Error: redis timeout exception c#
Solution
Resolving "Redis Timeout Exception" in C#
This error occurs when your C# application fails to receive a response from the Redis server within the specified timeout period. Here are specific steps to diagnose and resolve the issue.
Immediate Diagnostics
- Check Redis Server Status
```sh
redis-cli ping
```
If it doesn't return "PONG", your Redis server might be down. - Monitor Redis Performance
```sh
redis-cli --stat
```
This shows real-time statistics. Look for high memory usage or slow response times.
Common Causes and Solutions
- Insufficient Timeout Value
- Increase the timeout in your C# code:
```csharp
var options = ConfigurationOptions.Parse("localhost:6379");
options.ConnectTimeout = 5000; // 5 seconds
options.SyncTimeout = 5000; // 5 seconds
var redis = ConnectionMultiplexer.Connect(options);
```
- Network Latency
- Test network latency:
```sh
ping your-redis-server-ip
``` - If latency is high, consider moving your app closer to the Redis server.
- Redis Server Overload
- Check Redis INFO command output:
```sh
redis-cli info
``` - Look for high "used_memory" or "connected_clients"
- Consider scaling your Redis setup (e.g., Redis Cluster)
- Large Data Operations
- Use pipelining for bulk operations:
```csharp
var batch = db.CreateBatch();
var tasks = new List<Task>();
for (int i = 0; i < 1000; i++)
{
tasks.Add(batch.StringSetAsync($"key:{i}", $"value:{i}"));
}
batch.Execute();
await Task.WhenAll(tasks);
```
- Connection Pool Exhaustion
- Implement proper connection management:
```csharp
var redis = ConnectionMultiplexer.Connect("localhost");
// Reuse this instance across your application
```
- Slow Lua Scripts
- Optimize your Lua scripts
- Use KEYS and ARGV arrays instead of global variables
C# Code Optimizations
- Implement Retry Logic
```csharp
public async Task<string> GetWithRetry(string key, int maxRetries = 3)
{
for (int i = 0; i < maxRetries; i++)
{
try
{
return await _database.StringGetAsync(key);
}
catch (RedisTimeoutException)
{
if (i == maxRetries - 1) throw;
await Task.Delay(1000 * (i + 1)); // Exponential backoff
}
}
throw new Exception("Unexpected code path");
}
``` - Use Asynchronous Operations
```csharp
await database.StringSetAsync("key", "value");
var value = await database.StringGetAsync("key");
``` - Implement Circuit Breaker
```csharp
// Using Polly library
var policy = Policy
.Handle<RedisTimeoutException>()
.CircuitBreakerAsync(3, TimeSpan.FromMinutes(1));
await policy.ExecuteAsync(async () =>
{
return await _database.StringGetAsync("key");
});
```
- Monitor and Log Redis Operations
```csharp
redis.ConnectionFailed += (sender, e) =>
{
Console.WriteLine("Connection failed: " + e.Exception);
};
redis.ConnectionRestored += (sender, e) =>
{
Console.WriteLine("Connection restored");
};
```
By implementing these specific solutions and code optimizations, you should be able to significantly reduce or eliminate Redis timeout exceptions in your C# application. Remember to monitor your application's performance and Redis server's health regularly to prevent future issues.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Errors (with Solutions)
- could not connect to redis at 127.0.0.1:6379: connection refused
- redis error server closed the connection
- redis.exceptions.responseerror: value is not an integer or out of range
- redis.exceptions.responseerror moved
- redis.exceptions.responseerror noauth authentication required
- redis-server failed to start advanced key-value store
- spring boot redis unable to connect to localhost 6379
- unable to configure redis to keyspace notifications
- redis.clients.jedis.exceptions.jedismoveddataexception
- could not get resource from pool redis
- failed to restart redis service unit redis service not found
- job for redis-server.service failed because a timeout was exceeded
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
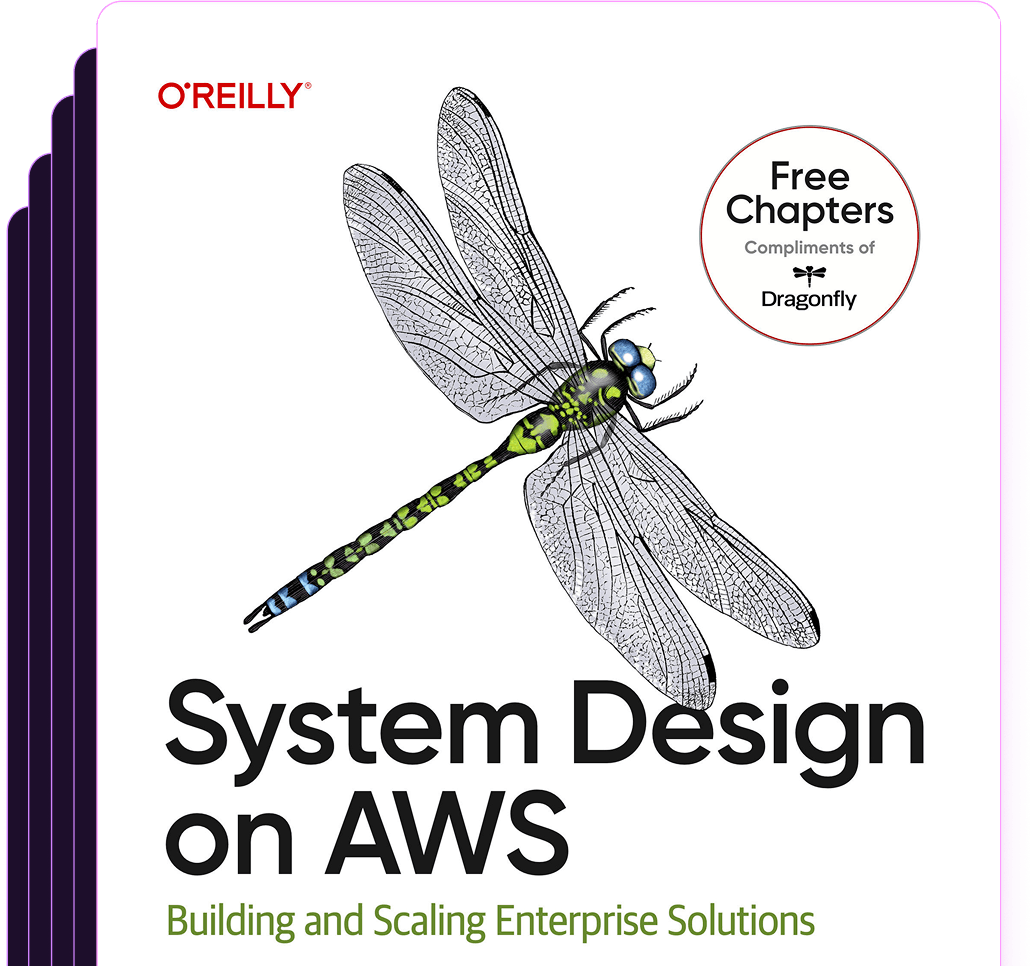
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost