Question: How do you save multiplayer game data, in a database or a file?
Answer
The choice between saving multiplayer game data into a database or a file depends on the specifics of your game and its requirements.
- Local Files - Local files are typically used for single-player games where all game data can be stored locally. This method is simple and works well for storing player progress, preferences, and game states. However, it's not usually a good fit for multiplayer games because sharing and synchronizing data between players can become hard.
# Example of saving to a local file in Python
with open('game_save_data.json', 'w') as f:
json.dump(game_data, f)
- Databases - Multiplayer games often use databases (SQL or NoSQL) to store game state, player data, inventory, world states, and similar data. Databases offer several advantages over local files, such as concurrent access, better data integrity, scalability, and structured data querying abilities. For instance, MongoDB, a popular NoSQL database, provides flexibility with its JSON-like documents.
# Example of saving to a MongoDB database in Python
from pymongo import MongoClient
client = MongoClient('mongodb://localhost:27017/')
db = client['game_database']
collection = db['game_save_data']
collection.insert_one(game_data)
- Server-side Files - In some cases, server-side files could be used as a middle ground when the data needs to be shared among multiple players but doesn't require a full-fledged database system. This method still may have issues with concurrent access and data consistency.
- Cloud Services - Services like Amazon S3 or Google Cloud Storage can also be used for storing game data, especially for large files like user-generated content.
Remember to ensure that all sensitive player data is appropriately secured and encrypted if you're storing it, and adhere to all relevant data protection laws.
In a nutshell, while local or server-side files might suffice for small-scale, simple multiplayer games, a database is often the most suitable choice for larger, more complex games.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Dev Questions (and Answers)
- Do Game Engines Cost Money?
- Can I Use an SQL Database for Game Development?
- How are databases used in game development?
- How can you design an efficient database for a game?
- Should I Use Redis or MySQL for Game Development?
- What are the differences between using a database and JSON for games?
- Do Video Games Use Databases?
- Does game development require knowledge of mathematics?
- Should I Use a Game Engine or Not?
- Is a game engine considered a framework?
- Do you need a game engine to make a game?
- How can you design a game leaderboard system?
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
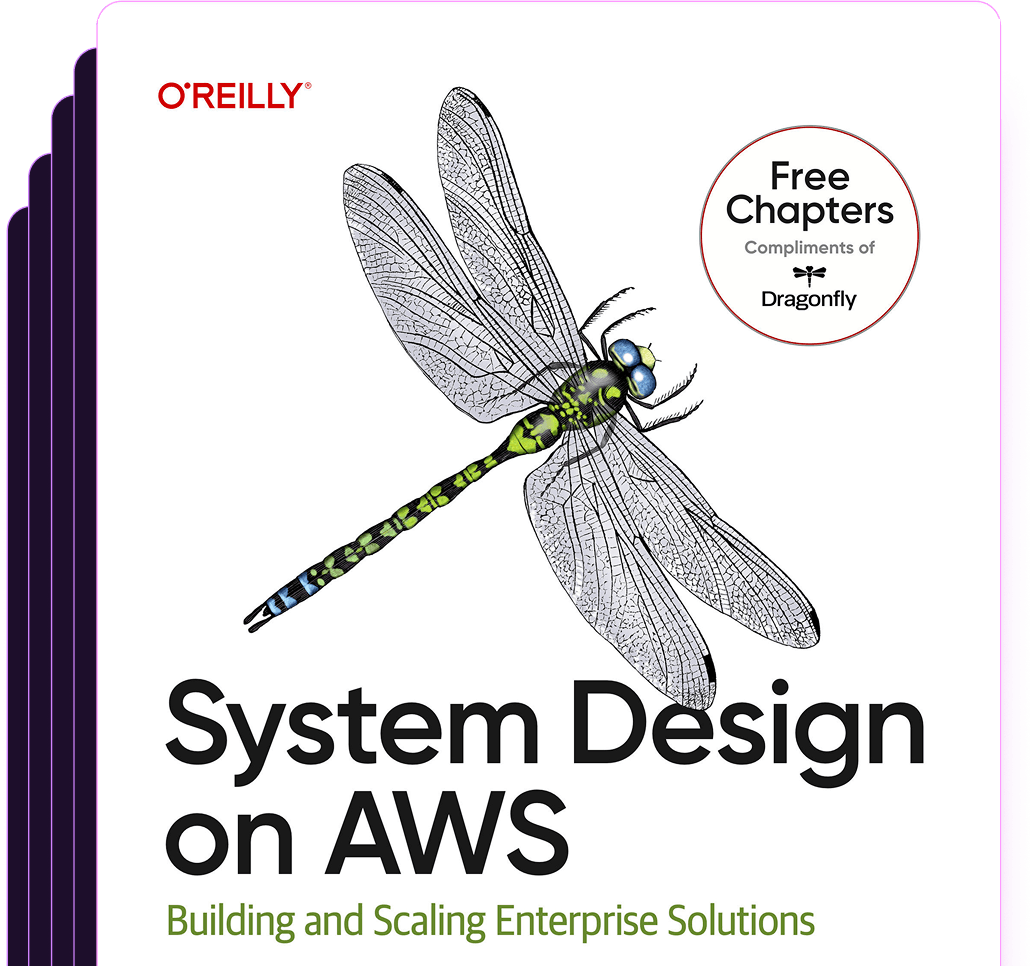
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost