Redis Sorted Sets - Everything You Need To Know
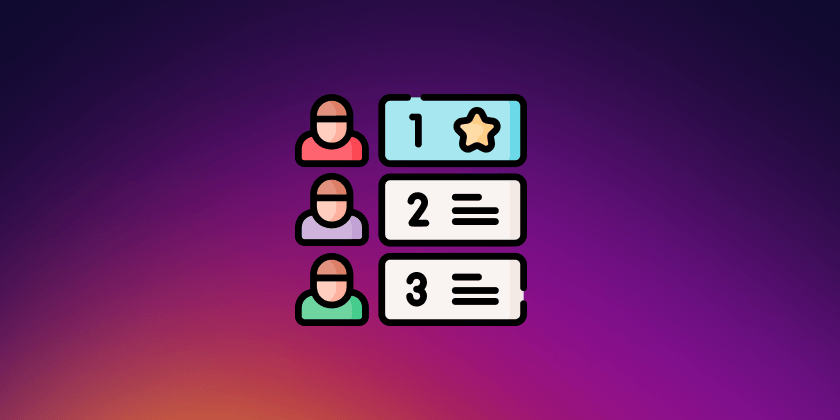
What are Redis Sorted Sets?
Redis, the highly acclaimed in-memory data structure store, is known for its versatility and speed. Among its various data structures, Sorted Sets stand out for their unique capabilities. This section of our post will dive deep into what Sorted Sets are, compare them with other Redis data structures, and explore some practical applications.
Definition and Characteristics of Sorted Sets
A Redis Sorted Set, often abbreviated as ZSET, is a collection that combines elements of both sets and hash tables. Each member in a Sorted Set is unique, but unlike regular sets, every member is associated with a score. This score is used to order the members from the smallest to the largest score. These scores can also be updated in real time, which makes Sorted Sets dynamic and flexible.
Here's a simple example of how you might add items to a Sorted Set in Redis:
ZADD myzset 1 "one"
ZADD myzset 2 "two"
ZADD myzset 3 "three"
In this example, "one"
, "two"
, and "three"
are the members each associated with scores 1
, 2
, and 3
, respectively.
Characteristics that set apart Sorted Sets include:
- Automatic ordering: Members are always sorted based on their score.
- Unique Members: No two members can be identical, reinforcing set-like behavior.
- Efficiency: Operations like adding, removing, or updating the score of an element have logarithmic complexity.
Comparison with Other Redis Data Structures (Like Lists, Hashes, and Sets)
To appreciate the uniqueness of Sorted Sets, let's compare them with other Redis data structures:
- Lists: Redis Lists are simply lists of strings, sorted by insertion order. Unlike Sorted Sets, they do not allow for easy sorting or scoring of the elements. Lists are ideal for scenarios where insertion order matters, such as messaging queues.
- Hashes: Hashes in Redis are key-value pairs within a single key. They are perfect for representing objects (like a user with multiple attributes) but do not support automatic sorting by values or keys.
- Sets: Regular Sets are similar to Sorted Sets but lack the scoring feature. They are unordered collections of unique elements which makes them suitable for membership testing and intersection/union operations, whereas Sorted Sets are excellent when the order of elements is crucial.
Common Use Cases for Sorted Sets
Sorted Sets are incredibly versatile. Here are a few scenarios where they shine:
- Leaderboards: By storing user scores as scores in a Sorted Set, you can easily retrieve a leaderboard. You can quickly update scores in real-time and fetch top or bottom N users efficiently.
Example: Update user score and fetch top 3 users.
ZADD leaderboard 500 "user123"
ZADD leaderboard 450 "user456"
ZADD leaderboard 530 "user789"
ZRANGE leaderboard 0 2 WITHSCORES
This command sequence first updates/adds scores for three users and then retrieves the top three users from the leaderboard.
- Priority Queues: Tasks can be added to a Sorted Set with their priority as the score, allowing higher priority tasks to be processed first.
- Access Data in Order: Whether it's time-series data or just a set of items that need to be accessed sequentially, Sorted Sets provide an efficient way to do so.
Understanding and leveraging the capabilities of Redis Sorted Sets can significantly enhance the performance and functionality of your applications, especially when dealing with ranked or ordered data.
How Redis Sorted Sets Work
The Underlying Data Structure (Skip List)
At their core, Redis sorted sets are powered by a data structure called a skip list. Skip lists are an ingenious probabilistic alternative to balanced trees. They allow for average time complexity of O(log N) for most operations — such as search, insert, delete — which makes them exceptionally efficient for the kinds of operations sorted sets need to perform.
A skip list consists of multiple levels of linked lists. The base level contains all the elements of the set, and each higher level acts like an "express lane" that skips several elements below, thus allowing faster traversal of the list. The decision to include an element in these higher levels is random, ensuring that the structure remains balanced without complex rebalancing routines.
How Elements Are Stored and Retrieved
In Redis, every element in a sorted set is stored with a score that dictates its order. This pairing of value and score ensures that the set remains sorted, even as elements are added or removed. When you query a sorted set, Redis uses the scores as well as the skip list structure to efficiently find and retrieve elements.
For example, if you want to retrieve elements with scores between 50 and 60, Redis starts at the head of the skip list and follows the links that offer the quickest path to the first element in this range, then continues sequentially until it surpasses the score of 60.
Uniqueness and Scoring of Elements (with Examples)
Let’s illustrate the functionality and efficiency of sorted sets with some practical examples. Each element in a Redis sorted set must be unique, but they can have identical scores. In queries, if multiple elements share the same score, they are further sorted lexicographically.
Here’s a basic example:
import redis
# Connect to Redis server
r = redis.Redis(host='localhost', port=6379, db=0)
# Adding elements to a sorted set
r.zadd('scores', {'Alice': 67, 'Bob': 85, 'Carol': 67, 'Dave': 91})
# Retrieving all elements with their scores
print(r.zrange('scores', 0, -1, withscores=True))
# Output: [(b'Alice', 67.0), (b'Carol', 67.0), (b'Bob', 85.0), (b'Dave', 91.0)]
In the above code, we add four elements to a sorted set named scores
. Note that both Alice and Carol have the same score of 67. When retrieved, they are ordered lexicographically within their score group.
Another example demonstrates how to manipulate ranges:
# Get elements with scores between 70 and 90
print(r.zrangebyscore('scores', 70, 90, withscores=True))
# Output: [(b'Bob', 85.0)]
These examples help underline the real-world applicability of Redis sorted sets in managing ordered data efficiently, particularly useful in scenarios such as leaderboards, event scheduling, or maintaining indexed access to dataset elements based on custom scoring criteria.
By understanding these fundamental concepts and execution examples, you can leverage the full potential of Redis sorted sets in your applications, ensuring optimal performance and scalability.
Core Commands in Redis Sorted Sets
Overview of Basic Commands (e.g., ZADD, ZRANGE, ZREM)
Sorted sets in Redis are non-repeating collections of strings, each associated with a floating point number called a score. This pairing allows the set to be sorted from the smallest to the largest score. Here are some fundamental commands:
- ZADD: Adds one or more members to a sorted set, or updates their score if they already exist.
- ZRANGE: Retrieves a subset of elements within a given range of indices, sorted by their scores.
- ZREM: Removes one or more members from a sorted set.
These commands form the backbone of interaction with sorted sets in Redis.
Detailed Examples of Each Command (with Syntax and Usage)
Let's explore each of these commands with practical examples:
1. ZADD
Syntax: ZADD key [NX|XX] [CH] [INCR] score member [score member ...]
NX
: Only add new elements. Don't update already existing elements.XX
: Only update elements that already exist. Don’t add new elements.CH
: Modify the return value to be the number of elements changed (new elements added plus elements updated).INCR
: Increment the score of an element instead of setting it.
Example:
ZADD myset 1 "apple"
ZADD myset 2 "banana" 3 "cherry"
This command will add "apple" with a score of 1, "banana" with a score of 2, and "cherry" with a score of 3 to "myset".
2. ZRANGE
Syntax: ZRANGE key start stop [WITHSCORES]
WITHSCORES
: Include scores of the returned elements.
Example:
ZRANGE myset 0 -1 WITHSCORES
This retrieves all elements in "myset" along with their scores.
3. ZREM
Syntax: ZREM key member [member ...]
Example:
ZREM myset "apple"
This removes "apple" from "myset".
Advanced Commands and Options (e.g., ZRANK, ZREVRANGE, ZINCRBY)
As you grow more comfortable with sorted sets, you’ll find these advanced commands incredibly useful:
- ZRANK: Returns the rank (index) of a member in the set, sorted by score.
- ZREVRANGE: Returns the specified range of elements in the set, in descending order by score.
- ZINCRBY: Increments the score of a member in the sorted set.
Examples:
1. ZRANK
Syntax: ZRANK key member
Example:
ZRANK myset "banana"
This retrieves the rank of "banana" within "myset".
2. ZREVRANGE
Syntax: ZREVRANGE key start stop [WITHSCORES]
Example:
ZREVRANGE myset 0 1 WITHSCORES
This command returns the top two scoring members and their scores from "myset".
3. ZINCRBY
Syntax: ZINCRBY key increment member
Example:
ZINCRBY myset 10 "banana"
Increases the score of "banana" by 10.
Using these commands effectively enables seamless management of ranked data in real-time applications such as leaderboards, priority queues, and more. By mastering Redis sorted sets, you enhance not only your application's performance but also its capability to handle complex data structures efficiently.
Real-World Application Scenarios
Case Study 1: Implementing Real-Time Leaderboards
Problem statement:
Online gaming platforms and social media sites often need to display user rankings based on scores or activities, updated in real time. Traditional databases might struggle with the high volume and velocity of write and read operations required for such features.
Solution using sorted sets:
Redis sorted sets are ideal for this scenario because they automatically maintain elements in a sorted order by score. This feature drastically simplifies the implementation of leaderboards, where scores are constantly updated and rankings need to be retrieved quickly.
Code snippets and explanations:
Here's how you can implement a basic leaderboard system using Redis sorted sets:
- Adding Scores: To add or update scores, use the
ZADD
command. It adds members to the sorted set stored at key, or updates their score if it already exists.
```bash
ZADD leaderboard 4500 "user123"
ZADD leaderboard 5300 "user456"
```
In these commands, leaderboard
is the key of the sorted set, each user has a score (e.g., 4500
), and a unique identifier (e.g., "user123"
).
- Fetching Top Users: To get the top 10 users, use the
ZREVRANGE
command along with their scores:
```bash
ZREVRANGE leaderboard 0 9 WITHSCORES
```
This command retrieves members ranked from highest to lowest score. The option WITHSCORES
tells Redis to return both the member and its score.
- Handling Real-Time Changes: Since sorted sets keep the data sorted, adding a new score or updating an existing one doesn’t require any additional operation to maintain the order. Reads will always retrieve the latest, correct order of users.
- Listening for Changes: Although directly not a function of sorted sets, you can utilize Redis Pub/Sub features to notify subscribers whenever a score is updated, keeping frontend leaderboards accurate in real time.
Case Study 2: Efficient Data Range Queries
Problem statement:
Applications that offer services like event scheduling or price comparisons need to handle queries for records within specific ranges, efficiently and quickly.
Solution using sorted sets:
Sorted sets provide a built-in way to quickly access data ranges based on scores. This is perfect for scenarios where you need to query data within a certain range of dates or prices.
Step-by-step implementation guide:
Let’s consider an application that allows users to look up events within a specific date range:
- Storing Events: Use scores in UNIX timestamp format to represent event dates, allowing easy range queries.
```bash
ZADD events 1597849261 "event1"
ZADD events 1598032061 "event2"
```
Here, events
is the sorted set, and each event has a corresponding UNIX timestamp as its score.
- Querying Events by Date Range: To find all events happening between two specific timestamps:
```bash
ZRANGEBYSCORE events 1597800000 1598200000
```
This command fetches all events with scores (timestamps) between 1597800000
and 1598200000
.
- Efficiency: The use of sorted sets ensures that these queries are executed swiftly, as Redis keeps the set sorted by the score. This avoids the need for full scans of the data.
By leveraging Redis sorted sets in these ways, developers can achieve high performance and maintainability for features that rely heavily on ordered data and range queries.
Sorted Sets - Performance Considerations
Performance Benefits of Using Sorted Sets
Sorted Sets, or ZSETs
in Redis, are a non-trivial data structure that provide a unique combination of versatility and performance. They store unique elements but each element is associated with a score that determines the order. This characteristic enables both fast access (read/write) and maintaining an ordered dataset which is crucial for use cases like leaderboards, priority queues, and time series data.
Key Performance Benefits:
- Logarithmic Complexity for Inserts and Deletes: Operations like adding or removing elements have a time complexity of O(log(N)), where N is the number of elements in the sorted set. This makes it very efficient even for large datasets.
- Fast Access to Elements by Score or Rank: Finding elements within a score range or accessing elements based on their rank benefits from the underlying sorted nature of ZSETs. These operations typically run in O(log(N)+M) time complexity where M is the number of elements being returned.
- Combination Operations: Redis allows performing intersection and union operations on multiple sorted sets, which are optimized and faster compared to equivalent operations in other data structures.
These features make sorted sets an optimal choice for real-time applications where high performance is critical and data needs to be maintained in a sorted order without additional computational overhead.
Tips for Optimizing Sorted Set Operations
To fully leverage the power of Redis Sorted Sets, here are some optimization tips:
- Use Appropriate Data Sizes: While Redis handles large data sizes quite efficiently, keeping the data size optimal ensures better memory management and faster backups. For instance, consider storing only necessary precision in scores or splitting very large sorted sets into smaller ones if feasible.
- Leverage Pipelining: When executing multiple commands, use pipelining to reduce the number of round trips between the client and server. This is particularly useful for bulk insertions or updates.
- Memory Optimization: Redis provides options to configure how much memory to use for storing data. Using data compression techniques and regularly evaluating your memory usage can prevent running out of memory.
- Regular Maintenance: Periodic cleanup tasks to remove outdated items from sorted sets can help maintain performance. Setting TTL (Time to Live) for elements can automatically handle this.
Example for adding bulk data using pipelining in Python:
import redis
r = redis.Redis()
pipeline = r.pipeline()
for i in range(1000):
pipeline.zadd('myzset', {'member'+str(i): i})
pipeline.execute()
Limitations and When Not to Use Sorted Sets
While sorted sets are highly efficient, they are not always the best tool for every job. Understanding their limitations helps in choosing the right data structure for the right task.
Considerations Before Using Sorted Sets:
- Memory Usage: Each element in a sorted set requires additional memory for the score and internal pointers, making it more memory-intensive than simpler data structures like lists or sets.
- Not Suitable for Highly Volatile Data: If the data changes too frequently, the overhead of updating the scores and the rank might overshadow the benefits of sorted retrieval.
- Complexity in Handling Non-Unique Values: Since sorted sets require unique members, managing non-unique values requires additional logic — such as appending unique identifiers to values.
In conclusion, while Redis Sorted Sets offer remarkable speed and efficiency for ordered data, they should be used judiciously, considering both the application's specific needs and the inherent characteristics of the data involved. The key to effective use lies in understanding both the strengths and limitations of sorted sets, ensuring that they are a true fit for the problem at hand.
Conclusion
Redis sorted sets offer an incredibly powerful and flexible way to handle sorted data, making them indispensable for many applications that require high performance and precise ordering of items. With the comprehensive set of commands available, you can manage, query, and manipulate your data efficiently, unlocking a wide range of possibilities for real-time data processing and analytics. This guide has equipped you with the knowledge to harness the full potential of Redis sorted sets, setting you up for success in your next data-driven project.
FAQs
Code Examples
Code Examples by Command
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost