Question: What is the queue scheduler in BullMQ and how does it work?
Answer
BullMQ uses a queue scheduler to manage the delayed jobs. The queue scheduler is a separate process that maintains the correct state of delayed jobs, moving them from the delayed
set to the waiting
list when they are due.
You can initiate a queue scheduler like this:
const { QueueScheduler } = require('bullmq');
const queueScheduler = new QueueScheduler('myQueue');
In the above code, 'myQueue'
is the name of your queue. The queue scheduler will ensure that all jobs in myQueue
with a delay are moved to be processed when their time comes.
However, note that creating a QueueScheduler
instance will also automatically create a Redis connection, so you need to ensure your environment can handle this load.
The queue scheduler is also responsible for maintaining job deadlines and removing stalled jobs.
Please also remember to properly close queue schedulers when they are not needed anymore, preferably using an async exit handler. Here is an example:
const { QueueScheduler } = require('bullmq');
const queueScheduler = new QueueScheduler('myQueue');
process.on('exit', async () => {
await queueScheduler.close();
});
In this way, BullMQ queue scheduler helps efficiently managing the job queue, ensuring jobs run at their specified times, and keeping the queue clean.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common BullMQ Questions (and Answers)
- What are the differences between BullMQ and Amazon SQS?
- What are the key differences between BullMQ and Agenda?
- What is the difference between BullMQ and RabbitMQ?
- What are the differences between BullMQ and Bull in job queueing?
- What are the differences between BullMQ and Celery?
- How can I use multiple consumers with BullMQ?
- How can I monitor the health of my BullMQ queue?
- How can I use BullMQ for job queue management in Node.js?
- What is the architecture of BullMQ?
- How can you handle errors in BullMQ?
- What are the differences between BullMQ and Kafka?
- What are some best practices for using BullMQ?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
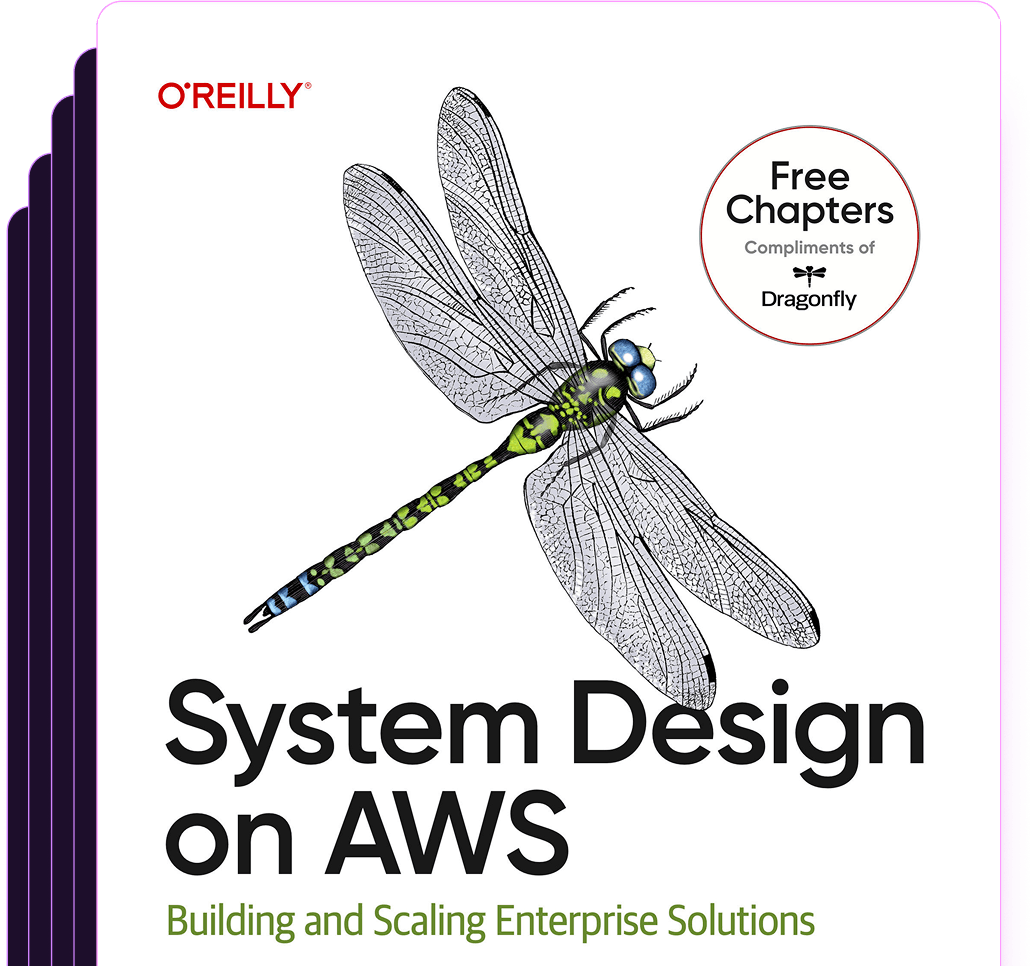
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost