Question: What are some common use cases of BullMQ?
Answer
BullMQ is a fast, reliable and highly customizable priority job queue for Node.js, based on the Redis database. Here are some common use cases of BullMQ:
- Background Jobs: One of the most common use cases of BullMQ is running tasks or jobs in the background that don't need to be executed instantly. This can range from sending emails, processing images, to data analytics and more.
const Queue = require('bull');
const myQueue = new Queue('myQueue');
myQueue.add({
email: 'example@example.com',
subject: 'Welcome',
message: 'Thank you for joining us!'
});
- Delayed Jobs: You can schedule jobs that run after a certain delay. This could be useful for reminders, notifications, or any task that should only be performed after some time.
myQueue.add({
email: 'example@example.com',
subject: 'Subscription ending soon',
message: 'Your subscription will end in 3 days.'
}, {
delay: 3 * 24 * 60 * 60 * 1000 // 3 days in milliseconds
});
- Priority Jobs: If there are jobs that need to be executed before others, BullMQ provides a priority system. Higher priority jobs get processed first.
```javascript
// High priority job
myQueue.add({
email: 'example@example.com',
subject: 'High Priority',
message: 'This is a high priority job'
}, { priority: 1 });
// Low priority job
myQueue.add({
email: 'example@example.com',
subject: 'Low Priority',
message: 'This is a low priority job'
}, { priority: 2 });
```
- Rate Limited Jobs: For tasks that should not be performed too frequently, you can set a rate limit on your job queue.
const rateLimitedQueue = new Queue('emailNotifications', {
limiter: {
max: 100,
duration: 60000 // 100 jobs per minute
}
});
- Repeated Jobs: BullMQ can schedule jobs to be run repeatedly at fixed intervals, similar to a cron job.
myQueue.add({
data: 'Repeat this job every day'
}, { repeat: { cron: '* * * * *' } }); // Cron syntax for every minute
Remember that usage patterns can vary widely depending on the specific needs of your application. These are just some of the highlights of what BullMQ has to offer.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common BullMQ Questions (and Answers)
- What are the differences between BullMQ and Amazon SQS?
- What are the key differences between BullMQ and Agenda?
- What is the difference between BullMQ and RabbitMQ?
- What are the differences between BullMQ and Bull in job queueing?
- What are the differences between BullMQ and Celery?
- How can I use multiple consumers with BullMQ?
- How can I monitor the health of my BullMQ queue?
- How can I use BullMQ for job queue management in Node.js?
- What is the architecture of BullMQ?
- How can you handle errors in BullMQ?
- What are the differences between BullMQ and Kafka?
- What are some best practices for using BullMQ?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
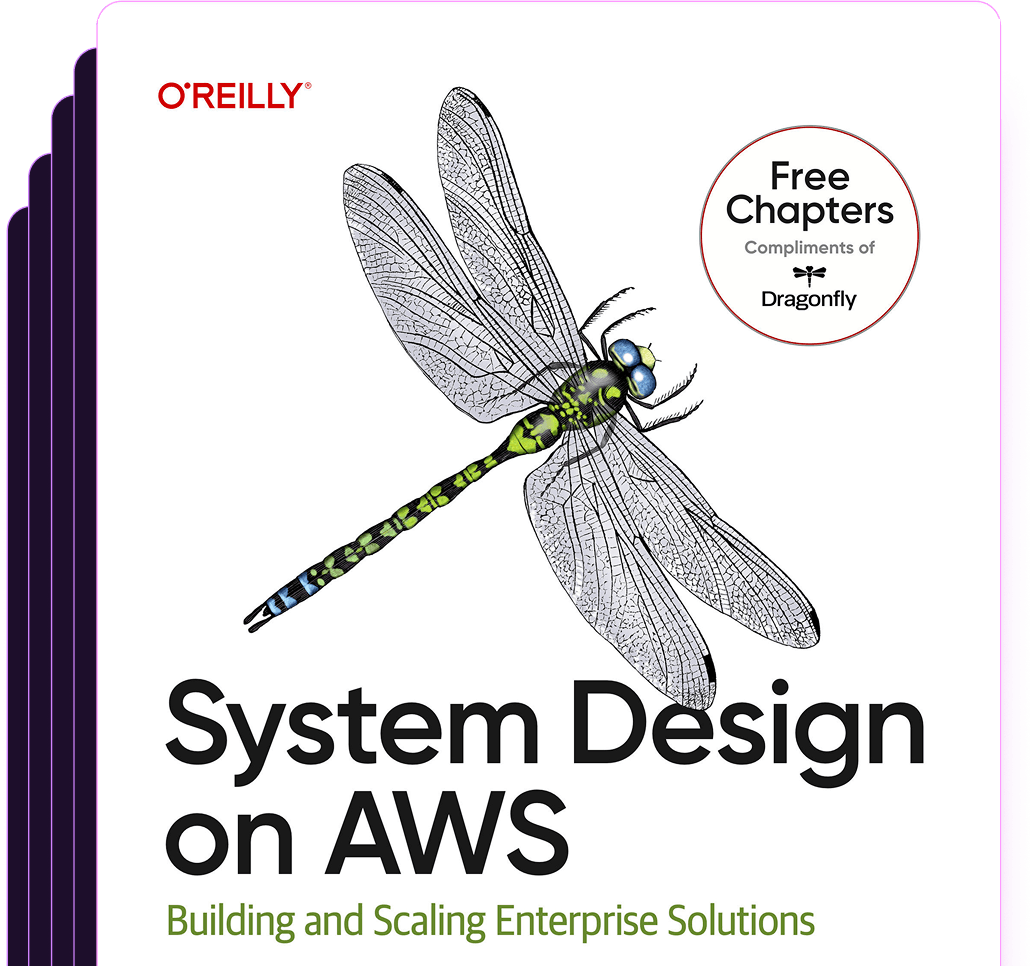
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost