Redis HDEL in Java (Detailed Guide w/ Code Examples)
Use Case(s)
One of the most common use cases for HDEL
in Redis is when you want to remove one or more fields from a hash stored at a certain key. This can be useful, for example, when you are using Redis as a cache and need to invalidate certain parts of it without affecting others.
Code Examples
Java has several libraries that provide connectivity to a Redis instance including Jedis and Lettuce. Here's an example of how to use HDEL with Jedis:
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args) {
// Connecting to Redis server on localhost
Jedis jedis = new Jedis("localhost");
// Create a hash
jedis.hset("hashKey", "field1", "value1");
jedis.hset("hashKey", "field2", "value2");
// Delete field1 from the hash
jedis.hdel("hashKey", "field1");
// Print Remaining fields
System.out.println(jedis.hgetAll("hashKey"));
// Close connection
jedis.close();
}
}
In this example, first we connect to the Redis server running on localhost. Then we create a hash with two fields: 'field1' and 'field2'. The hdel
method is then used to delete 'field1' from the hash. Finally, we print out the remaining fields in the hash and close the connection.
Best Practices
- Always close your Jedis instances once you've finished using them - this is crucial to prevent resource leaks.
- Use a try-with-resources statement if you're using Java 7 or above to automatically close the instance and handle exceptions.
Common Mistakes
- Do not use HDEL on non-hash keys. Using HDEL against a key holding a data type other than hash results in an error.
- Not handling exceptions - network or Redis server issues can cause operations to fail, so always have error handling in place.
FAQs
1. What happens if I use HDEL on a field that doesn't exist? It will simply return 0 and not throw an error.
2. What happens if I use HDEL on a key that doesn't exist? The command will be ignored silently.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
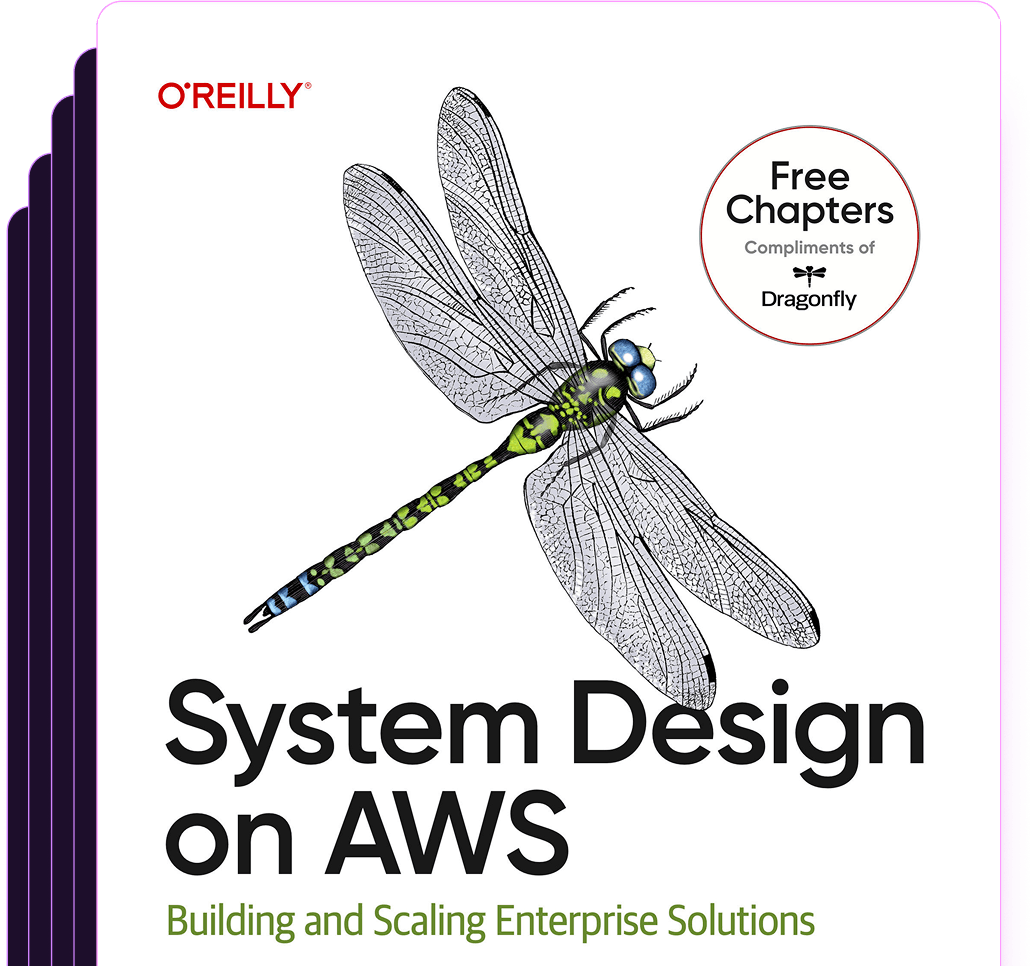
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost