Redis Update Value in List in Python (Detailed Guide w/ Code Examples)
Use Case(s)
- Updating elements in a list: Modify the value of an existing element in a Redis list to ensure data accuracy or reflect changes.
- Maintaining ordered collections: Keep an ordered list updated with the latest information, such as user actions, logs, or leaderboard scores.
Code Examples
Example 1: Updating an Element by Index
To update a value at a specific index in a Redis list, use the LSET
command. Here's how you do it in Python with the redis-py
library:
CODE_BLOCK_PLACEHOLDER_0
Explanation:
- Connect to Redis using
redis.StrictRedis
. - Populate the list
mylist
with initial values. - Use
lset
to update the value at index 1. - Retrieve and print the list to verify the update.
Example 2: Conditional Update
Sometimes you may need to update a value if certain conditions are met, like if the current value equals a specified value.
CODE_BLOCK_PLACEHOLDER_1
Explanation:
- Retrieve the current value at the specified index using
lindex
. - Conditionally update the value using
lset
.
Best Practices
- Index Validation: Ensure the index is within the bounds of the list to avoid errors.
- Atomic Operations: Wrap operations in transactions (
MULTI
/EXEC
) if updating multiple elements to maintain atomicity.
Common Mistakes
- Invalid Index: Using an out-of-bounds index will raise an error. Always check the list length before setting an index.
- Data Type Mismatch: Make sure the key you're operating on is indeed a list.
FAQs
Q: What happens if I try to update a non-existing index?
A: Redis will return an error if you attempt to update an index that does not exist. Validate the index within the list's range to prevent this.
Q: Can I update multiple values in a single command?
A: No, Redis does not support updating multiple values in a single LSET
command. You need to issue separate LSET
commands for each index.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Redis Concurrent Update in Python
- Redis Update Value Without Changing TTL in Python
- Redis Update Cache in Python
- Redis JSON Update in Python
- Redis Bulk Update in Python
- Redis Update TTL in Python
- Redis Update Value in Python
- Redis Update TTL on Read in Python
- Redis Atomic Update in Python
- Redis Conditional Update in Python
- Redis Partial Update in Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
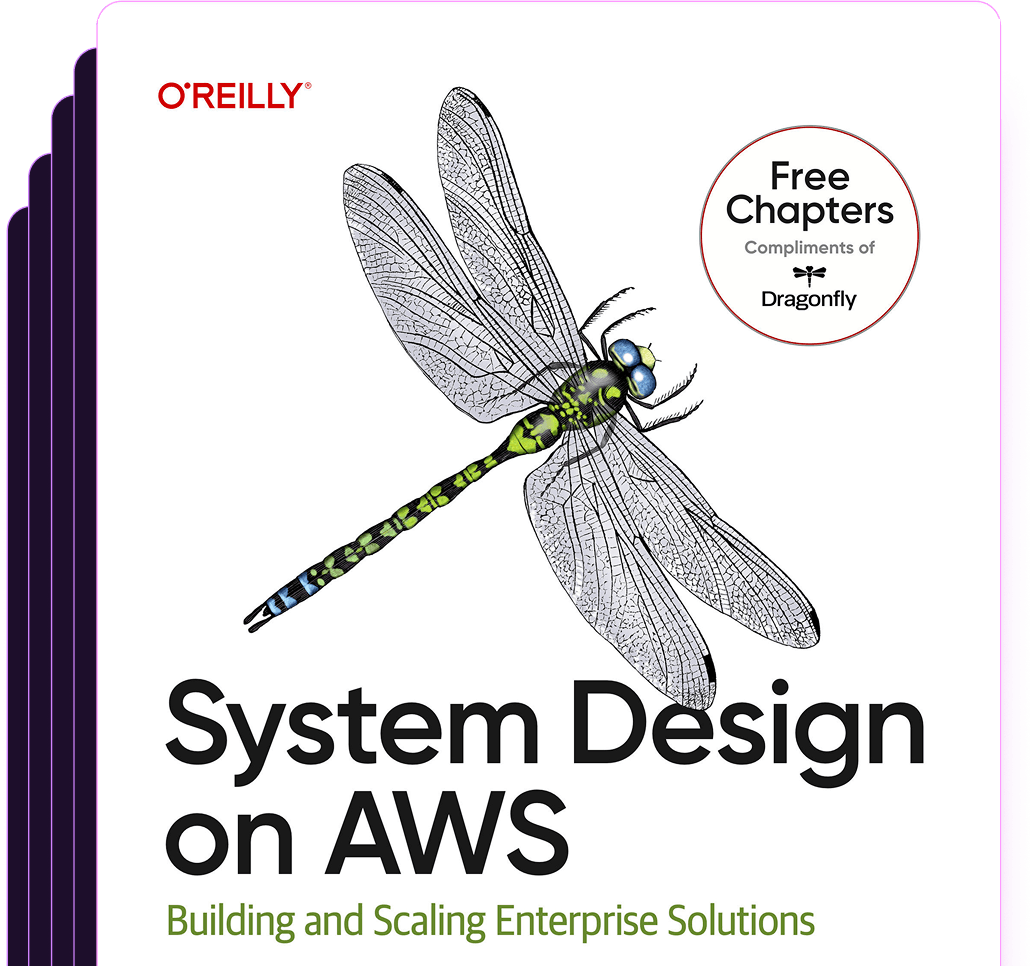
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost