Node Redis: Get All Keys (Detailed Guide w/ Code Examples)
Use Case(s)
In Node.js applications, you may need to fetch all keys from a Redis database. This is often used for debugging purposes or when you need to analyze or manipulate all data.
## Code Examples
- Using
KEYS *
command:
```
const redis = require('redis');
const client = redis.createClient();
client.keys('*', (err, keys) => {
if (err) return console.log(err);
for(let i = 0; i < keys.length; i++) {
console.log(keys[i]);
}
});
```
In this example, KEYS *
command is used to fetch all the keys from Redis. The returned keys are then logged to the console.
- Using
SCAN
command for large databases:
```
const redis = require('redis');
const client = redis.createClient();
let cursor = '0';
function scan() {
client.scan(cursor, 'MATCH', '*', 'COUNT', '100', function(err, reply) {
if(err) throw err;
cursor = reply[0];
reply[1].forEach(function(key, i) {
console.log(key);
});
if(cursor === '0') {
return console.log('Scan complete');
} else {
return scan();
}
});
}
scan();
```
For larger databases, it's better to use SCAN
as KEYS *
may end up blocking the server while it fetches all keys. In this example, SCAN
is used in a loop until all keys are fetched and logged.
Best Practices
- Avoid using
KEYS *
command in a production environment as it might affect performance. Instead, useSCAN
as it's more efficient and doesn't block the server.
Common Mistakes
- Using
KEYS *
on large databases can lead to performance issues. It's best to useSCAN
for such cases.
FAQs
- Q: Can I filter keys when fetching them from Redis?
A: Yes, bothKEYS
andSCAN
commands accept a pattern that can be used to filter keys.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Node Redis: Get Length of List
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get Hash Values at Key
- Node Redis: Get Current Memory Usage
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
- Getting Master Status in Node Redis
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
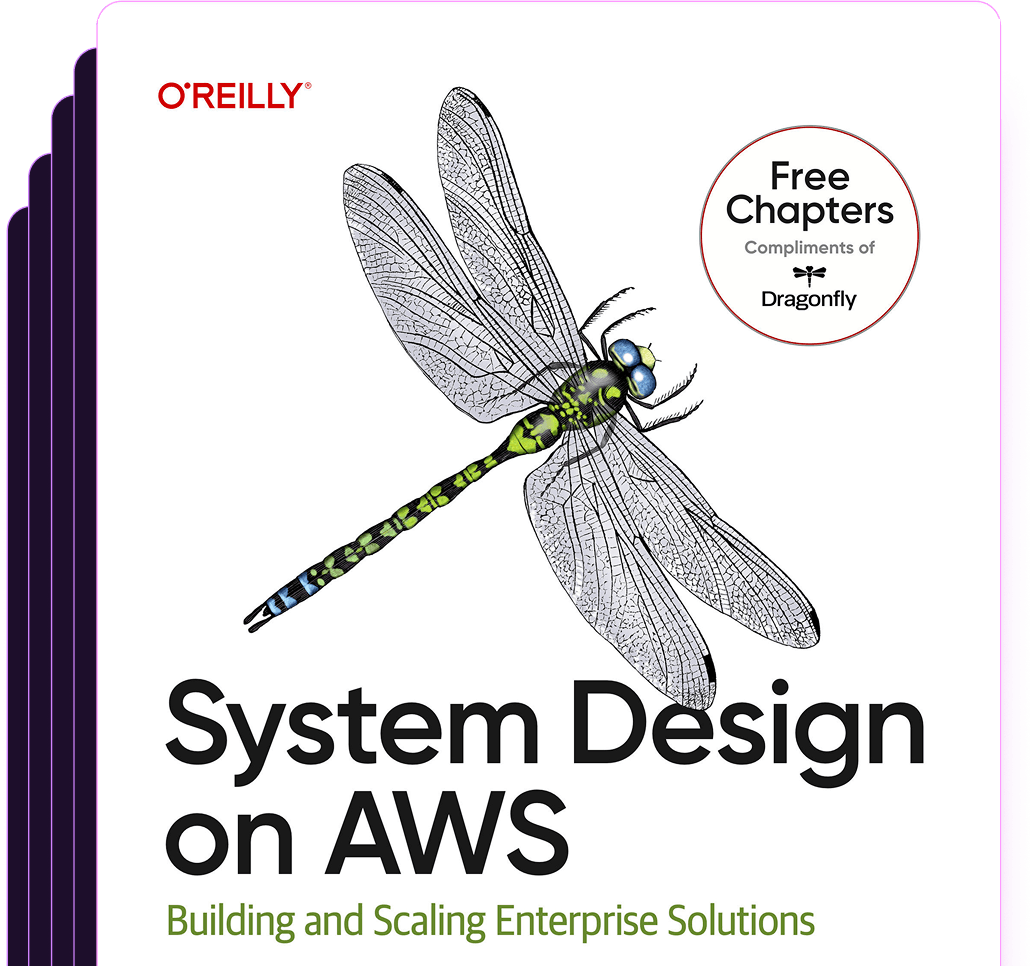
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost