Question: How can you implement a delay in BullMQ job queues?
Answer
In BullMQ, you can implement a delay in job queues using the delay
option when adding jobs. The delay
option allows you to specify a time duration (in milliseconds) that BullMQ should wait before processing the job.
Here's an example:
const Queue = require('bullmq').Queue;
const queue = new Queue('my-queue');
async function addJob() {
await queue.add(
'my-job',
{ foo: 'bar' },
{ delay: 5000 } // Delay job by 5 seconds
);
}
addJob()
.then(() => console.log('Job added'))
.catch(err => console.error(err));
In this example, we're adding a job named 'my-job'
with data { foo: 'bar' }
to the queue called 'my-queue'
. The job will not be available for processing until 5 seconds (5000 milliseconds) have passed.
Note, the actual delay may be longer than the specified delay time due to how the event loop scheduling works in Node.js. Also remember, the delay is relative to when queue.add()
is called, not when the job reaches the front of the queue.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common BullMQ Questions (and Answers)
- What are the differences between BullMQ and Amazon SQS?
- What are the key differences between BullMQ and Agenda?
- What is the difference between BullMQ and RabbitMQ?
- What are the differences between BullMQ and Bull in job queueing?
- What are the differences between BullMQ and Celery?
- How can I use multiple consumers with BullMQ?
- How can I monitor the health of my BullMQ queue?
- How can I use BullMQ for job queue management in Node.js?
- What is the architecture of BullMQ?
- How can you handle errors in BullMQ?
- What are the differences between BullMQ and Kafka?
- What are some best practices for using BullMQ?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
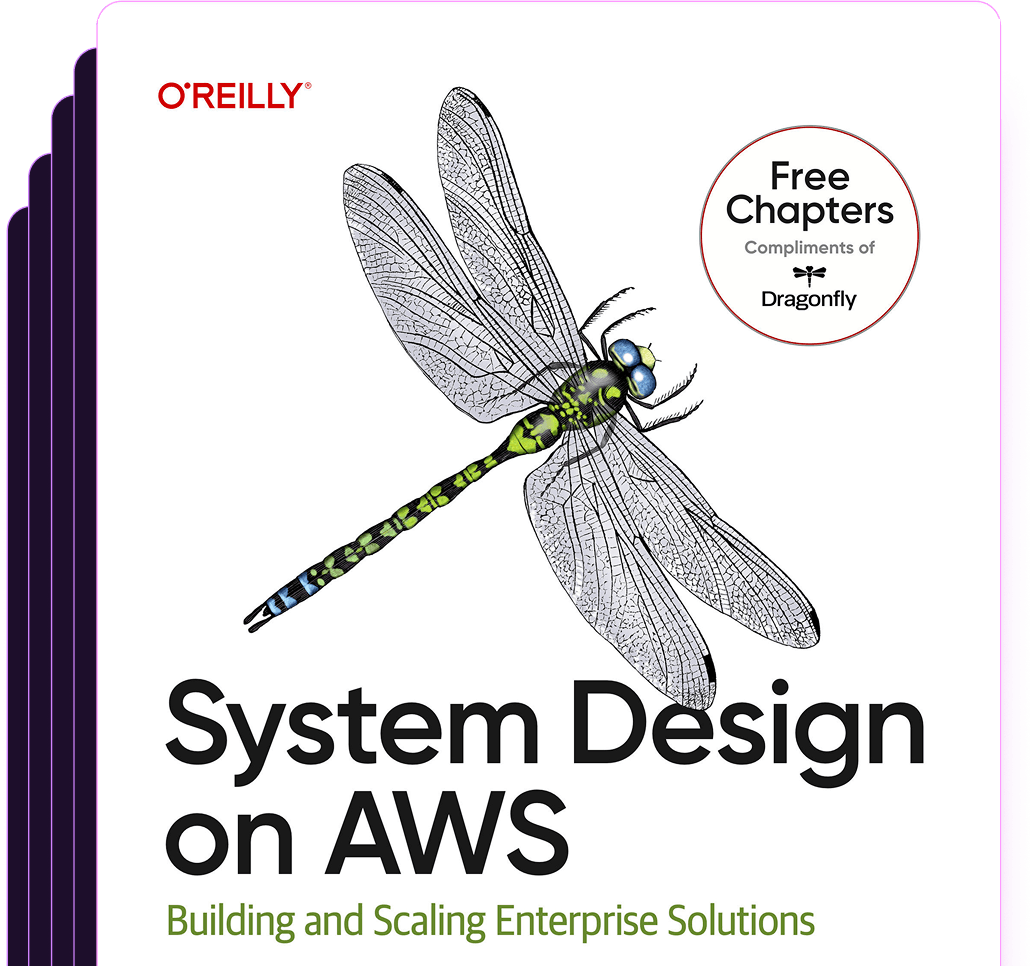
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost