Redis ZREM in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The ZREM
command in Redis is used to remove one or more members from a sorted set. Common use cases include:
- Real-time ranking systems where you need to remove outdated or irrelevant items.
- Leaderboards in gaming scenarios, where players might be removed due to inactivity.
- Managing sessions or tokens that are no longer valid and should not be considered in active sets.
Code Examples
Example 1: Removing a Single Member from a Sorted Set
In this example, we remove a single member from a sorted set named "highscores".
import redis
# Connect to Redis server
r = redis.Redis(host='localhost', port=6379, db=0)
# Adding some members to the sorted set for demonstration
r.zadd('highscores', {'Alice': 500, 'Bob': 400})
# Remove a member from the set
r.zrem('highscores', 'Bob')
# Display the updated sorted set
print(r.zrange('highscores', 0, -1, withscores=True))
Example 2: Removing Multiple Members from a Sorted Set
Here, we demonstrate how to remove multiple members from a sorted set at once.
import redis
# Connect to Redis server
r = redis.Redis(host='localhost', port=6379, db=0)
# Adding members to the sorted set
r.zadd('highscores', {'Alice': 500, 'Bob': 400, 'Charlie': 800})
# Remove multiple members from the set
r.zrem('highscores', 'Alice', 'Bob')
# Display the updated sorted set
print(r.zrange('highscores', 0, -1, withscores=True))
Best Practices
- Ensure the key exists and has the correct data type before attempting to remove members. Trying to remove members from a non-existing key or a key associated with a different data type might lead to errors.
- When working with large sorted sets, consider the performance implications of frequent updates, including additions and deletions.
Common Mistakes
- Forgetting to check if the key exists and is a sorted set before attempting a removal operation, which can lead to runtime errors.
- Misunderstanding the return value;
ZREM
returns the number of elements removed, not the current state of the set.
FAQs
Q: What happens if the member is not present in the sorted set? A: The ZREM
command will simply return 0 indicating that no members were removed.
Q: Can ZREM
be used on normal Redis sets or lists? A: No, ZREM
specifically works with sorted sets. For normal sets, the SREM
command is used; for lists, operations like LREM
would be applicable.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Redis ZCOUNT in Python
- Redis ZRANGEBYLEX in Python
- Redis ZRANGE in Python
- Redis ZREVRANGEBYSCORE in Python
- Redis ZREMRANGEBYSCORE in Python
- Redis ZREVRANGE in Python
- Redis ZUNIONSTORE in Python
- Redis ZRANK in Python
- Redis ZADD in Python
- Redis ZREVRANK in Python
- Redis ZSCORE in Python
- Redis ZRANGEBYSCORE in Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
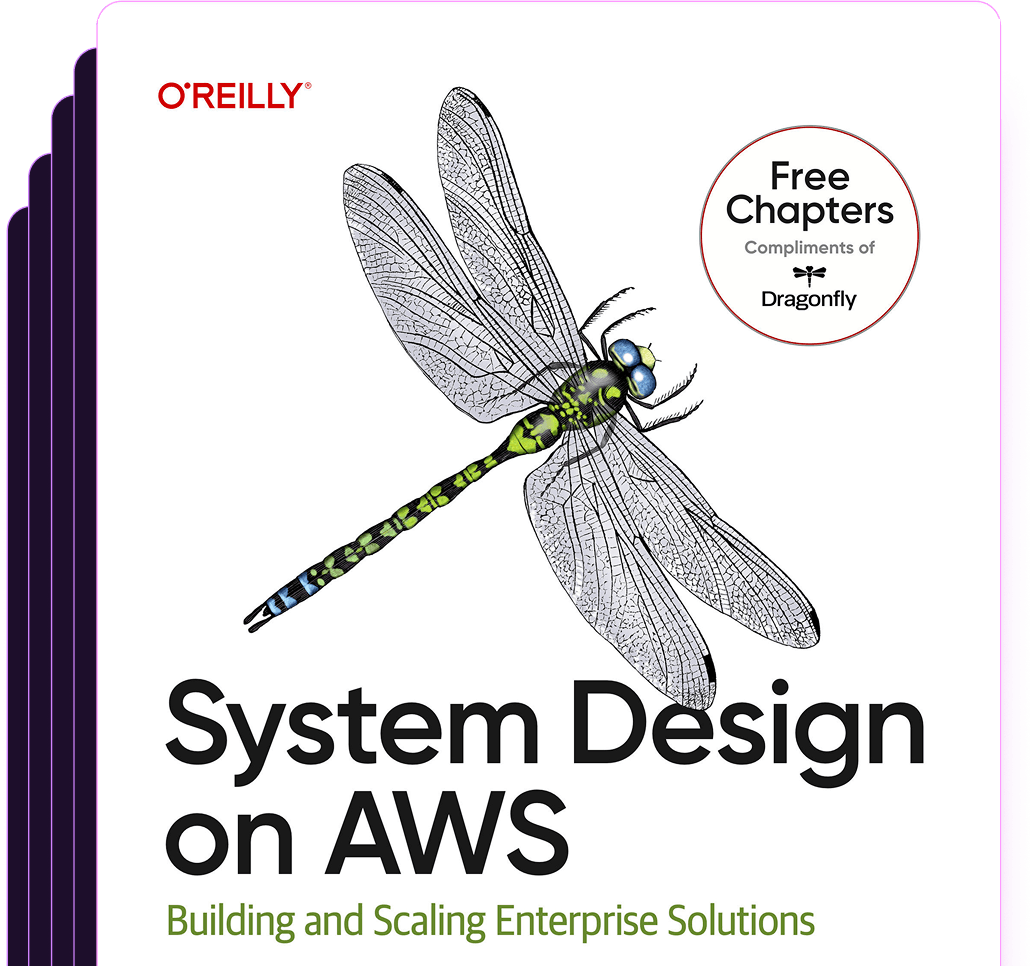
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost