Redis Update Value Without Changing TTL in Python (Detailed Guide w/ Code Examples)
Use Case(s)
Updating a value in Redis without altering its existing Time-To-Live (TTL) is often needed when the expiration policy is crucial. For example:
- Caching frequently updated data while maintaining the original expiration time.
- Updating session data where each user's session has a fixed expiration time.
Code Examples
Example 1: Using getset
to Update Value While Retaining TTL
import redis
# Connect to Redis
client = redis.StrictRedis(host='localhost', port=6379, db=0)
key = 'my_key'
new_value = 'new_value'
# Fetch current TTL
ttl = client.ttl(key) # Returns -2 if the key does not exist, or -1 if it exists but has no associated expire
if ttl > 0:
# Only update the value if the key exists and has an associated expire
client.set(key, new_value, ex=ttl)
else:
# If key does not have an expiration or does not exist, set the value without TTL
client.set(key, new_value)
Explanation:
- Connect to the Redis server.
- Fetch the current TTL of the key.
- Check if the TTL is positive.
- Update the value while retaining the original TTL using the
ex
parameter. - Handle cases where the key has no TTL or does not exist.
Example 2: Atomic Update with Lua Script
import redis
# Connect to Redis
client = redis.StrictRedis(host='localhost', port=6379, db=0)
key = 'my_key'
new_value = 'new_value'
# Lua script to update value and retain TTL
lua_script = """
local ttl = redis.call('ttl', KEYS[1])
if ttl > 0 then
redis.call('set', KEYS[1], ARGV[1])
redis.call('expire', KEYS[1], ttl)
else
redis.call('set', KEYS[1], ARGV[1])
end
"""
# Execute Lua script
client.eval(lua_script, 1, key, new_value)
Explanation:
- Connect to the Redis server.
- Define a Lua script that:
- Retrieves the current TTL of the key.
- Sets the new value.
- Re-applies the original TTL if it was positive.
- Execute the Lua script using
client.eval
.
Best Practices
- Always check for the existence of a key and its TTL before updating to avoid unintended behavior.
- Use Lua scripts for atomic operations to prevent race conditions, especially in a multi-client environment.
Common Mistakes
- Forgetting to handle keys that do not exist or have no TTL, leading to unexpected results.
- Overwriting the key without preserving its original TTL due to logic errors.
FAQs
Q: What happens if the key does not exist? A: The scripts and examples provided will handle this by either setting the key without TTL or doing nothing, depending on the specific implementation details.
Q: Can I use other methods to fetch TTL and update values? A: Yes, you can use pipeline transactions to fetch and update values, though Lua scripting is generally preferred for atomicity and simplicity.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Redis Concurrent Update in Python
- Redis Update Value in List in Python
- Redis Update Cache in Python
- Redis JSON Update in Python
- Redis Bulk Update in Python
- Redis Update TTL in Python
- Redis Update Value in Python
- Redis Update TTL on Read in Python
- Redis Atomic Update in Python
- Redis Conditional Update in Python
- Redis Partial Update in Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
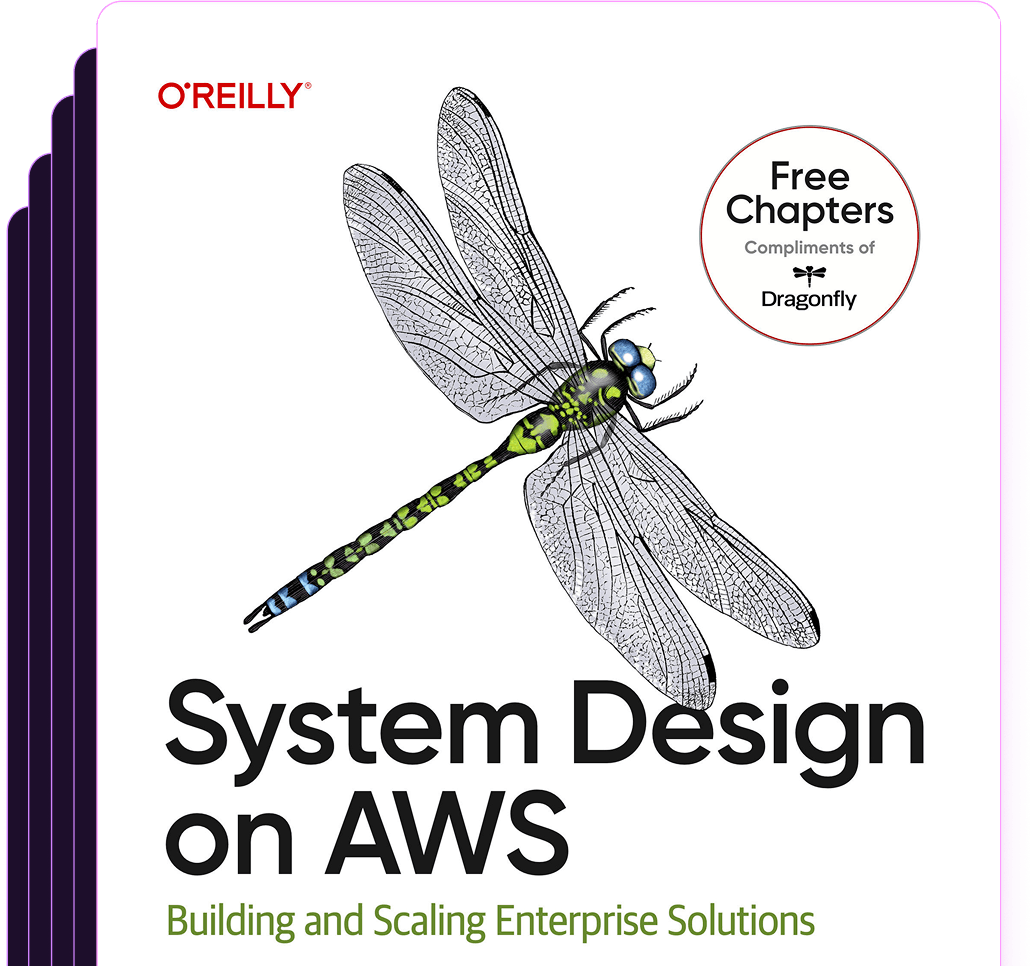
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost