Redis Bulk Update in Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
- Efficiently updating multiple keys in a single operation to reduce network latency.
- Performing batch updates on cache data.
- Synchronizing bulk data changes from a database to Redis.
Code Examples
Example 1: Using MSET
for Bulk Updates
The MSET
command is used to set multiple keys to multiple values in a single atomic operation.
const redis = require('redis');
const client = redis.createClient();
client.on('error', (err) => {
console.error('Error connecting to Redis:', err);
});
client.connect().then(async () => {
try {
await client.mSet({
'key1': 'value1',
'key2': 'value2',
'key3': 'value3'
});
console.log('Bulk update successful');
} catch (err) {
console.error('Bulk update failed:', err);
} finally {
client.quit();
}
});
Explanation: This example demonstrates how to use the MSET
command to update multiple keys at once. The mSet
method accepts an object where each property corresponds to a key-value pair.
Example 2: Using Pipelining for Bulk Updates
Pipelining allows you to send multiple commands to Redis without waiting for the replies, which can be more efficient than sending them one by one.
const redis = require('redis');
const client = redis.createClient();
client.on('error', (err) => {
console.error('Error connecting to Redis:', err);
});
client.connect().then(async () => {
const pipeline = client.pipeline();
pipeline.set('key1', 'bulk_value1');
pipeline.set('key2', 'bulk_value2');
pipeline.set('key3', 'bulk_value3');
try {
const results = await pipeline.exec();
console.log('Pipeline execution results:', results);
} catch (err) {
console.error('Pipeline execution failed:', err);
} finally {
client.quit();
}
});
Explanation: This example makes use of Redis pipelining to execute multiple SET
commands in a single request/response cycle, improving performance for bulk operations.
Best Practices
- Use pipelining or
MSET
to minimize round-trip time and improve performance when performing bulk updates. - Always handle errors appropriately to ensure that your application can recover from failures during bulk operations.
Common Mistakes
- Forgetting to call
pipeline.exec()
after adding commands to the pipeline, which results in the commands not being executed. - Not properly handling connection errors, which can lead to unhandled promise rejections or application crashes.
FAQs
Q: What is the difference between using MSET
and pipelining for bulk updates?
A: MSET
performs a single atomic operation to update multiple keys, while pipelining allows you to queue multiple commands and execute them together. Pipelining can be more flexible as it supports different types of commands, not just SET
.
Q: Can I use transactions for bulk updates in Redis?
A: Yes, you can use Redis transactions with MULTI
and EXEC
commands to ensure that a series of commands are executed atomically, but this typically has more overhead compared to pipelining or MSET
.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
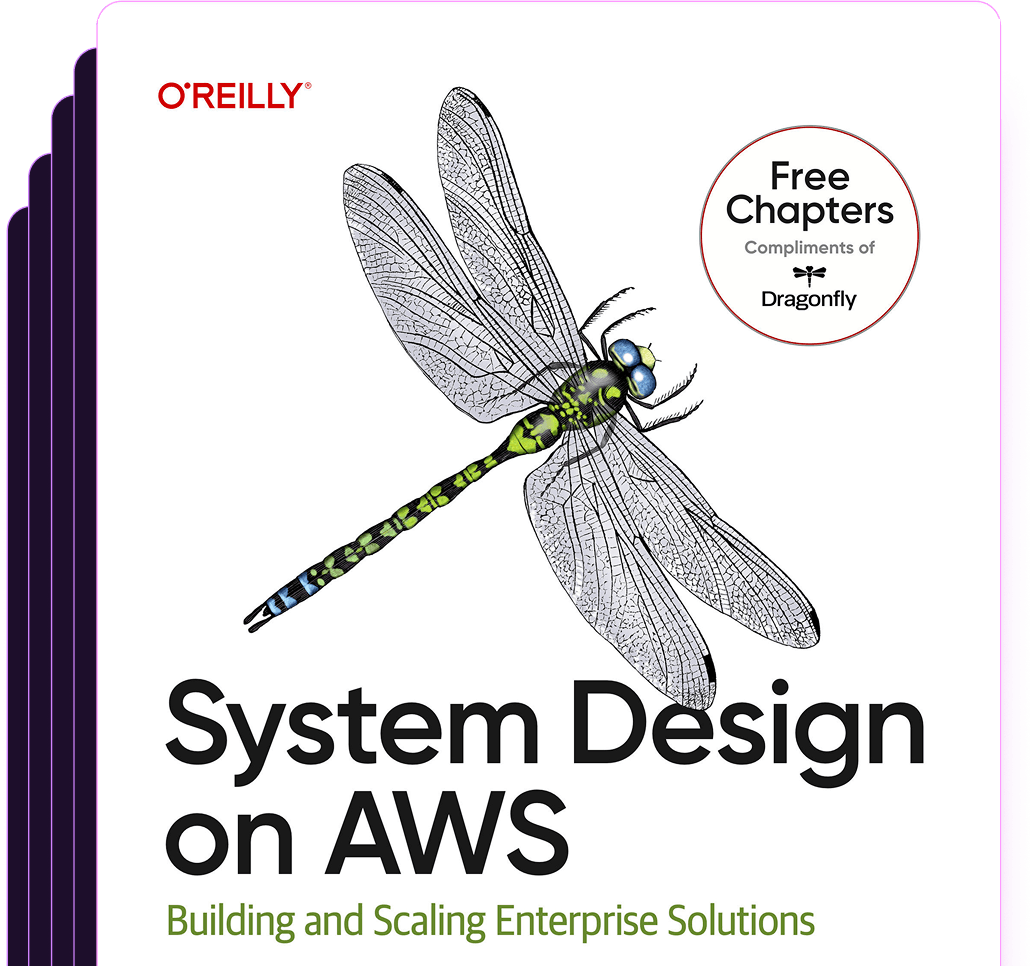
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost