Error: redis error server closed the connection
Solution
Understanding the "Redis Error: Server Closed the Connection"
This error occurs when the Redis server unexpectedly terminates the connection with the client. Common causes include:
- Network instability
- Redis server overload
- Connection timeout
- Firewall interference
- Misconfigured Redis settings
Step-by-Step Solutions to Resolve the Error
1. Check Redis Server Status
First, ensure the Redis server is running:
sudo systemctl status redis
If it's not running, start it:
sudo systemctl start redis
2. Verify Network Connectivity
Test the connection to your Redis server:
redis-cli -h <your_redis_host> -p <your_redis_port> ping
If this fails, check your firewall settings and network configuration.
3. Increase Max Connections
Edit the Redis configuration file:
sudo nano /etc/redis/redis.conf
Find and modify the maxclients
directive:
```
maxclients 10000
```
Save and restart Redis:
sudo systemctl restart redis
4. Adjust Timeout Settings
In the same configuration file, modify these settings:
```
timeout 300
tcp-keepalive 60
```
Restart Redis after changes.
5. Implement Connection Pooling
If using Node.js with ioredis
, for example:
const Redis = require('ioredis');
const pool = new Redis.Cluster([
{
port: 6379,
host: '127.0.0.1'
}
], {
redisOptions: {
maxRetriesPerRequest: 3,
connectTimeout: 10000
}
});
6. Enable Persistent Connections
In your application code, reuse connections instead of creating new ones for each operation.
7. Monitor Redis Performance
Use the Redis CLI to check performance:
redis-cli info
Look for high memory usage or slow response times.
8. Update Redis Client Library
Ensure you're using the latest version of your Redis client library. For example, with npm:
npm update redis
9. Enable Redis Logging
In redis.conf
, set:
```
loglevel notice
```
Check logs for specific error messages:
tail -f /var/log/redis/redis-server.log
10. Implement Reconnection Logic
In your application, add reconnection logic. For example, in Node.js:
const redis = new Redis({
host: 'your_redis_host',
retryStrategy(times) {
const delay = Math.min(times * 50, 2000);
return delay;
}
});
11. Consider Redis Cluster
For high-availability, set up a Redis Cluster:
- Configure multiple Redis instances
- Use the
redis-cli
to create the cluster - Update your application to use cluster-aware client
If these steps don't resolve the issue, consider upgrading your Redis server or consulting with a database administrator for more advanced troubleshooting.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Errors (with Solutions)
- could not connect to redis at 127.0.0.1:6379: connection refused
- redis.exceptions.responseerror: value is not an integer or out of range
- redis.exceptions.responseerror moved
- redis.exceptions.responseerror noauth authentication required
- redis-server failed to start advanced key-value store
- spring boot redis unable to connect to localhost 6379
- unable to configure redis to keyspace notifications
- redis.clients.jedis.exceptions.jedismoveddataexception
- could not get resource from pool redis
- failed to restart redis service unit redis service not found
- job for redis-server.service failed because a timeout was exceeded
- failed to start redis-server.service unit redis-server.service is masked
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
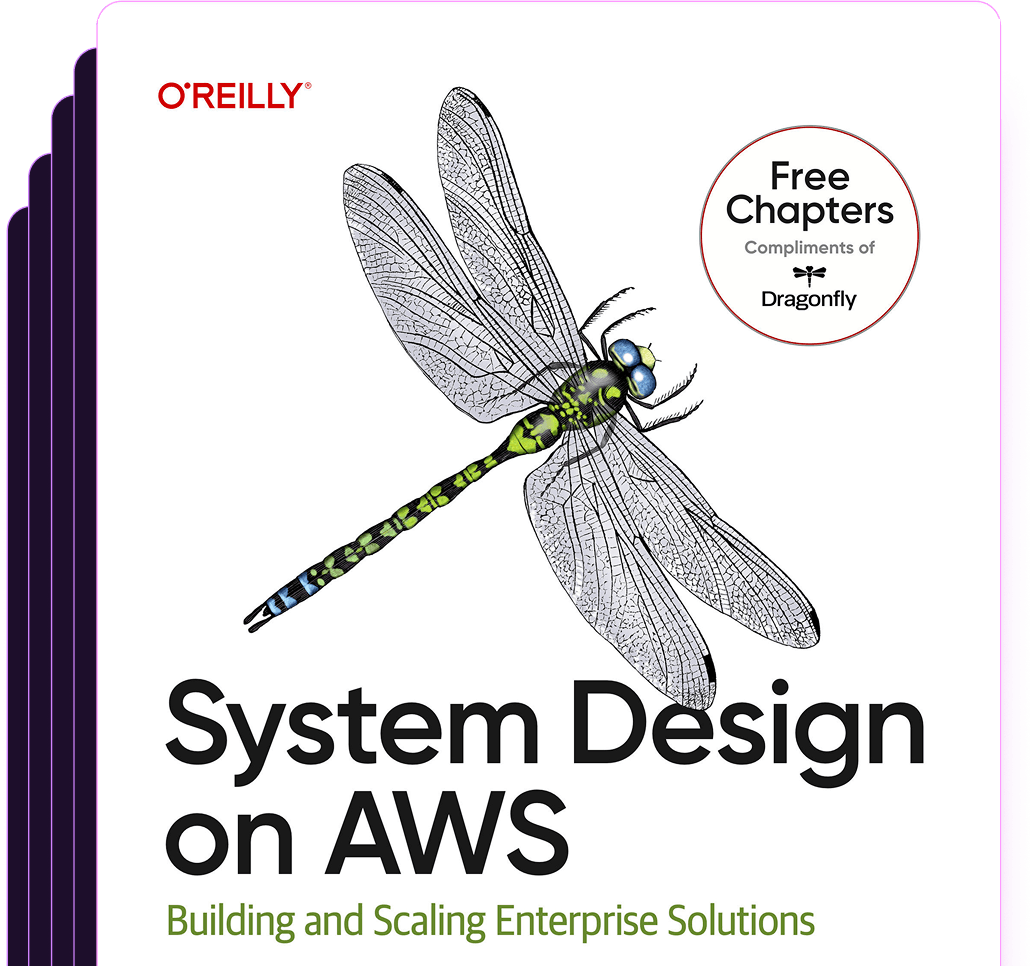
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost