Redis Sorted Set: Limit Size (Detailed Guide w/ Code Examples)
Use Case(s)
- Maintaining a leaderboard with a fixed number of top scores.
- Keeping track of the latest N items in a log or feed.
- Enforcing size constraints on sorted sets to manage memory usage efficiently.
Code Examples
To limit the size of a sorted set, you can use the ZADD
command followed by the ZREMRANGEBYRANK
command to remove elements outside the desired range.
Python: CODE_BLOCK_PLACEHOLDER_0
Node.js: CODE_BLOCK_PLACEHOLDER_1
Golang: CODE_BLOCK_PLACEHOLDER_2
Best Practices
- Regularly trim your sorted sets to enforce size limits and prevent uncontrolled growth.
- Use appropriate data expiration strategies and Redis persistence options to manage large datasets effectively.
Common Mistakes
- Forgetting to handle potential errors from Redis commands, which can lead to unexpected behavior in your application.
- Incorrectly calculating the range for
ZREMRANGEBYRANK
, leading to unintended deletions.
FAQs
Q: What happens if the sorted set is smaller than the specified maximum size? A: If the sorted set has fewer elements than the maximum size, the ZREMRANGEBYRANK
command will effectively do nothing, leaving the sorted set unchanged.
Q: Is there a way to atomically add an element and trim the sorted set in one operation? A: Redis does not support atomic operations for adding and trimming in a single command. However, using Lua scripting, you can achieve atomicity for more complex operations.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Redis Sorted Set: Get Highest Score
- Redis Sorted Set: Create
- Redis Sorted Set: Get by Key
- Redis Sorted Set: Same Score
- Redis Sorted Set: Sorting by Multiple Fields
- Redis Sorted Set TTL
- Redis Sorted Set: Sort by Date
- Redis Sorted Set: Expire Key
- Redis Sorted Set: Check Exists
- Redis Sorted Set: Remove by Score
- Redis Sorted Set: Rate Limit
- Redis Sorted Set: Custom Order
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
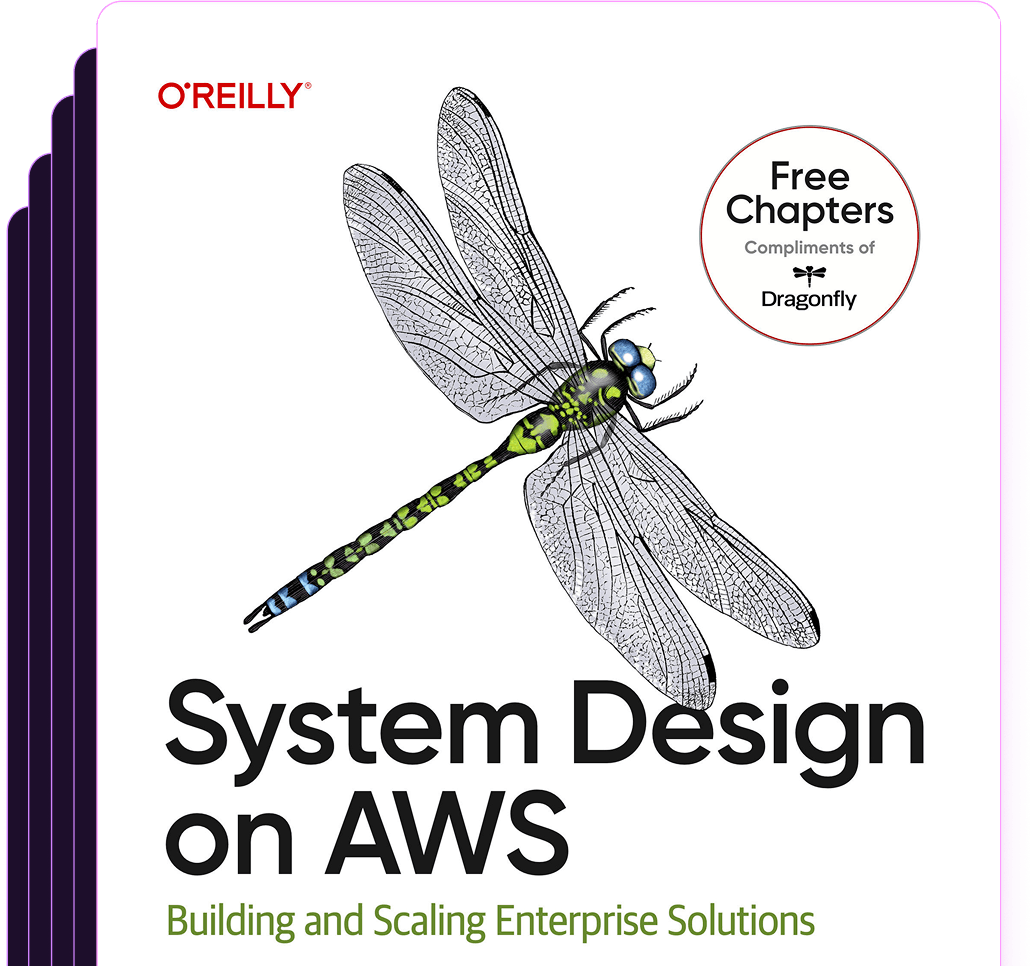
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost