Redis HGETALL in Java (Detailed Guide w/ Code Examples)
Use Case(s)
HGETALL
is a command used in Redis to retrieve all fields and their values of a hash stored at a key. In Java, it's often used when:
- Fetching the complete details of an object that is stored as Hash in Redis.
- Analyzing or processing all elements of a cached dataset.
Code Examples
Here we're using Jedis, a popular Java client for interfacing with a Redis database.
Example 1: Basic usage of hgetAll
import redis.clients.jedis.Jedis;
Jedis jedis = new Jedis("localhost");
String key = "user:1";
Map<String, String> result = jedis.hgetAll(key);
In this example, the hgetAll
method is used to retrieve all fields and their corresponding values from the hash at the key "user:1".
Example 2: Iterating over the result
import redis.clients.jedis.Jedis;
Jedis jedis = new Jedis("localhost");
String key = "user:1";
Map<String, String> result = jedis.hgetAll(key);
for (Map.Entry<String, String> entry : result.entrySet()) {
System.out.println("Field: " + entry.getKey() + ", Value: " + entry.getValue());
}
In the second example, we iterate over each key-value pair of the returned map and print them out.
Best Practices
- Avoid large hashes: While Redis can handle large hashes, retrieving huge datasets into your application might cause performance issues.
- Use connection pooling: Reusing connections via a pool (like JedisPool) is more efficient than creating a new one for every operation.
- Error handling: Always include error handling while dealing with Redis operations.
Common Mistakes
- Ignoring return type:
HGETALL
returns a map of strings (field-value pairs). Ignoring or mishandling this can lead to runtime errors. - Not checking if the key exists: Before using
HGETALL
, make sure that the key exists and is of hash type to avoid unexpected results.
FAQs
Q: What happens if the key does not exist in Redis? A: The hgetAll
method will return an empty Map.
Q: Can I use HGETALL
on keys holding data types other than hashes? A: No, HGETALL
is specifically designed for hash data types. Using it on others will result in an error.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
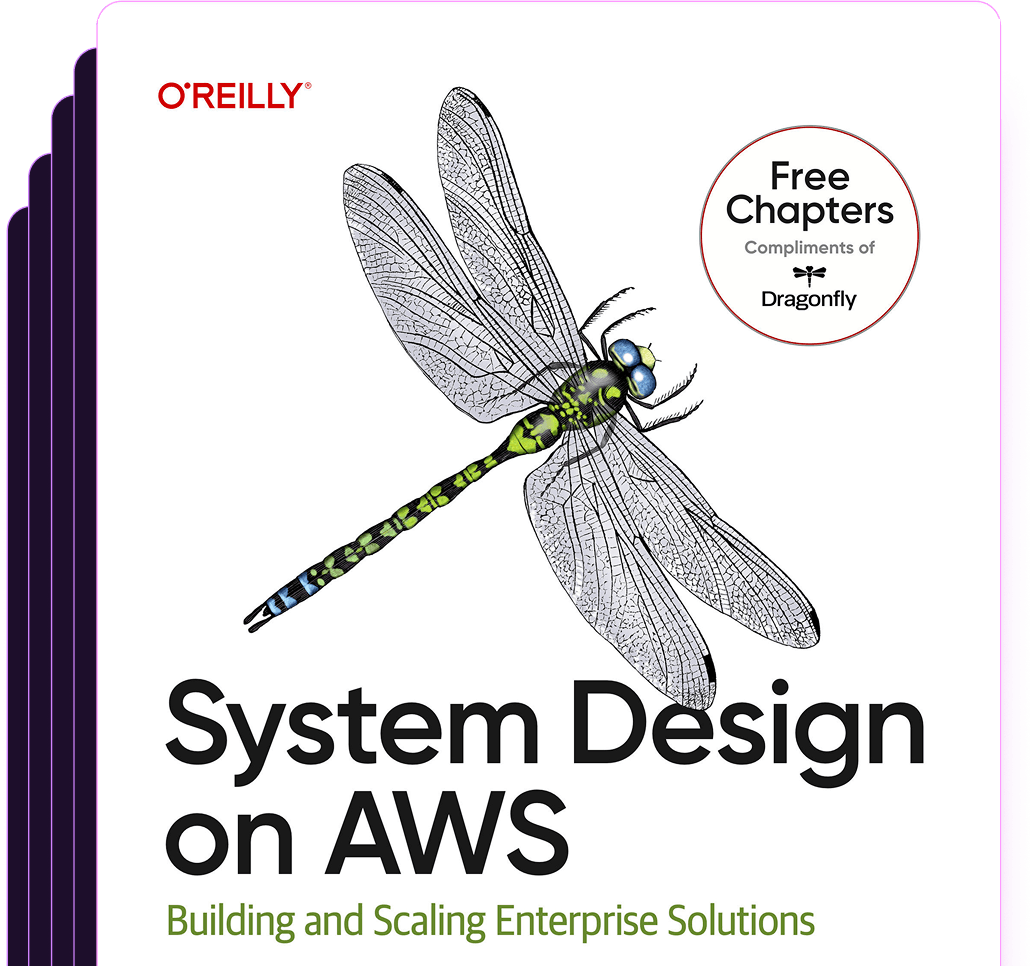
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost