Question: How do you design a database using key-value tables?
Answer
Key-value stores are a simplistic, yet powerful way to store and manage data. They work by storing data as a collection of key-value pairs, where each unique key is associated with one specific value. This model offers high performance and scalability for certain types of applications, notably those requiring rapid access to large amounts of unstructured data. Here's how to design a database using key-value tables:
Understanding Key-Value Stores
Before diving into design, it's essential to grasp the core concepts of key-value stores. They are optimized for scenarios where data access patterns are primarily through a unique key. They excel in use cases such as caching, session storage, and settings or preference storage.
Identify Suitable Use Cases
Key-value stores are not a one-size-fits-all solution. They are best suited for:
- Caching: Storing temporary, frequently accessed data.
- Session Storage: Managing user sessions in web applications.
- Configuration Data: Storing configuration settings that an application can read quickly.
- NoSQL Databases: As part of broader NoSQL solutions, where flexibility in data models is required.
Designing Key Structures
One of the most critical aspects of designing with key-value stores is devising an effective key naming and structure strategy. Keys should be constructed in a way that makes them both unique and easily queryable. Consider including identifiers, timestamps, or other relevant data in your keys to ensure they are descriptive and can be efficiently sorted or accessed.
Example:
\`\`\`
user:12345
session:abcd1234:user:12345
config:featureX:enabled
\`\`\`
Value Storage Strategies
Values in key-value stores can be simple strings, numbers, or more complex serialized objects like JSON or binary data. When designing your database, decide on the serialization format based on the data retrieval and processing needs. JSON is flexible and human-readable, but binary formats might offer better performance and space efficiency for complex objects.
Dealing with Scalability
Key-value stores are inherently scalable, especially if choosing a distributed key-value store system. However, plan for data partitioning and replication strategies upfront. Consistent hashing, sharding, and replication factors are crucial decisions to ensure data availability and performance at scale.
Example: Redis Implementation
Redis is a popular key-value store offering advanced data structures. Below is a simple example of setting and getting a key-value pair in Redis using Python.
\`\`\`python
import redis
Connect to Redis server
r = redis.Redis(host='localhost', port=6379, db=0)
Set a key
r.set('mykey', 'Hello World')
Get the key's value
print(r.get('mykey')) # Output: b'Hello World'
\`\`\`
Conclusion
Designing a database around key-value tables requires understanding both the limitations and strengths of key-value stores. By focusing on suitable use cases, carefully planning key structures, and considering scalability from the outset, key-value databases can provide fast, flexible, and scalable data storage solutions.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Key-Value Databases Questions (and Answers)
- What are the disadvantages of key-value databases?
- What are the advantages of a key-value database?
- Is MongoDB a key-value database?
- How fast are key-value databases?
- What are the differences between key-value stores and relational databases?
- What is the difference between key-value and document databases?
- What are the characteristics and features of key-value store databases?
- What are the differences between key-value databases and Cassandra?
- When should a key-value database not be used?
- Are key-value databases similar to tables in RDBMS?
- Is Redis a key-value store?
- How do key-value stores support secondary indexes?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
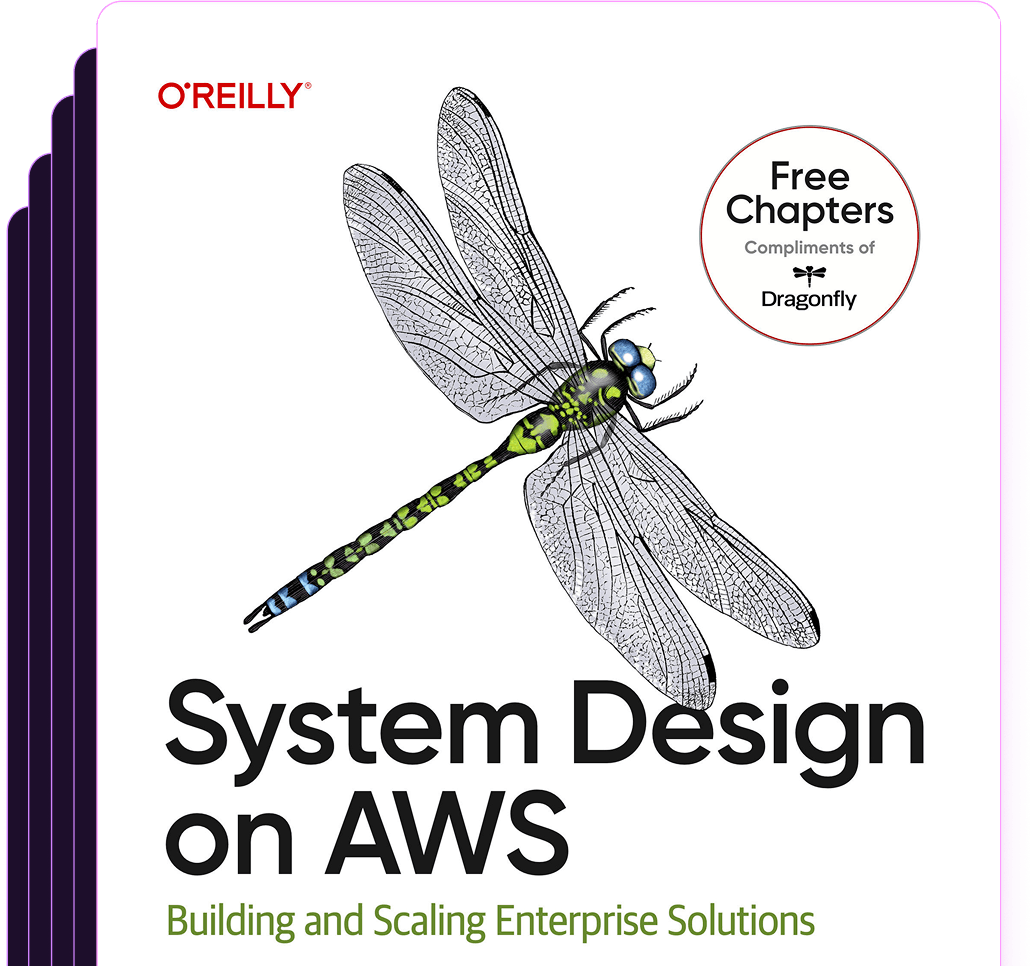
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost