Redis JSON Update in Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
- Updating specific fields in a JSON object stored in Redis.
- Efficiently managing complex data structures without retrieving and rewriting entire objects.
- Real-time updates to user profiles, session data, or configuration settings.
Code Examples
Example 1: Updating a Single Field in a JSON Object
This example demonstrates how to update a single field in a JSON object stored in Redis using the node-redis
package along with the redis-json
module.
const redis = require('redis');
const { promisify } = require('util');
const client = redis.createClient();
const setAsync = promisify(client.set).bind(client);
const getAsync = promisify(client.get).bind(client);
// Assuming you have previously set a JSON object
async function updateJsonField(key, field, value) {
const jsonString = await getAsync(key);
let jsonObject = JSON.parse(jsonString);
jsonObject[field] = value;
await setAsync(key, JSON.stringify(jsonObject));
console.log(`Updated ${field} to ${value} in ${key}`);
}
// Usage
updateJsonField('user:1000', 'age', 30);
Explanation:
- Connect to Redis using the
redis
package. - Define a function
updateJsonField
to update a field within a JSON object. - Retrieve and parse the existing JSON object.
- Update the specified field and save the updated JSON back to Redis.
Example 2: Using RedisJSON Module for Efficient Updates
RedisJSON is a Redis module that allows natively handling, storing, and updating JSON documents. This example uses the ioredis
package with RedisJSON.
const Redis = require('ioredis');
const redis = new Redis();
// Assuming you have previously set a JSON object using RedisJSON
async function updateJsonFieldRedisJSON(key, path, value) {
await redis.call('JSON.SET', key, path, JSON.stringify(value));
console.log(`Updated ${path} to ${JSON.stringify(value)} in ${key}`);
}
// Usage
updateJsonFieldRedisJSON('user:1000', '.age', 30);
Explanation:
- Connect to Redis using the
ioredis
package. - Define a function
updateJsonFieldRedisJSON
to update a field within a JSON object using the RedisJSON module. - Use the
JSON.SET
command to update the specific path in the JSON document.
Best Practices
- Use Modules: Leverage Redis modules like RedisJSON for more efficient and native handling of JSON documents.
- Minimal Updates: Update only necessary fields to minimize network overhead and improve performance.
- Error Handling: Always include error handling to deal with potential issues when interacting with Redis.
Common Mistakes
- Not Parsing JSON: Forgetting to parse JSON strings retrieved from Redis can lead to errors when trying to update fields.
- Overwriting Entire Objects: Avoid fetching and rewriting entire JSON objects when only a small update is needed; this is inefficient and prone to race conditions.
- Incorrect Path Syntax: When using RedisJSON, ensure the path syntax (e.g.,
.age
) is correct to avoidERR wrong parameters
errors.
FAQs
Q: Can I update nested fields within a JSON object in Redis?
A: Yes, using RedisJSON, you can update nested fields by specifying the path (e.g., user:1000
, .address.city
).
Q: Is the RedisJSON module required for handling JSON in Redis?
A: No, it's not required but highly recommended for efficiency and native JSON support. Without it, you'll need to handle JSON serialization and deserialization manually.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
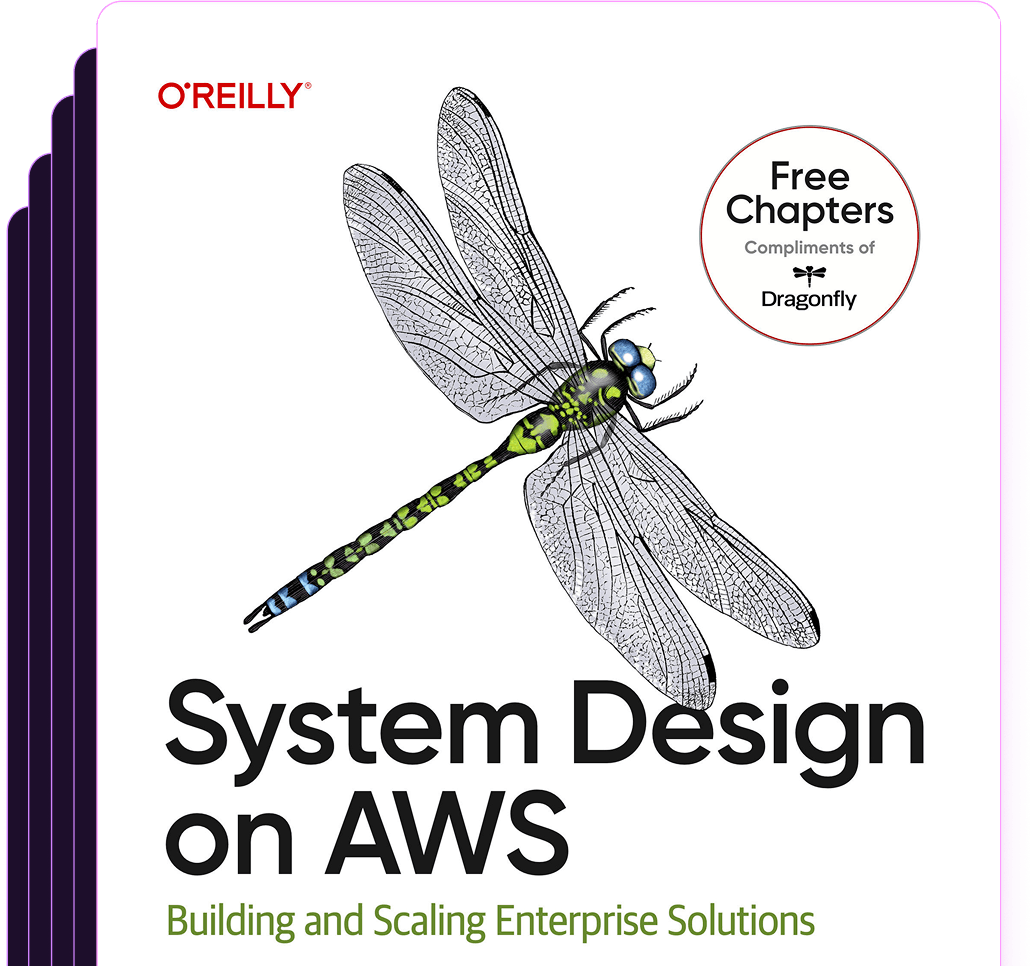
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost