Question: How can I use multiple consumers with BullMQ?
Answer
In BullMQ, you can easily set up multiple consumers to process jobs in a queue. This can be achieved by creating multiple worker instances.
Here is a simple example:
const { Queue, Worker } = require('bullmq');
// Create a queue
const myQueue = new Queue('myQueue');
// Create multiple workers (consumers)
new Worker('myQueue', async job => {
// Process the job data
console.log(job.data);
});
new Worker('myQueue', async job => {
// Process the job data
console.log(job.data);
});
In this code snippet, two workers are created for 'myQueue'. Both workers can process jobs from the queue concurrently. Since each worker is an independent Node.js process, they don't share memory space and can run on different cores or even different machines, thus allowing parallel processing of jobs.
Remember that the processing function (async job => {}
in the example) is called independently in each worker. Therefore, any variables defined outside of this function will not be shared between workers.
Also note that the ordering of job processing cannot be guaranteed when using multiple consumers. If the order of job execution is important, you should consider using other strategies, like named jobs or job dependencies.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common BullMQ Questions (and Answers)
- What are the differences between BullMQ and Amazon SQS?
- What are the key differences between BullMQ and Agenda?
- What is the difference between BullMQ and RabbitMQ?
- What are the differences between BullMQ and Bull in job queueing?
- What are the differences between BullMQ and Celery?
- How can I monitor the health of my BullMQ queue?
- How can I use BullMQ for job queue management in Node.js?
- What is the architecture of BullMQ?
- How can you handle errors in BullMQ?
- What are the differences between BullMQ and Kafka?
- What are some best practices for using BullMQ?
- What are some common use cases of BullMQ?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
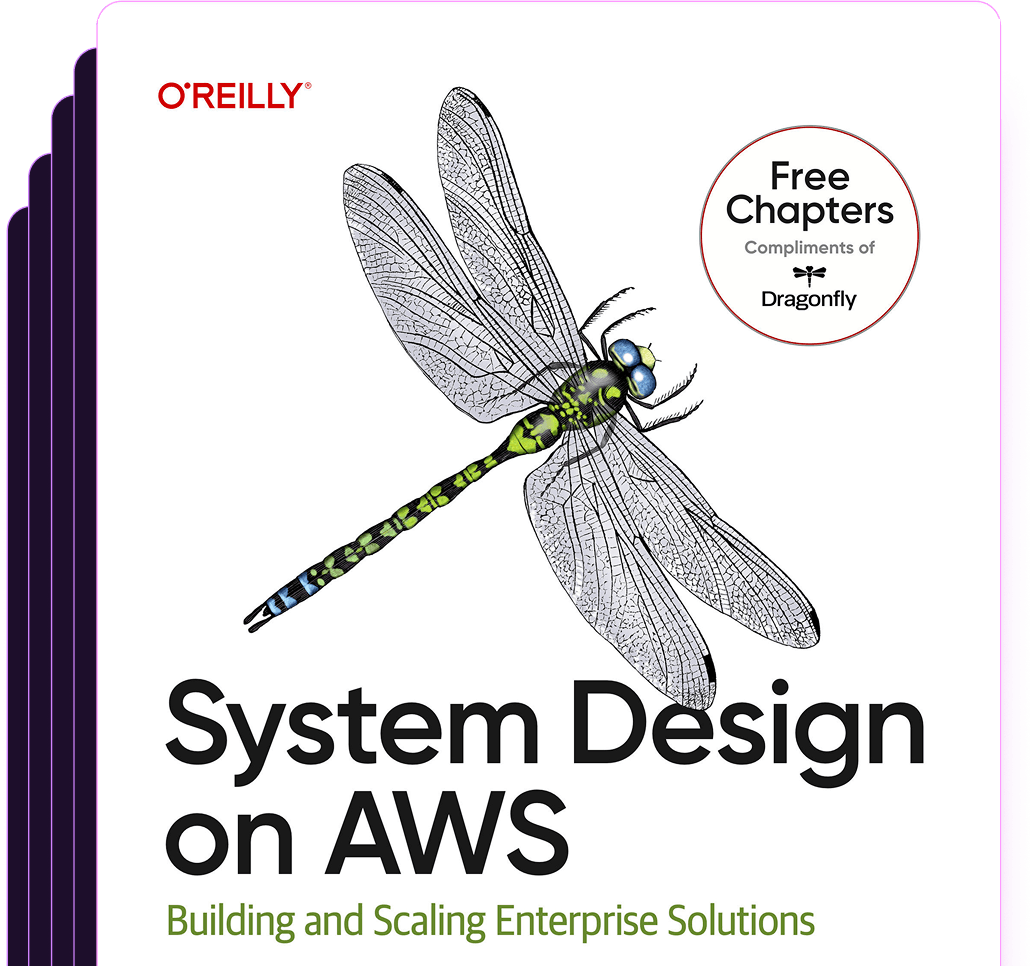
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost