Question: What are the key differences between BullMQ and Agenda?
Answer
Both BullMQ and Agenda are popular task scheduling libraries for Node.js applications, but they have different features and strengths that might make one more suitable than the other depending on the specific use case. Here are some of the key differences:
1. Backing Store:
- BullMQ: Uses Redis as its backing store. This makes it extremely fast and capable of handling a large number of jobs efficiently.
- Agenda: Uses MongoDB as its backing store. While not as speedy as Redis, MongoDB provides rich querying capabilities.
// Creating BullMQ queue
const Queue = require('bull');
const myQueue = new Queue('myQueue', 'redis://localhost:6379');
// Creating Agenda job
const Agenda = require('agenda');
const agenda = new Agenda({db: {address: 'mongodb://localhost/agenda-db'}});
2. Priority Support:
- BullMQ: Supports priority-based job processing. You can set a priority value when you add a job to the queue.
- Agenda: Does not natively support priority-based job processing.
// Add job with priority in BullMQ
myQueue.add({email: 'example@email.com'}, {priority: 1});
3. Job Types:
- BullMQ: Supports delayed jobs, repeatable jobs, and rate-limited jobs.
- Agenda: Supports delayed jobs, repeatable jobs, but lacks rate-limited job functionality.
// Setting up a repeatable job in BullMQ
myQueue.add({}, {repeat: {cron: '* * * * *'}});
// Setting up a repeatable job in Agenda
agenda.every('1 minute', 'send email job');
4. Robustness and Reliability:
- BullMQ: Offers robust handling of stalled jobs and network issues, although it requires manual setup for job events.
- Agenda: Has built-in support for job events, but could be less robust when dealing with stalled or failed jobs.
Remember, the choice between BullMQ and Agenda will depend on your specific needs, such as the volume of jobs to process, the nature of the jobs, the database system you prefer or already using, etc.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common BullMQ Questions (and Answers)
- What are the differences between BullMQ and Amazon SQS?
- What is the difference between BullMQ and RabbitMQ?
- What are the differences between BullMQ and Bull in job queueing?
- What are the differences between BullMQ and Celery?
- How can I use multiple consumers with BullMQ?
- How can I monitor the health of my BullMQ queue?
- How can I use BullMQ for job queue management in Node.js?
- What is the architecture of BullMQ?
- How can you handle errors in BullMQ?
- What are the differences between BullMQ and Kafka?
- What are some best practices for using BullMQ?
- What are some common use cases of BullMQ?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
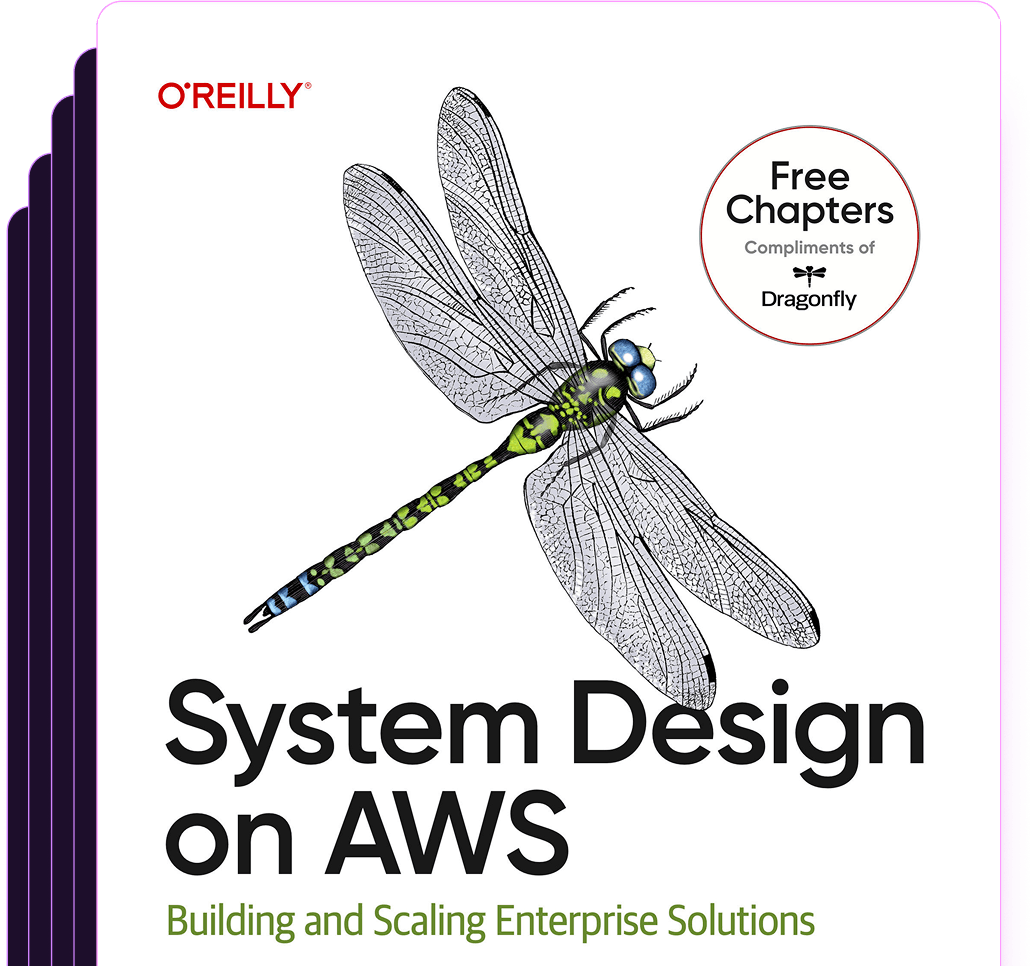
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost