Question: How do you create and use an array of objects in GameMaker?
Answer
In GameMaker, an array is a data structure that can hold a collection of values, which includes instances of objects. To create and use an array of objects, follow these steps:
- Creating an Array of Objects: You declare an array and then assign object instances to its elements. In GameMaker, object instances are referred to by their instance IDs, which are essentially numbers.
// Create an empty array
var objectArray = [];
// Add instances to the array
objectArray[0] = instance_create_layer(x, y, "Instances", obj_Enemy);
objectArray[1] = instance_create_layer(x, y, "Instances", obj_Player);
// ... and so on for as many objects as you need
- Accessing Array Elements: To access or modify an element within the array, you refer to it by its index.
// Access the first object in the array (index 0)
var firstObject = objectArray[0];
// Move the first object
firstObject.x += 10;
- Iterating Over an Array of Objects: If you want to perform operations on every object in the array, you use a loop.
// Loop through each object in the array
for (var i = 0; i < array_length(objectArray); ++i) {
var objInstance = objectArray[i];
// Perform some action with objInstance
objInstance.x += 5; // For example, move each object to the right
}
- Multidimensional Arrays: If necessary, you can also create multidimensional arrays to store objects, which can be useful for grid-based games or complex data structures.
// Create a two-dimensional array
var gridArray = array_create(10, undefined);
for (var i = 0; i < 10; ++i) {
gridArray[i] = array_create(10, undefined);
for (var j = 0; j < 10; ++j) {
gridArray[i][j] = instance_create_layer(i * 32, j * 32, "Instances", obj_Tile);
}
}
- Dynamic Arrays: GameMaker's arrays are dynamic, meaning they can grow and shrink during runtime. You can add items using the
array_push
function or remove them witharray_delete
.
// Push a new object onto the end of the array
array_push(objectArray, instance_create_layer(x, y, "Instances", obj_Collectible));
// Delete the second object in the array
array_delete(objectArray, 1);
Remember to manage your object instances properly to avoid memory leaks or errors. This means you should free up resources when they are no longer needed, such as using instance_destroy()
to remove an object instance from the game when it's no longer in use.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- Does Unreal Engine work on Linux?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
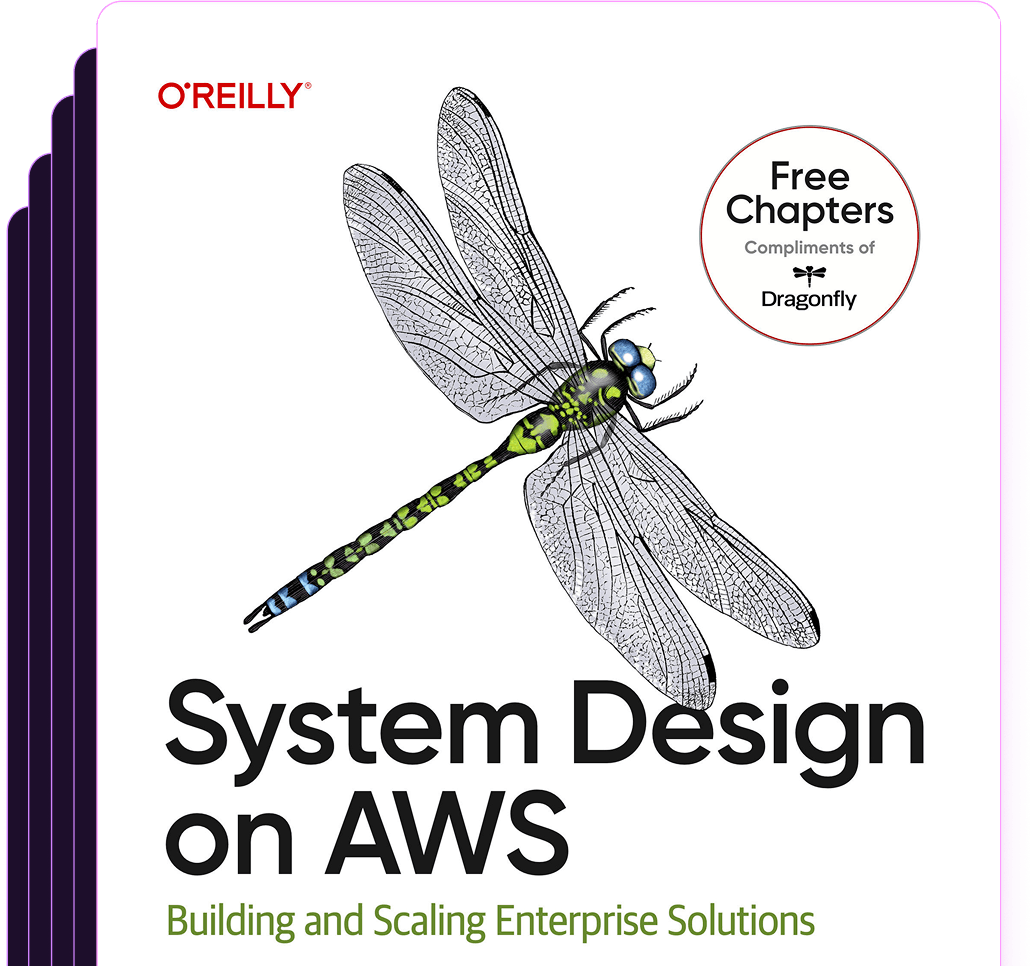
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost