Question: What are the job options in BullMQ and how can they be used?
Answer
In BullMQ, a job is a unit of work that can be processed by a worker. When creating a new job in BullMQ, there are several options you can specify to configure its behavior:
- delay: This option allows you to delay the execution of the job by a certain amount of milliseconds.
- attempts: This option lets you specify the number of attempts to perform if the job fails.
- backoff: This option can be used to control the backoff strategies on retries.
- lifo: If set to
true
, the job will be placed last in the queue (last in, first out). - timeout: This represents the max amount of time in milliseconds a job can be active. If exceeded, the job fails.
- removeOnComplete: If
true
, removes the job when successfully completed. - removeOnFail: If
true
, removes the job when it fails after all attempts. - stackTraceLimit: Limits the amount of stack trace lines stored in case of failure.
Here's an example on how to use these options when adding a job:
const Queue = require('bullmq').Queue;
const queue = new Queue('my-queue');
queue.add(
'my-job',
{ foo: 'bar' }, // Job data
{
delay: 5000,
attempts: 3,
backoff: {
type: 'exponential',
delay: 5000
},
lifo: false,
timeout: 20000,
removeOnComplete: true,
removeOnFail: true,
stackTraceLimit: 5
}
);
This will add a job named 'my-job' with some data, and configure it with the provided options.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common BullMQ Questions (and Answers)
- What are the differences between BullMQ and Amazon SQS?
- What are the key differences between BullMQ and Agenda?
- What is the difference between BullMQ and RabbitMQ?
- What are the differences between BullMQ and Bull in job queueing?
- What are the differences between BullMQ and Celery?
- How can I use multiple consumers with BullMQ?
- How can I monitor the health of my BullMQ queue?
- How can I use BullMQ for job queue management in Node.js?
- What is the architecture of BullMQ?
- How can you handle errors in BullMQ?
- What are the differences between BullMQ and Kafka?
- What are some best practices for using BullMQ?
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
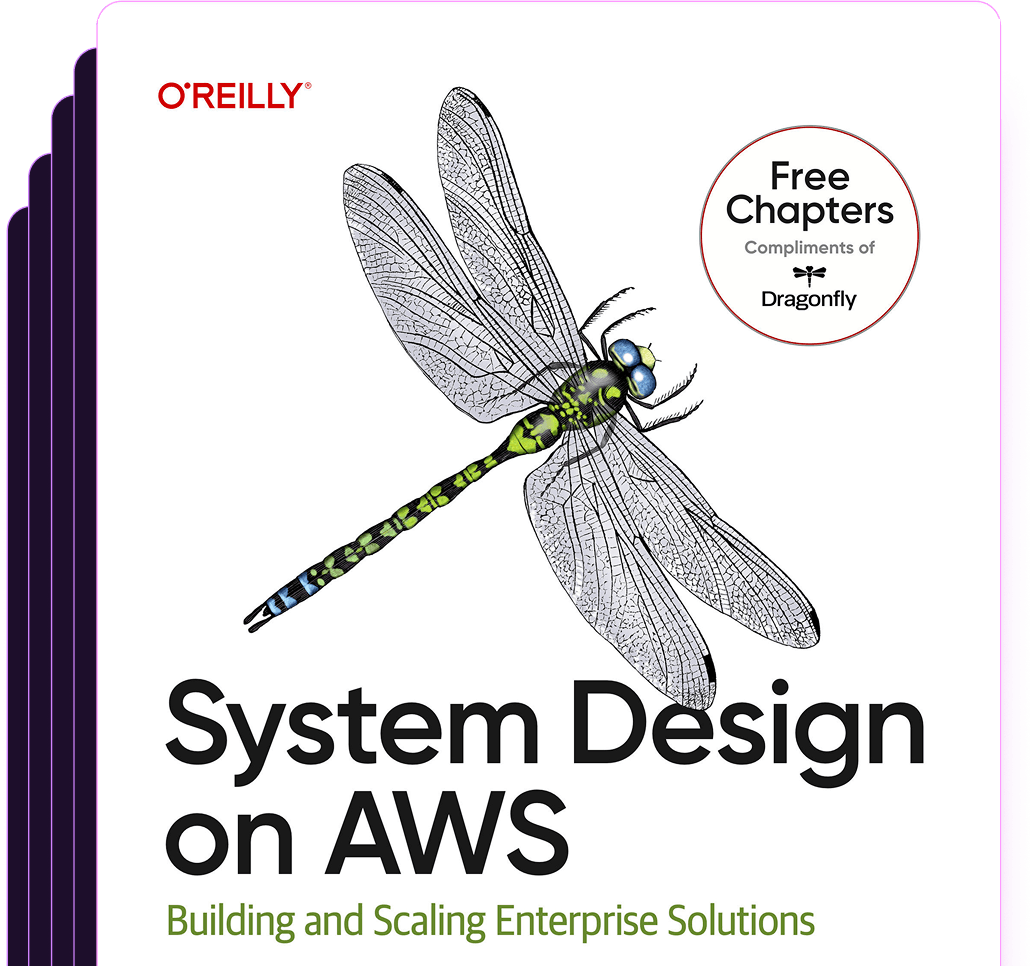
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost