Node Redis: Get Length of List (Detailed Guide w/ Code Examples)
Use Case(s)
Redis lists can be used to implement queues, stacks, and other data structures. A common use case is when you need to find the length of a list in Redis using Node.js. For example, you might have a queue of jobs and want to know how many are waiting.
Code Examples
In Node.js, you can use the lLen
function of the redis
package to get the length of a list.
Example:
const redis = require('redis');
const client = redis.createClient();
client.on('connect', function() {
console.log('Connected to Redis');
});
client.lLen('myList', function(err, length) {
if (err) throw err;
console.log('Length:', length);
});
In this example, we first connect to the Redis server using createClient
. After confirming the connection, lLen
is used to get the length of the list named 'myList'. The length is then logged to the console.
Best Practices
- Always handle errors in callbacks to prevent unhandled exceptions.
- Close the client connection after operations to free up resources.
Common Mistakes
- Attempting to get the length of a non-existent list will return 0 - it does not result in an error. Ensure the list exists before attempting operations.
- Blocking operations on an empty or non-existent list can block the client indefinitely.
FAQs
Q: What happens if the key exists but does not hold a list? A: Redis will return an error because lLen
is only for lists.
Q: Does getting the length of a list impact performance? A: No, getting the length of a list in Redis is an O(1) operation, meaning it has constant time complexity and does not depend on the size of the list.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
- Getting Master Status in Node Redis
- Node Redis: Get Number of Subscribers
- Getting the Last Update Time in Redis with Node.js
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
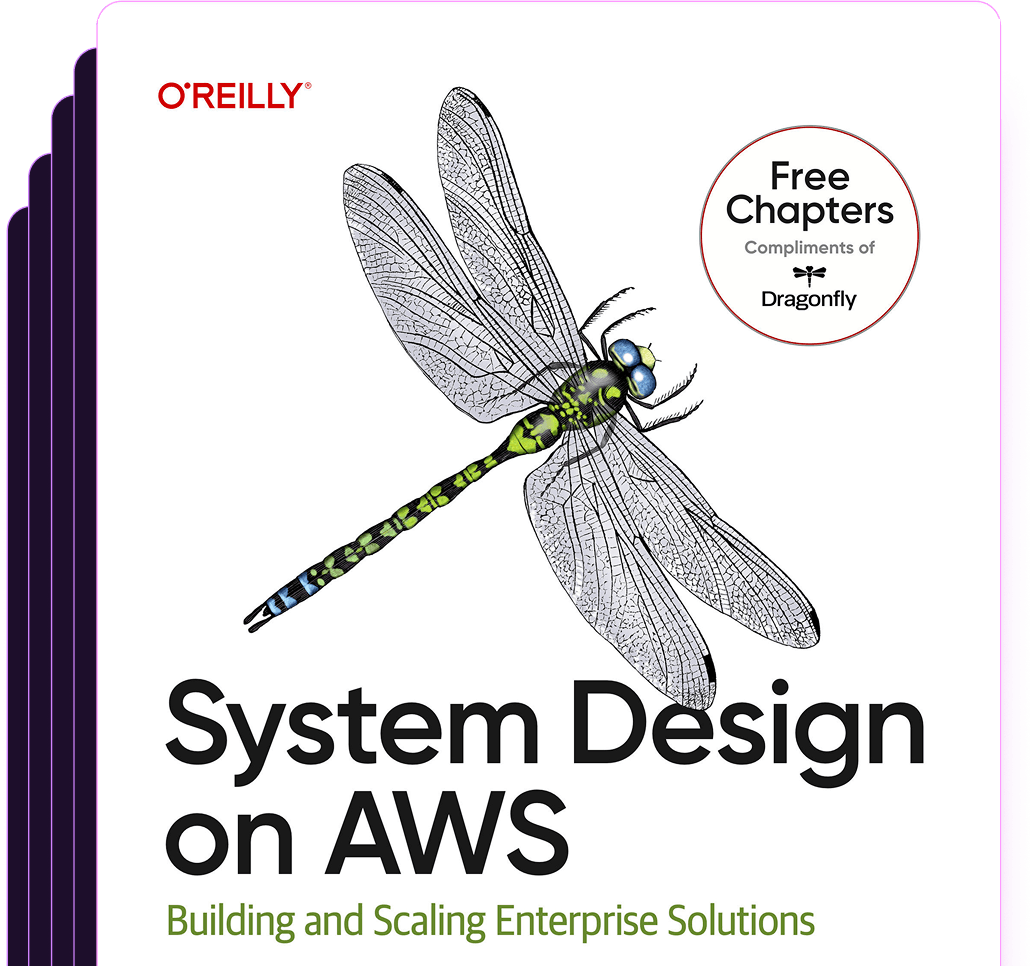
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost