Question: How can I effectively use BullMQ for production environments?
Answer
BullMQ is a powerful and versatile job queue library for Node.js. It's based on Redis, which means it can handle heavy loads and is well-suited for production environments. Here are some key points to consider when using BullMQ in a production setting:
- Proper Connection Handling: Make sure to properly handle connections with your Redis instance. This includes setting up retry strategies and handling connection loss.
const client = new Queue('my-queue', {
connection: {
host: '127.0.0.1',
port: 6379,
// Custom retry strategy
retryStrategy: function(times) {
return Math.min(times * 50, 2000);
}
}
});
- Queue Priority: If you have different types of jobs where some need to be processed before others, you can assign them different priority levels.
await myQueue.add('high priority job', {foo: 'bar'}, {priority: 1});
await myQueue.add('low priority job', {baz: 'qux'}, {priority: 3});
- Rate Limited Queues: For tasks that require rate limiting (like sending emails), BullMQ provides an easy way to manage this.
const emailQueue = new Queue('email', {
limiter: {
max: 100, // Max 100 jobs per interval
duration: 60000 // Every minute
}
});
- Job Events and Progress: Let's not forget the importance of monitoring your jobs. Job events and progress indicators can help trace issues if they appear.
myQueue.on('completed', (job, result) => {
console.log(`Job ${job.id} completed with result: ${result}`);
});
- Error Handling: Implement appropriate error handling at both the job level and queue level.
// Job level
myQueue.process(async (job) => {
try {
// Process job here
} catch (error) {
console.error(`Job failed with error: ${error}`);
throw error;
}
});
// Queue level
myQueue.on('failed', (job, err) => {
console.log(`Job ${job.id} failed with error: ${err.message}`);
});
- Redundancy and Fault Tolerance: Ensure that your Redis server is set up in a way that provides redundancy and fault tolerance. This could mean using a managed service that provides automatic failover or setting up your own Redis Sentinel or Cluster configuration. Note that BullMQ supports Redis Sentinel out of the box.
Remember to always thoroughly test your queue logic before deploying it into production to ensure it behaves as expected under different edge cases.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common BullMQ Questions (and Answers)
- What are the differences between BullMQ and Amazon SQS?
- What are the key differences between BullMQ and Agenda?
- What is the difference between BullMQ and RabbitMQ?
- What are the differences between BullMQ and Bull in job queueing?
- What are the differences between BullMQ and Celery?
- How can I use multiple consumers with BullMQ?
- How can I monitor the health of my BullMQ queue?
- How can I use BullMQ for job queue management in Node.js?
- What is the architecture of BullMQ?
- How can you handle errors in BullMQ?
- What are the differences between BullMQ and Kafka?
- What are some best practices for using BullMQ?
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
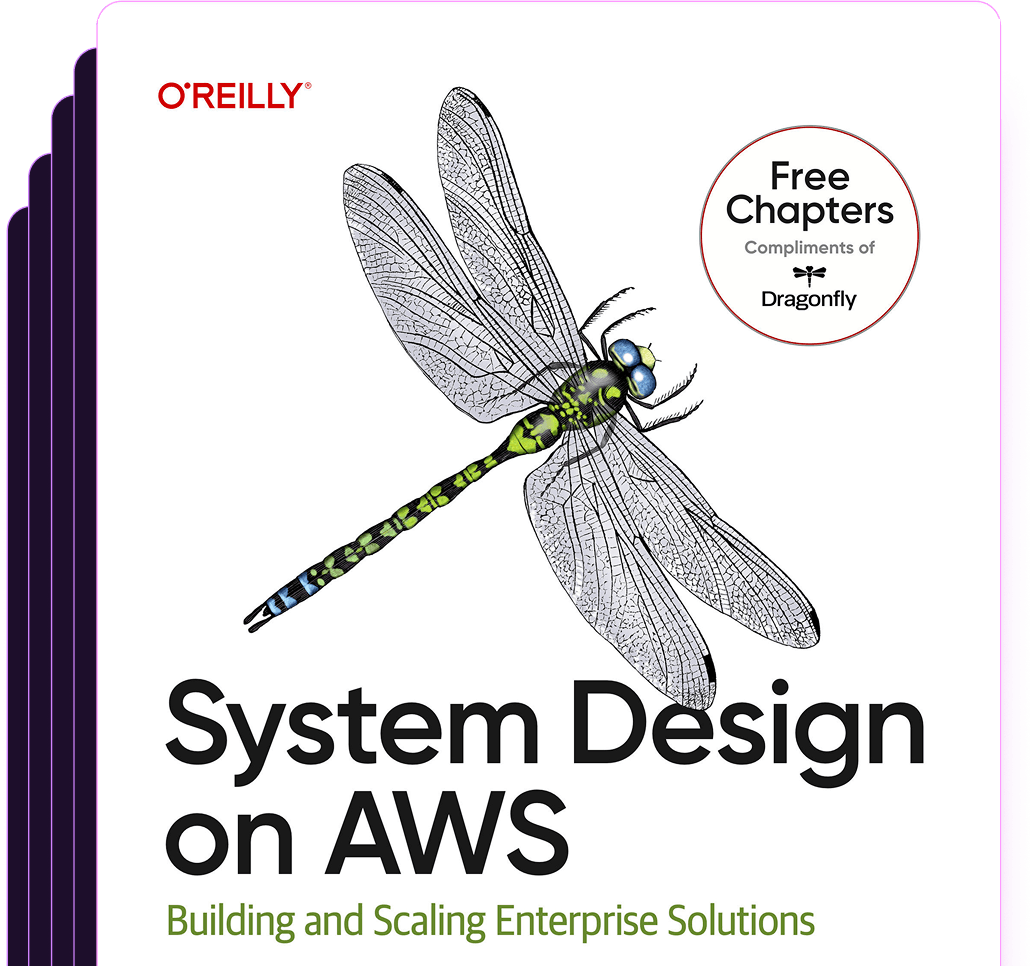
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost