Question: Is Couchbase an in-memory database?
Answer
Yes and No. Couchbase Server, often simply referred to as Couchbase, is a NoSQL database that does use in-memory storage for active data to provide fast read and write operations, but it's not purely an in-memory database like Redis. This means that while data is held in memory for performance reasons, it is also persisted on disk for durability, making Couchbase a distributed, multi-model NoSQL document-oriented database.
In fact, Couchbase utilizes both in-memory and on-disk technology. It uses a managed cache to hold the most frequently used or recently used data in RAM. It also maintains a full copy of all the data on disk to ensure that even in the event of power failure, no data is lost. This combination of in-memory speed with on-disk data persistence is what differentiates Couchbase from pure in-memory databases.
Here's a simple example of how you might interact with Couchbase in Java:
// Connect to Couchbase
Cluster cluster = CouchbaseCluster.create("127.0.0.1");
Bucket bucket = cluster.openBucket("default");
// Create a JSON Document
JsonObject arthur = JsonObject.create()
.put("name", "Arthur")
.put("email", "kingarthur@couchbase.com")
.put("interests", JsonArray.from("Holy Grail", "African Swallows"));
// Store the Document
bucket.upsert(JsonDocument.create("u:king_arthur", arthur));
// Load the Document and print it
// Prints Content and Metadata of the stored Document
System.out.println(bucket.get("u:king_arthur"));
// Close all buckets and disconnect
cluster.disconnect();
Please note that depending on the specific requirements of your applications, especially in terms of durability vs performance, Couchbase might be more suitable than purely in-memory databases, or vice versa.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common In Memory Questions (and Answers)
- What is a Distributed Cache and How Can It Be Implemented?
- How do you design a distributed cache system?
- What is a persistent object cache and how can one implement it?
- How can I set up and use Redis as a distributed cache?
- Why should you use a persistent object cache?
- What are the differences between an in-memory cache and a distributed cache?
- What is AWS's In-Memory Data Store Service and how can it be used effectively?
- What is a distributed cache in AWS and how can it be implemented?
- How can you implement Azure distributed cache in your application?
- What is the best distributed cache system?
- Is Redis a distributed cache?
- What is the difference between a replicated cache and a distributed cache?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
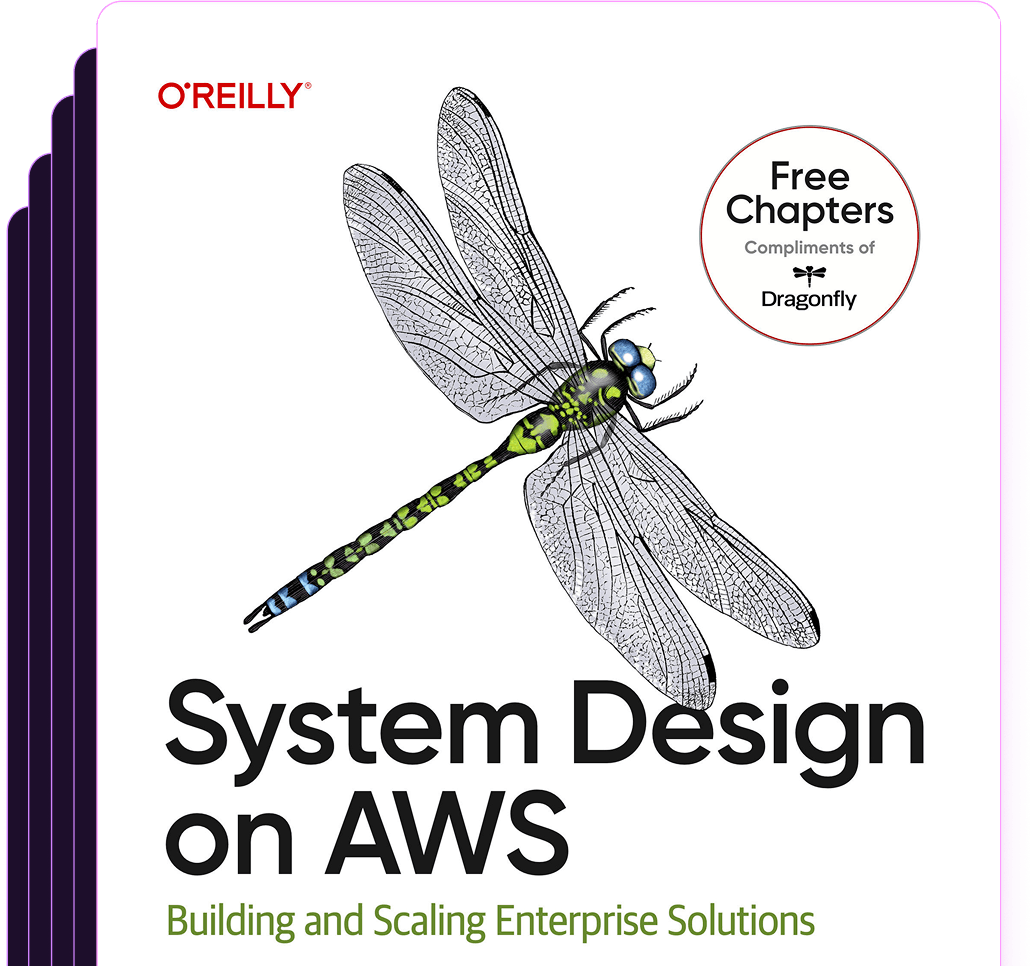
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost