Question: Why is my MongoDB loading slow?
Answer
There can be several reasons why MongoDB is loading slow. Below are some common causes and solutions to improve performance:
1. Unoptimized Queries
Poorly designed queries can significantly impact the performance of your MongoDB database. Ensure indexes are properly used to speed up query execution.
Solution:
- Use the
explain()
method on your queries to analyze their execution plan. - Create indexes that match your query patterns.
db.collection.createIndex({ fieldName: 1 }) // Creates an ascending index on 'fieldName'
2. Insufficient Indexes
Lack of appropriate indexes means MongoDB has to scan more documents than necessary, leading to slower response times.
Solution:
- Identify frequently accessed fields and create indexes on those fields.
- Regularly review and optimize your indexing strategy.
3. Large Documents
Retrieving large documents can slow down your queries, especially if you only need a part of the document.
Solution:
- Use projection to limit the fields returned by your query.
db.collection.find({}, { field1: 1, field2: 1 }) // Only returns field1 and field2
4. Hardware Limitations
MongoDB's performance can be constrained by hardware, such as CPU, RAM, or disk speed.
Solution:
- Monitor your system's resources and upgrade if necessary.
- Consider using faster SSDs for storage.
- Ensure there's enough RAM to hold your working set.
5. Network Issues
Slow network connections between your application and MongoDB server can lead to latency.
Solution:
- Optimize your network infrastructure.
- Keep your MongoDB servers geographically close to your users.
- Consider using MongoDB Atlas for cloud-based solutions with global clusters.
6. Database Size
Very large databases can experience performance issues due to the sheer volume of data.
Solution:
- Implement sharding to distribute data across multiple servers.
- Regularly archive old or infrequently accessed data.
7. Concurrency
High levels of concurrent accesses can overload the server.
Solution:
- Use connection pooling in your application to manage simultaneous connections efficiently.
- Evaluate MongoDB's WiredTiger storage engine concurrency settings.
By addressing these common issues, you can significantly improve the loading speed of your MongoDB database. Monitoring tools like MongoDB Atlas or third-party applications can provide insights into database performance and help identify bottlenecks.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common MongoDB Performance Questions (and Answers)
- How to improve MongoDB query performance?
- How to check MongoDB replication status?
- How do you connect to a MongoDB cluster?
- How do you clear the cache in MongoDB?
- How many connections can MongoDB handle?
- How does MongoDB sharding work?
- How to check MongoDB cluster status?
- How to change a MongoDB cluster password?
- How to create a MongoDB cluster?
- How to restart a MongoDB cluster?
- How do I reset my MongoDB cluster password?
- How does the $in operator affect performance in MongoDB?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
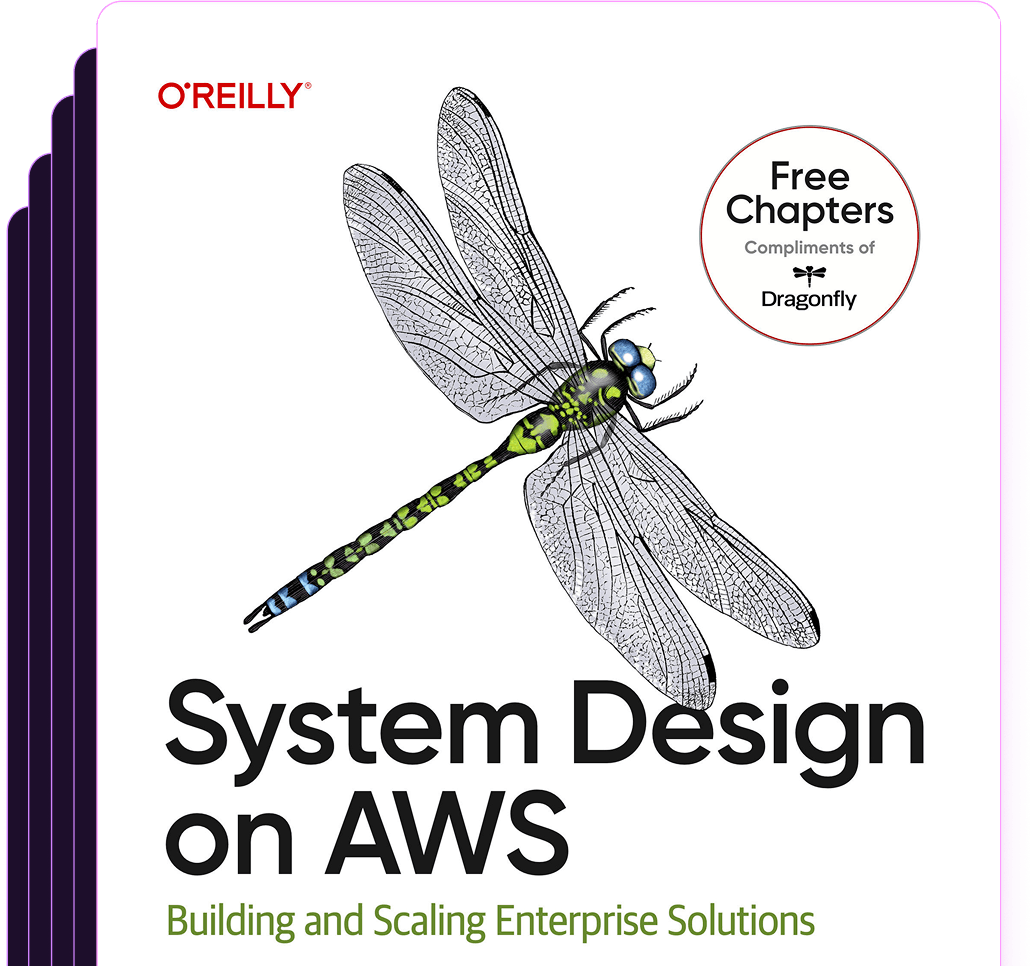
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost