Question: How does a message queue facilitate one-to-many communication?
Answer
In messaging systems, a message queue is a fundamental component that helps in managing messages. When it comes to the one-to-many message pattern, the goal is for a message from a single producer to be consumed by multiple consumers. Understanding how this functionality works is crucial for designing robust systems that require message broadcasting.
How Message Queues Support One-to-Many Communication
- Overview of Message Queues:
A message queue is a form of asynchronous communication protocol that allows for decoupling of message producers and consumers. The producer sends messages to the queue, and consumers receive them on demand. - One-to-Many Distribution:
In one-to-many communication, a single input (message) from a producer should reach multiple consumers. The typical way to accomplish this in a message queue is through either a fan-out or publishing/subscribing mechanism.
- Fan-Out Pattern:
- Implementation: The 'fan-out' mechanism involves broadcasting the message to all attached consumers. This pattern is often implemented using a publish-subscribe model where the producer sends messages to multiple queues or via topics.
- Use Case: It's commonly used in scenarios like notification systems, live updates, or other broadcast-style interactions.
- Publish-Subscribe Model:
- Mechanism: In a publish-subscribe (pub/sub) model, producers publish messages to topics instead of queues. Consumers subscribe to these topics to receive messages.
- Benefits: This model ensures that all subscribed consumers receive the message, thus supporting the one-to-many distribution.
- Example with RabbitMQ:
An example to illustrate this can be seen using RabbitMQ, a popular message broker. The publish-subscribe pattern can be implemented using the exchange
object with a type of fanout
. Here's a simple illustration in Python using the Pika library:
```python
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
# Declare a fanout exchange
channel.exchange_declare(exchange='logs', exchange_type='fanout')
# Publish a message to the exchange
message = "Hello, all subscribers!"
channel.basic_publish(exchange='logs', routing_key='', body=message)
print(" [x] Sent %r" % message)
connection.close()
```
In this example, any queue that is bound to the logs
exchange would receive the message, enabling one-to-many distribution.
- Other Mechanisms:
- Multicast and Broadcast: In some contexts, one-to-many can be referred to as multicast or broadcast, where messages are distributed to multiple destinations.
- Cloud Solutions: Native cloud services such as AWS SNS (Simple Notification Service) or Google Cloud Pub/Sub offer built-in support for these patterns.
Implementing a one-to-many communication strategy using message queues allows for expanding the messaging system's reach and efficiency, ensuring that multiple consumers can process the same piece of information simultaneously. By using pub/sub or fan-out designs, systems can become more scalable and resilient to demand without tightly coupling producers with consumers.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Messaging Systems Questions (and Answers)
- What are the benefits of a message broker?
- When to use a message broker?
- What are the benefits of using a message queue?
- What are the use cases for message queues?
- What are the use cases for a message broker?
- When to use a message queue?
- What are the best practices for using message queues?
- What is the fastest message broker?
- Is message queue bidirectional?
- Can I delete a message queue?
- What are the types of message brokers?
- Message Broker vs ESB - What's The Difference?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
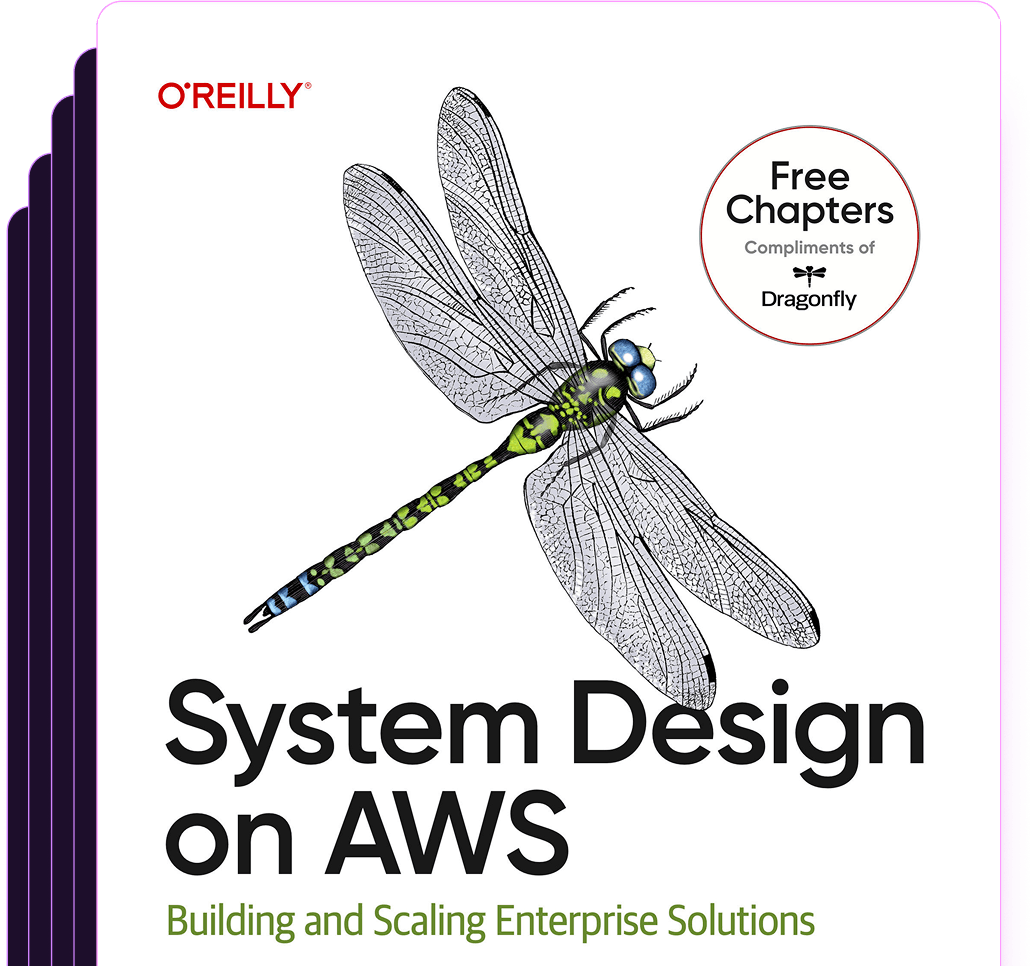
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost