Question: How can you integrate BullMQ with Amazon SQS (Simple Queue Service)?
Answer
BullMQ is a job and message queue that relies on Dragonfly or Redis for storage. It offers robust features such as priority-based queues, delayed jobs, scheduling, and more although it does not directly integrate with Amazon Simple Queue Service (SQS), which is a fully managed message queuing service by AWS.
However, you could create a workaround system where an intermediate service reads from the BullMQ queue and writes to an Amazon SQS queue, or vice versa. Here's a simplified pseudocode example:
const Queue = require('bullmq').Queue;
const AWS = require('aws-sdk');
AWS.config.update({region: 'REGION'}); // your region
// setup bull queue
const myBullQueue = new Queue('myBullQueue');
// setup sqs
const sqs = new AWS.SQS({apiVersion: '2012-11-05'});
const queueURL = 'SQS_QUEUE_URL'; // your SQS queue URL
// listen to completed jobs from bullmq and push them to sqs
myBullQueue.on('completed', (job, result) => {
const params = {
MessageBody: JSON.stringify(result),
QueueUrl: queueURL
};
sqs.sendMessage(params, (err, data) => {
if (err) {
console.log('Error', err);
} else {
console.log('Successfully added to SQS', data.MessageId);
}
});
});
Remember that this is an oversimplified example. In a real-world application, you'd need to handle errors robustly, deal with job failures, and possibly use a more efficient method of transferring jobs between queues such as batch processing. Furthermore, the kind of architecture you choose (pushing from BullMQ to SQS or pulling from BullMQ to SQS) will depend on your specific use case.
Lastly, while this example demonstrates how you might integrate BullMQ and SQS, it's important to consider the architectural implications of doing so. If you're using both BullMQ and SQS in the same application, it might be worth reconsidering whether both are needed or if one would suffice."
Was this content helpful?
Help us improve by giving us your feedback.
Other Common BullMQ Questions (and Answers)
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
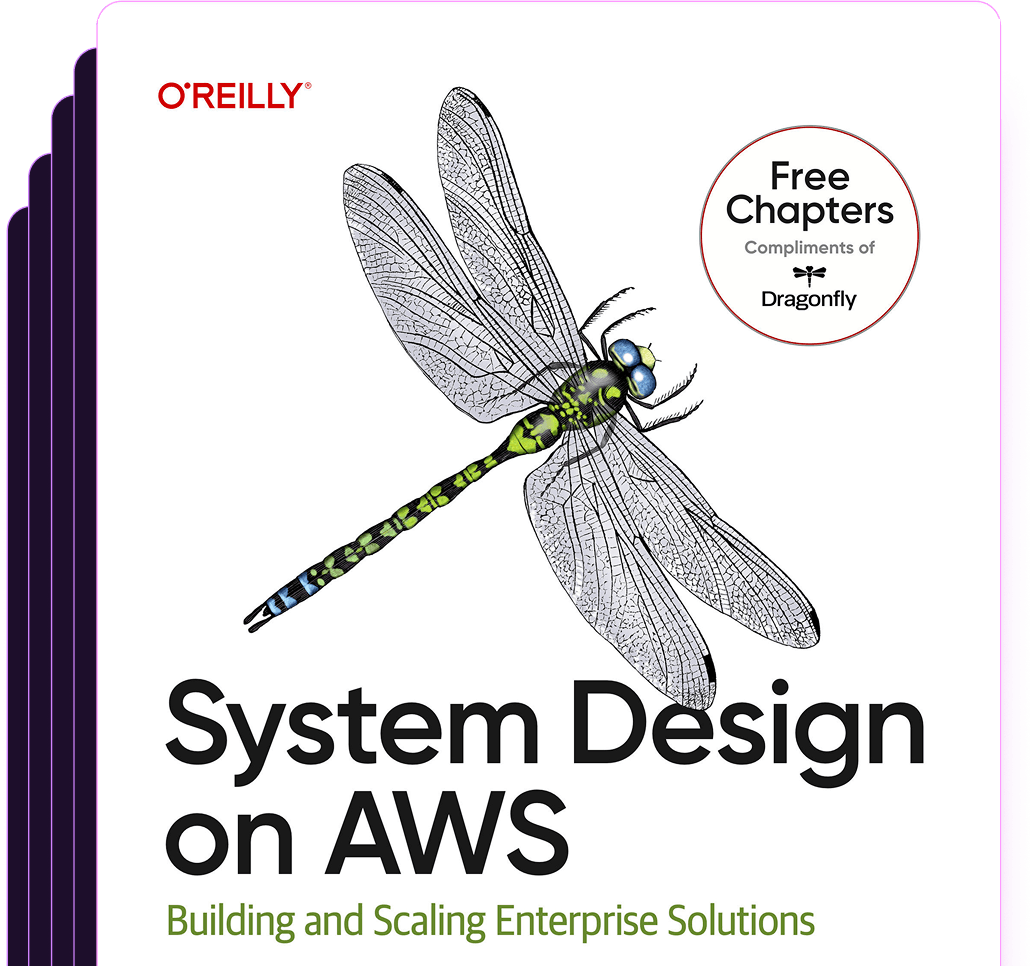
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost