Question: How can Redis be used for a game server?
Answer
Redis can be a valuable asset for game development, particularly in the context of handling real-time gaming scenarios. It's a high-performance in-memory database system that offers data structures and functionalities such as strings, hashes, lists, sets, sorted sets, bitmaps, hyperloglogs, and more.
Here are some ways Redis can be used specifically for game servers:
- Session Store: Redis can store session data like player profiles, game states, and other transient information.
- Leaderboards and Rankings: With sorted sets, Redis can easily manage real-time leaderboards.
- Real-Time Analytics: Redis can process large amounts of data in real time, making it suitable for live analytics.
- Pub/Sub & Messaging: Redis supports Publish/Subscribe patterns to deliver messages among various parts of your application or between multiple users.
- Rate Limiting: Use Redis to limit actions in your game, for example, limit API calls or gameplay actions per user.
Here's an example of how you might use Redis to maintain a leaderboard for a game server in Python:
import redis
# Initialize a Redis client
r = redis.Redis(host='localhost', port=6379, db=0)
# Add players with their scores.
r.zadd('leaderboard', {'player1': 2300})
r.zadd('leaderboard', {'player2': 2100})
r.zadd('leaderboard', {'player3': 2200})
# Get top 2 players
top_players = r.zrevrange('leaderboard', 0, 1, withscores=True)
for player, score in top_players:
print(f"{player.decode('utf-8')}: {score}")
In this example, we use the zadd
method to add players with their scores on a leaderboard. The zrevrange
command is then used to fetch the top players from the leaderboard.
Remember, as Redis is an in-memory database, it's best suited for transient data that does not need to be stored permanently. For persistent data storage, consider pairing Redis with traditional databases like MySQL or PostgreSQL.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Dev Questions (and Answers)
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
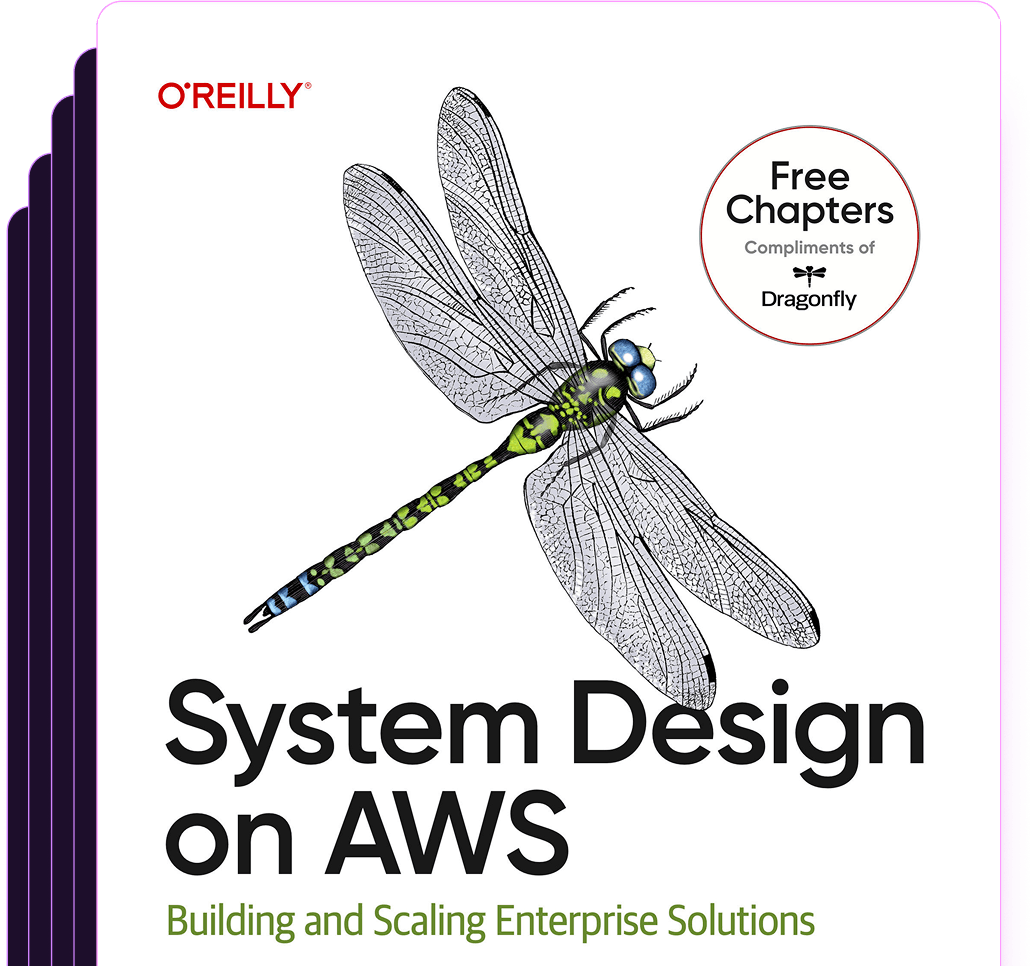
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost