Question: What are the differences between BullMQ and Kafka?
Answer
"BullMQ and Kafka both serve as message brokers but they're designed to meet different needs and thus have some key differences.
- Language Support: BullMQ is a Node.js based job and message queue library, so it's a great choice if you're working predominantly with JavaScript. On the other hand, Apache Kafka has client libraries available for multiple languages such as Java, Python, C#, Go, etc.
- Persistence: Both provide persistent storage of messages until they're consumed. However, Kafka retains all messages for a set period of time (a week by default) regardless of whether they've been processed or not, enabling reprocessing. On the contrary, BullMQ will remove jobs once they are completed, unless specified to keep them.
- Distributed System : Kafka is a distributed streaming platform which can handle trillions of messages in a day, thus it is more suitable for big data use cases where massive amounts of real-time data need to be processed. BullMQ does not inherently support this level of distribution.
- Use Cases: Kafka is typically used for stream processing, event sourcing, log aggregation and analysis. It is ideal for building real-time data pipelines that reliably move data between systems. Meanwhile, BullMQ is excellent for handling background jobs: tasks that should be performed outside of your application’s main flow, and that are resource-intensive or time-consuming.
- Setup and Maintenance: Setting up Kafka and maintaining it can be complex due to its distributed nature while BullMQ is relatively easier to setup and get started with.
Here's an example of how to create a job in BullMQ:
const Queue = require('bullmq').Queue;
const myQueue = new Queue('Paint the bikeshed');
myQueue.add('green', {paint: 'lime'});
And in Kafka, producing messages looks more like this:
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
ProducerRecord<String, String> record = new ProducerRecord<>("test", "key", "value");
producer.send(record);
producer.close();
Overall, the choice between BullMQ and Kafka depends on your specific use case, programming language, and the scale of data you're intending to process."
Was this content helpful?
Help us improve by giving us your feedback.
Other Common BullMQ Questions (and Answers)
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
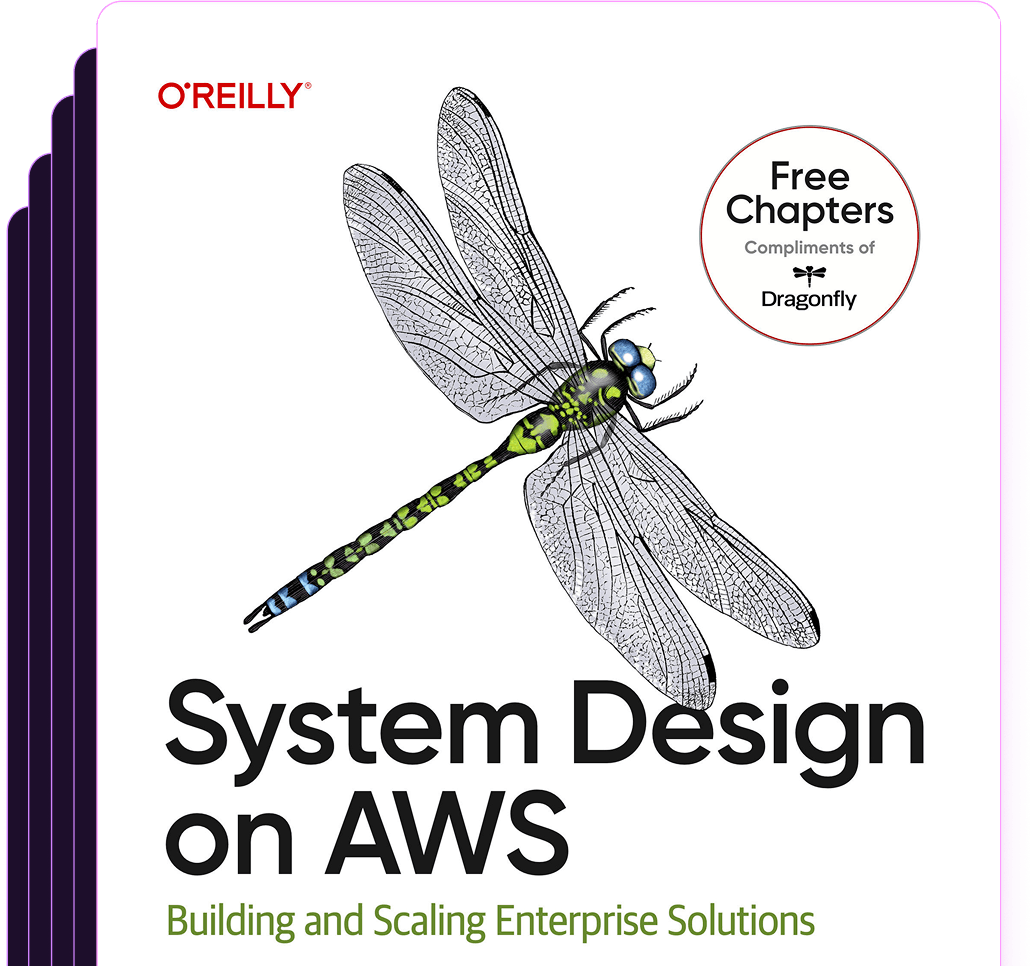
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost