Redis HMGET in PHP (Detailed Guide w/ Code Examples)
Use Case(s)
The HMGET
command in Redis is used when we need to retrieve multiple field values from a hash stored in Redis. This is particularly useful in cases where a single key is associated with numerous field-value pairs, such as storing user profiles where a username might be linked to attributes like email, age, and address.
Code Examples
Let's say you have a hash in Redis that represents a user profile with the key 'user:1' and fields 'name', 'email', and 'age'. Here's how you can retrieve these details using HMGET
in PHP:
<?php
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
// Fetches 'name', 'email', and 'age' of 'user:1'
$fields = $redis->hMGet('user:1', array('name', 'email', 'age'));
print_r($fields);
?>
This script will return an associative array containing the requested fields and their respective values.
Best Practices
- Prioritize the use of
HMGET
over multipleHGET
commands for efficiency when retrieving multiple fields. - Remember that
HMGET
will returnnull
for non-existing keys or fields.
Common Mistakes
- Trying to get values from a key that doesn't exist will not raise an error, but will return
null
for those fields. Make sure the key and fields exist before fetching. - Misunderstanding that
HMGET
only works on hashes. Trying to use it on other data types will result in an error.
FAQs
Q: What's the difference between HGET
and HMGET
in Redis?
A: While both are used to retrieve values from hashes, HGET
returns the value of a specific field, while HMGET
can return the values of multiple fields.
Q: Can I use HMGET
with keys that aren't hashes?
A: No, HMGET
is specifically designed for hash keys. Using it with other data types will result in an error.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
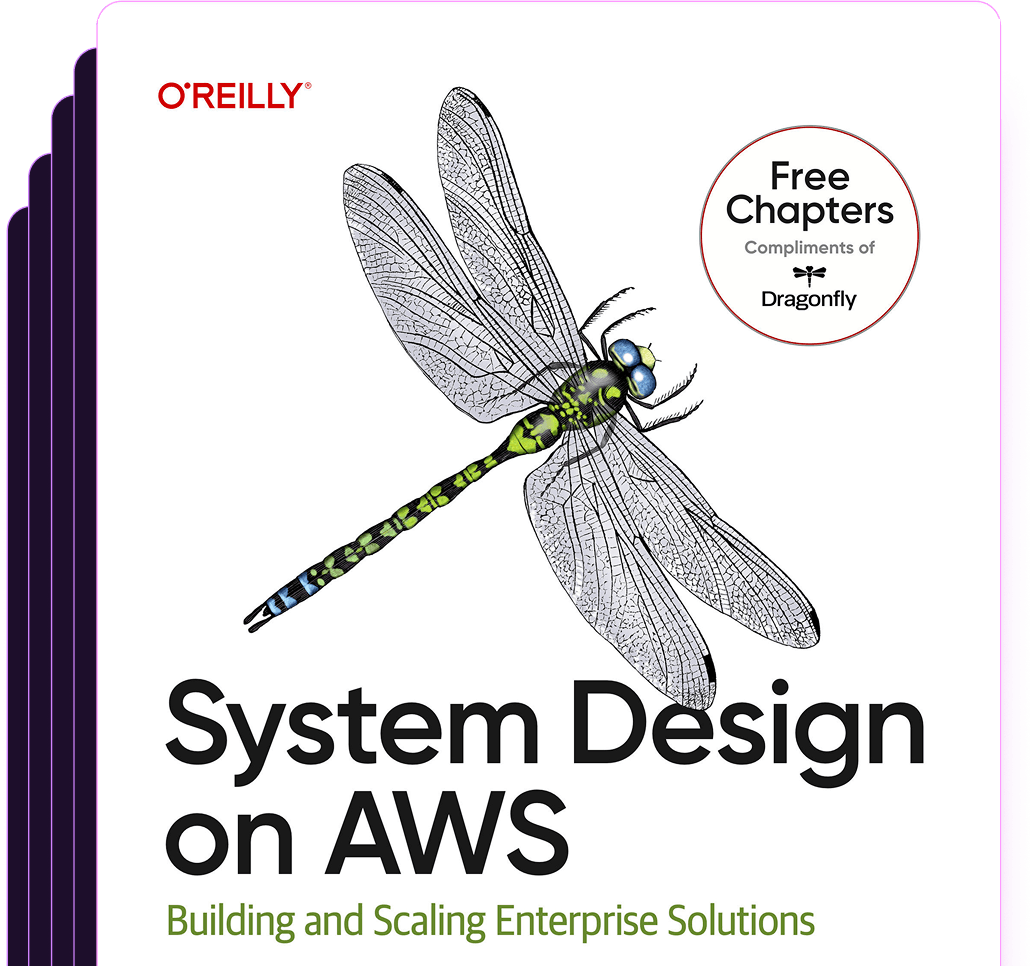
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost