Redis Sorted Set: Get Highest Score (Detailed Guide w/ Code Examples)
Use Case(s)
- Retrieving the member with the highest score in a game leaderboard.
- Finding the most popular item based on voting or rating systems.
- Identifying the latest entry in a time-series dataset where scores represent timestamps.
Code Examples
Python
Using the zrevrange
command to get the member with the highest score:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
highest_score_member = r.zrevrange('my_sorted_set', 0, 0, withscores=True)
print(highest_score_member)
Node.js
Using the zrevrange
command with the ioredis
library:
const Redis = require('ioredis');
const redis = new Redis();
redis.zrevrange('my_sorted_set', 0, 0, 'WITHSCORES').then((result) => {
console.log(result);
});
Golang
Using the ZRANGE
command with the go-redis
library:
package main
import (
"fmt"
"github.com/go-redis/redis/v8"
"context"
)
func main() {
ctx := context.Background()
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
})
result, err := rdb.ZRevRangeWithScores(ctx, "my_sorted_set", 0, 0).Result()
if err != nil {
panic(err)
}
fmt.Println(result)
}
Best Practices
- Ensure that your sorted sets are properly indexed to avoid performance degradation when querying large datasets.
- Use appropriate key namespacing to prevent conflicts in large-scale applications.
Common Mistakes
- Forgetting to include the
WITHSCORES
option to retrieve both the members and their scores. - Misinterpreting the
zrevrange
command's range parameters (start and stop are inclusive).
FAQs
Q: What is the difference between ZRANGE
and ZREVRANGE
? A: ZRANGE
retrieves elements in ascending order by score, while ZREVRANGE
retrieves them in descending order.
Q: How can I efficiently remove the highest score after retrieving it? A: Use a transaction (MULTI
/EXEC
) to ensure atomicity when getting and removing the element with the highest score.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Redis Sorted Set: Create
- Redis Sorted Set: Get by Key
- Redis Sorted Set: Limit Size
- Redis Sorted Set: Same Score
- Redis Sorted Set: Sorting by Multiple Fields
- Redis Sorted Set TTL
- Redis Sorted Set: Sort by Date
- Redis Sorted Set: Expire Key
- Redis Sorted Set: Check Exists
- Redis Sorted Set: Remove by Score
- Redis Sorted Set: Rate Limit
- Redis Sorted Set: Custom Order
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
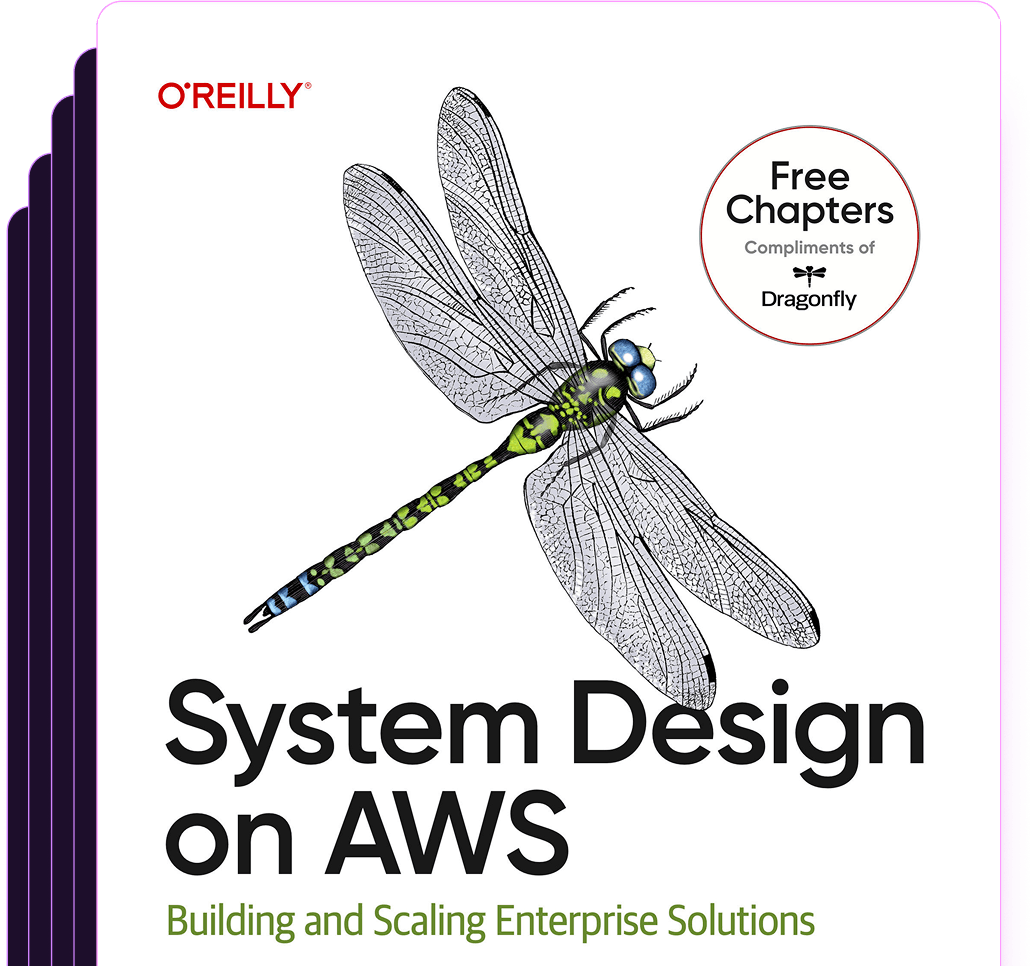
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost