Question: Should I Use Redis or MySQL for Game Development?
Answer
Both Redis and MySQL have their own unique strengths and are suited to different tasks in game dev. Your choice will depend on your specific needs:
MySQL is a relational database management system that uses structured query language (SQL) for managing its data. It is great for situations where you need ACID compliance (Atomicity, Consistency, Isolation, Durability), complex queries, and the ability to handle relations between entities. If your game heavily relies on complex databases and relationships between entities (like an MMORPG with tons of items, NPC characters, player stats, etc.), MySQL would be a good fit.
For example, storing player records could look like this:
CREATE TABLE Players (
PlayerID INT AUTO_INCREMENT,
Name VARCHAR(100),
Score INT,
PRIMARY KEY (PlayerID)
);
Redis, on the other hand, is an open-source, in-memory data structure store used as a database, cache, and message broker. Redis has a reputation for speed as it operates on in-memory datasets. If you need caching, session management, real-time analytics, or job & queue management, Redis would be the go-to choice. Let's say you have a real-time multiplayer game where speed is crucial; using Redis to manage player sessions would likely improve performance.
A simple way to set and get key-value pairs in Redis using Python would be:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
# set a value
r.set('Player1', '100') # sets Player1 score as 100
# get a value
print(r.get('Player1')) # gets and prints the score of Player1
However, it's important to note that these systems can, and often do, coexist within the same application. For instance, MySQL could be used to store game state and player profiles, while Redis is used for real-time leaderboards.
The best choice for your game depends on the specific requirements, the nature of the interaction with the data, and the scale at which your game operates.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Dev Questions (and Answers)
- Do Game Engines Cost Money?
- Can I Use an SQL Database for Game Development?
- How are databases used in game development?
- How do you save multiplayer game data, in a database or a file?
- How can you design an efficient database for a game?
- What are the differences between using a database and JSON for games?
- Do Video Games Use Databases?
- Does game development require knowledge of mathematics?
- Should I Use a Game Engine or Not?
- Is a game engine considered a framework?
- Do you need a game engine to make a game?
- How can you design a game leaderboard system?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
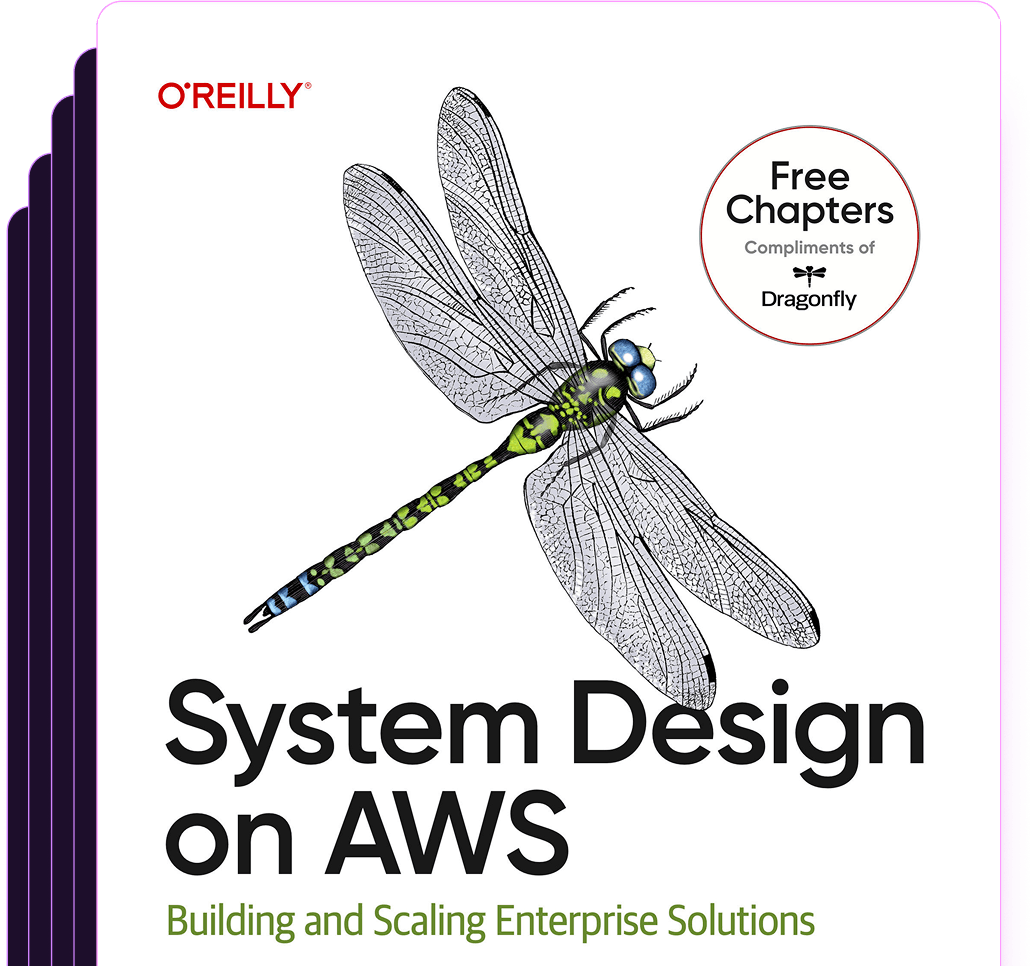
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost