Question: Is Hazelcast a distributed cache?
Answer
Yes, Hazelcast is indeed a distributed cache.
Hazelcast IMDG (In-Memory Data Grid) is an open-source in-memory data grid based on Java. It provides distributed implementations for core data structures such as java.util.{Map, Queue, Set, List}
, and additional data structures like MultiMap
, Lock
, Topic
etc.
Here are key features that make Hazelcast a potent distributed cache:
- Distributed and Replicated In-Memory Store: Hazelcast stores data in memory across all nodes in the cluster. This makes it lightning fast as there's no disk I/O overhead. Additionally, to handle failures, it replicates data across multiple nodes.
- Elastic Scalability: Hazelcast can scale out to hundreds of nodes, and scales back in when nodes are removed. It can do this while your application is running, with no downtime.
- Data Sharding: Data in Hazelcast is automatically partitioned across nodes, ensuring balanced data and workload distribution.
- Transparent Networking: Hazelcast has automatic discovery and handling of network partitions. The latter is especially important in maintaining data consistency and availability during network disruptions.
- Thread-Safe Operations: Hazelcast is entirely thread-safe. It uses locks to maintain data integrity during concurrent access.
- Transactional Capability: It supports JTA (Java Transaction API) and XA transactions helping you control data manipulation and ensure data integrity.
- Persistence: You can persist the cached data to a database, making it suitable for caching database queries.
Here's an example of how to create a Hazelcast instance and use it as a distributed cache:
import com.hazelcast.core.Hazelcast;
import java.util.Map;
HazelcastInstance hz = Hazelcast.newHazelcastInstance();
Map<Integer, String> map = hz.getMap("my-distributed-map");
map.put(1, "Hello Hazelcast");
System.out.println("Value for key 1 is " + map.get(1));
hz.shutdown();
This example creates a Hazelcast instance and adds an entry into a distributed map. This map is not local; it's distributed across all Hazelcast nodes.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common In Memory Questions (and Answers)
- What is a Distributed Cache and How Can It Be Implemented?
- How do you design a distributed cache system?
- What is a persistent object cache and how can one implement it?
- How can I set up and use Redis as a distributed cache?
- Why should you use a persistent object cache?
- What are the differences between an in-memory cache and a distributed cache?
- What is AWS's In-Memory Data Store Service and how can it be used effectively?
- What is a distributed cache in AWS and how can it be implemented?
- How can you implement Azure distributed cache in your application?
- What is the best distributed cache system?
- Is Redis a distributed cache?
- What is the difference between a replicated cache and a distributed cache?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
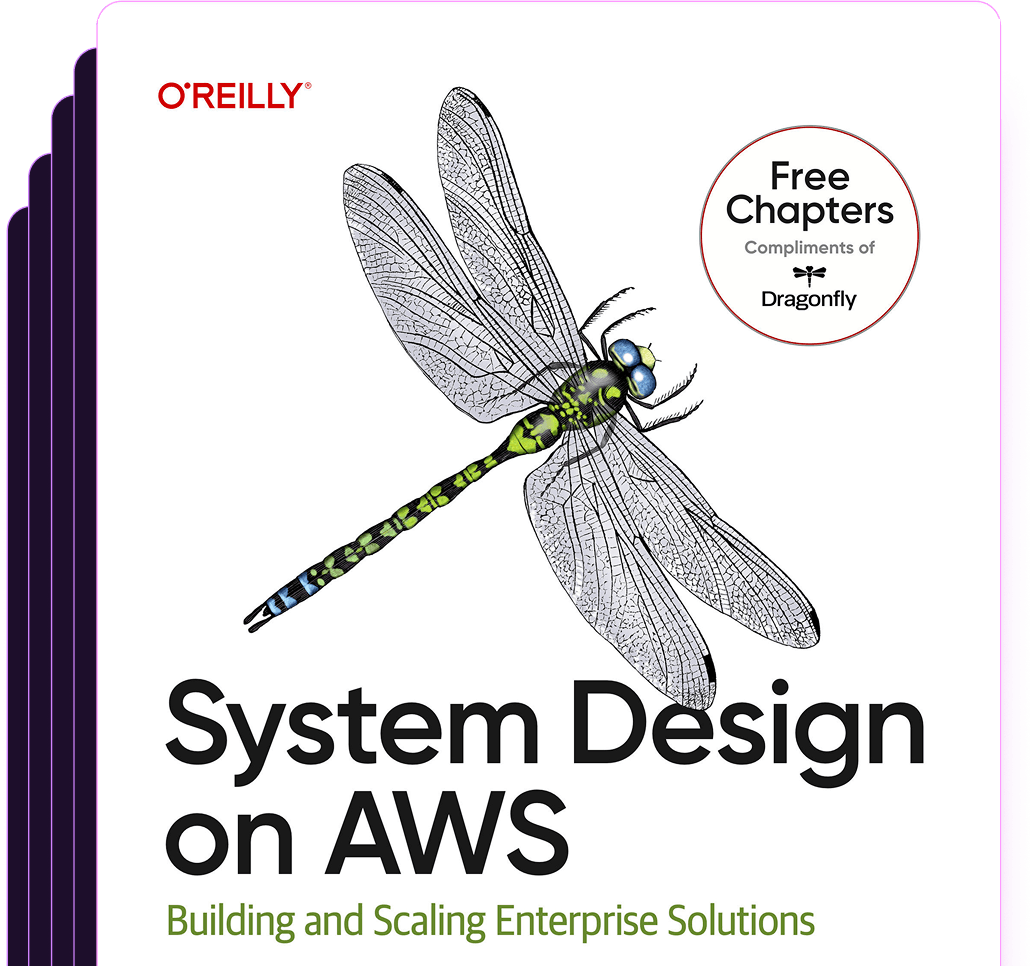
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost