Question: Do Video Games Use Databases?
Answer
Yes, video games often use databases, particularly in the case of larger or more complex games like MMORPGs (Massively Multiplayer Online Role-Playing Games), where tracking player data, game states, inventories, and other information is crucial. Even smaller games may use simple databases or similar structures to store player progress, high scores, and settings.
In large-scale online games, databases are used for a variety of purposes:
- Player Data: This includes account details, characters' stats, inventory items, quest progress, etc.
- Game State: In multiplayer games, the current state of the world, including player locations and statuses of in-game events, is stored in a database.
- Dynamic Content: Some games have content that changes regularly, such as daily quests or rotating stock in in-game stores. This information is often stored and managed through a database.
- Analytics: Game developers collect gameplay data to understand player behavior and tweak the gaming experience. This data is typically stored and processed in a database.
Typically, these databases can be relational databases like MySQL or PostgreSQL, NoSQL databases like MongoDB, or even in-memory databases like Redis for very fast, temporary storage. The choice of database often depends on the specific requirements of the game, including factors like the expected scale of the game, the complexity of the data being stored, and the speed at which the game needs to access the data.
Here's an example of how you might use a database in a game using Python with SQLite:
import sqlite3
# Connect to SQLite database
connection = sqlite3.connect('game.db')
cursor = connection.cursor()
# Create table
cursor.execute('''
CREATE TABLE players (
id INTEGER PRIMARY KEY,
name TEXT,
score INTEGER
)
''')
# Insert a player
cursor.execute('''
INSERT INTO players (name, score) VALUES (?, ?)
''', ('Player1', 1000))
# Commit the transaction
connection.commit()
# Query the database
cursor.execute('''
SELECT * FROM players
''')
for row in cursor.fetchall():
print(row)
# Close the connection
connection.close()
In this basic example, we're creating a simple database for a game with SQLite, adding a player to our database, and then retrieving the player data.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Dev Questions (and Answers)
- Do Game Engines Cost Money?
- Can I Use an SQL Database for Game Development?
- How are databases used in game development?
- How do you save multiplayer game data, in a database or a file?
- How can you design an efficient database for a game?
- Should I Use Redis or MySQL for Game Development?
- What are the differences between using a database and JSON for games?
- Does game development require knowledge of mathematics?
- Should I Use a Game Engine or Not?
- Is a game engine considered a framework?
- Do you need a game engine to make a game?
- How can you design a game leaderboard system?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
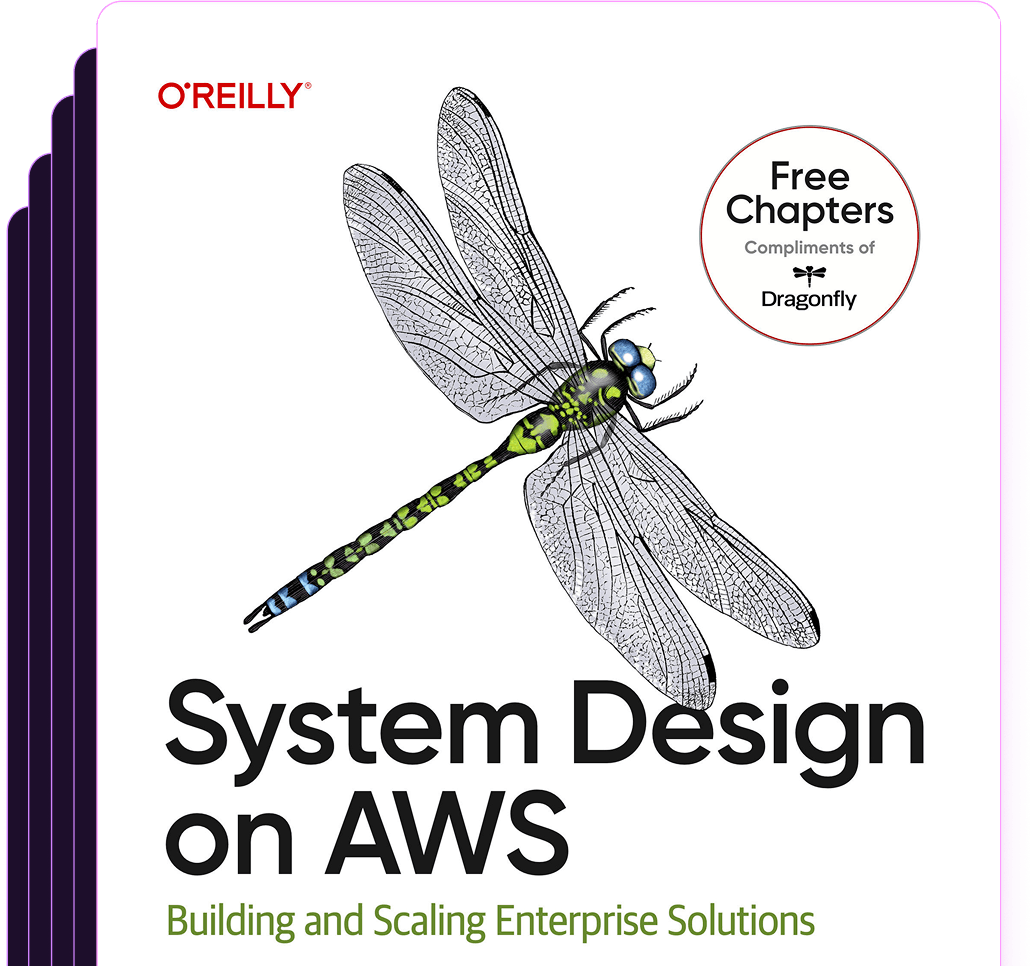
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost