Question: What are BullMQ queue events and how can they be used?
Answer
In BullMQ, a sophisticated Redis-based job queue for Node.js, events are emitted during the lifecycle of jobs. These events can be utilized to monitor job progress or trigger certain actions when something occurs.
Here are some common events:
completed
: Emitted every time a job has been completed successfully.failed
: Emitted when a job fails to execute.stalled
: This is emitted when a job has been stalled. Note that job stalling is a sign of improper shutdown of your processing function.progress
: Emitted when a job updates its progress percentage.waiting
: Emitted when a job is waiting in the queue (i.e., it has been added).active
: Emitted when a job starts to be processed (i.e., moved from waiting state to active).
Here's an example of how you can listen to these events:
const queue = new Queue('my-queue');
queue.on('completed', (job, result) => {
console.log(`Job with id ${job.id} has completed. Result: ${result}`);
});
queue.on('failed', (job, err) => {
console.log(`Job with id ${job.id} has failed with error ${err.message}`);
});
queue.on('progress', (job, progress) => {
console.log(`Job with id ${job.id} is ${progress}% complete.`);
});
Remember, Jobs are atomic and every event is tied to each specific job instance. You should not use these events as a mechanism to issue commands to other processes or as inter-process communication.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common BullMQ Questions (and Answers)
- What are the differences between BullMQ and Amazon SQS?
- What are the key differences between BullMQ and Agenda?
- What is the difference between BullMQ and RabbitMQ?
- What are the differences between BullMQ and Bull in job queueing?
- What are the differences between BullMQ and Celery?
- How can I use multiple consumers with BullMQ?
- How can I monitor the health of my BullMQ queue?
- How can I use BullMQ for job queue management in Node.js?
- What is the architecture of BullMQ?
- How can you handle errors in BullMQ?
- What are the differences between BullMQ and Kafka?
- What are some best practices for using BullMQ?
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
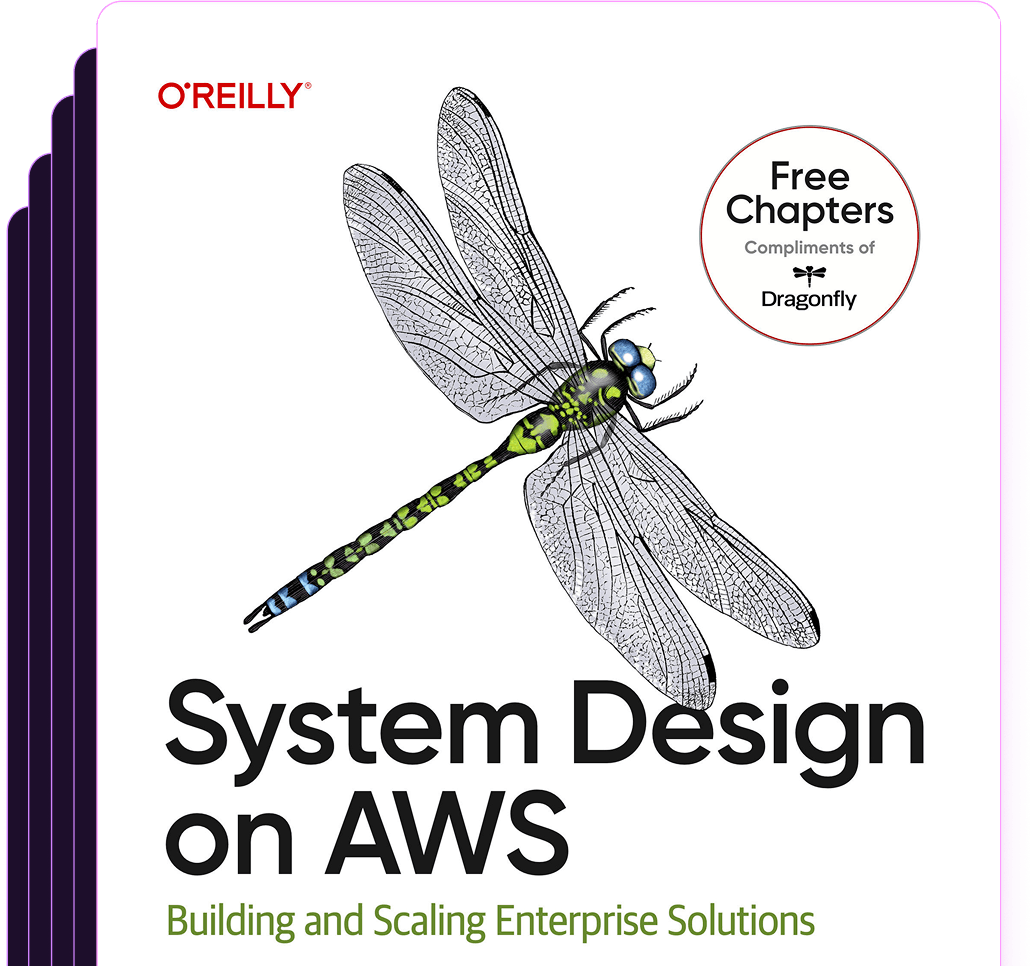
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost