Deleting Redis Keys by Pattern in PHP (Detailed Guide w/ Code Examples)
Use Case(s)
Deleting Redis keys by a pattern is commonly used when you need to remove multiple keys that share a common naming scheme. Typical examples would be session keys prefixed by user id or cache keys with a common prefix.
Code Examples
Let's assume we have a Redis instance and connection established via Predis client:
$client = new Predis\Client();
Example 1: Delete keys matching a certain pattern:
$keys = $client->keys('pattern*');
foreach ($keys as $key) {
$client->del($key);
}
In this example, we first get all keys matching the pattern 'pattern*' and then delete each key individually.
Example 2: If you want to delete keys across different databases (if your Redis setup has multiple databases), you need to select each database and perform the deletion operation:
for ($i = 0; $i < $numDatabases; $i++) {
$client->select($i);
$keys = $client->keys('pattern*');
foreach ($keys as $key) {
$client->del($key);
}
}
Best Practices
- Be very careful with the
keys
command in a production environment because it can potentially block the server while it's retrieving keys, especially when you have many keys. - Instead of using
del
, consider usingunlink
which is a non-blocking delete operation in Redis.
Common Mistakes
- Not properly defining the key pattern which can lead to deletion of unintended keys.
- Using
keys
command in production, it's better to usescan
for large datasets to avoid blocking the server.
FAQs
Q: Can I delete keys by pattern in one command?
A: Redis does not provide an atomic operation to delete keys by pattern. You need to get all keys by pattern first using keys
or scan
, then delete them individually.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
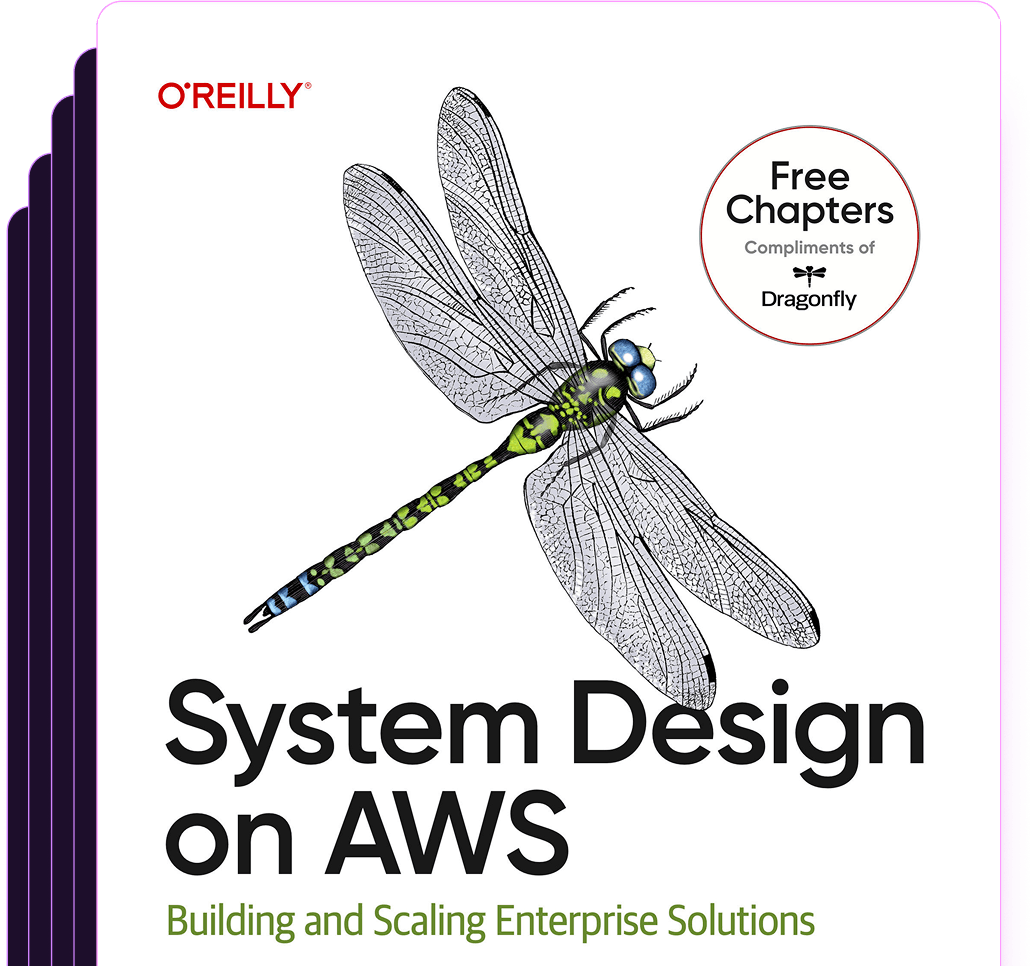
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost