Question: How can you use a key-value store for images?
Answer
Key-value stores are a type of NoSQL database designed for storing, retrieving, and managing associative arrays, or dictionaries, where each key is unique and associated with one particular value. Storing images in a key-value store can be quite effective for certain use cases, such as caching thumbnails or user profile pictures. Here's how to do it effectively:
Why Use Key-Value Stores for Images?
- Performance: Key-value stores are optimized for speed, making them a great option for applications that require fast access to data like images.
- Scalability: They can scale out easily, allowing you to store millions of images without significant performance degradation.
- Simplicity: The simple structure of key-value stores makes them easy to use and manage.
How to Store Images
Storing images in a key-value store involves converting the image into a format that can be stored as a value. Generally, this means converting the image into a binary format or base64 encoded string.
Example using Redis:
Redis is a popular in-memory key-value store that supports storing binary data, which makes it suitable for image storage.
import redis
from PIL import Image
import io
# Connect to Redis
r = redis.Redis(host='localhost', port=6379, db=0)
# Load your image with Pillow
image_path = 'path/to/your/image.jpg'
image = Image.open(image_path)
# Convert the image to bytes
img_byte_arr = io.BytesIO()
image.save(img_byte_arr, format=image.format)
img_byte_arr = img_byte_arr.getvalue()
# Use the image name or any unique identifier as the key
key = 'unique_image_key'
# Store the image
r.set(key, img_byte_arr)
# Retrieve the image
img_bytes = r.get(key)
# Convert back to an image
img = Image.open(io.BytesIO(img_bytes))
img.show()
Considerations
- Storage Limits: Some key-value stores might have limits on the value size (e.g., Redis has a 512 MB limit for a single value).
- Cost: While key-value stores are efficient, storing a large number of high-resolution images may require substantial memory or disk space, potentially increasing costs.
- Access Patterns: Key-value stores are best suited for scenarios where you access images via a known key. If you need complex querying capabilities, consider pairing with another database or search index.
Conclusion
Using key-value stores for images can be a powerful solution for specific scenarios requiring fast read access and simple storage needs. However, it's important to consider the limitations and costs associated with storing large amounts of binary data in these databases.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Key-Value Databases Questions (and Answers)
- What are the disadvantages of key-value databases?
- What are the advantages of a key-value database?
- Is MongoDB a key-value database?
- How fast are key-value databases?
- What are the differences between key-value stores and relational databases?
- What is the difference between key-value and document databases?
- What are the characteristics and features of key-value store databases?
- What are the differences between key-value databases and Cassandra?
- When should a key-value database not be used?
- How do you design a database using key-value tables?
- Are key-value databases similar to tables in RDBMS?
- Is Redis a key-value store?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
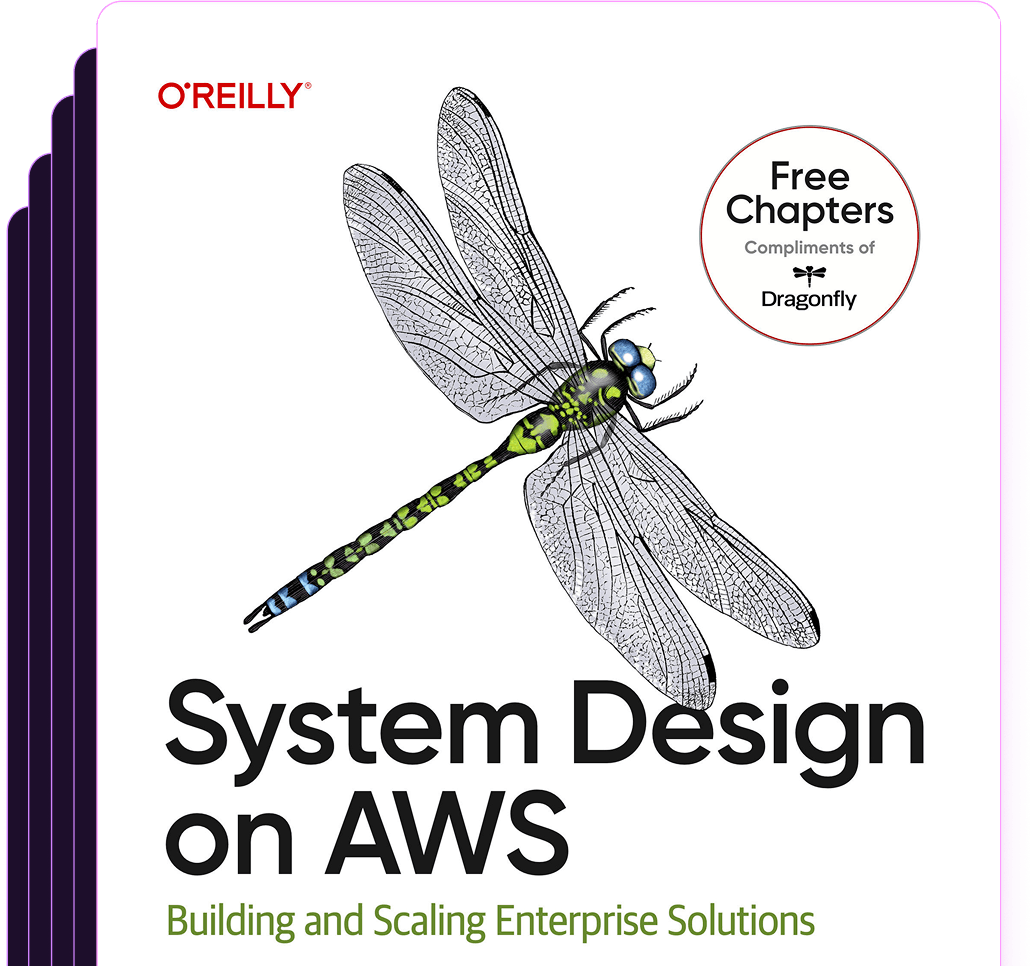
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost