Question: How can I maximize throughput in BullMQ?
Answer
To maximize throughput in BullMQ (a Node.js library for handling jobs and messages in a distributed manner), you need to consider several factors: the job processing rate, the level of parallelism, network latency, as well as the power and capabilities of your hardware. Here are some strategies to optimize these:
- Job Processing Rate: The speed at which jobs get processed directly affects throughput. If possible, make your job handlers more efficient by optimizing code and reducing database query time.
const queue = new Queue('my-queue');
queue.process(async (job) => {
// Optimize this function to process jobs faster.
});
- Parallelism: BullMQ supports processing multiple jobs concurrently. You can increase throughput by increasing parallelism. However, be aware that too many concurrent jobs may overload your system or database.
// Process up to 5 jobs concurrently.
queue.process(5, async (job) => {
// Job processing logic here.
});
- Network Latency: If your BullMQ setup includes separate instances communicating over a network, latency may impact throughput. Consider using a high-speed network or locating your processors closer to your Redis instance.
- Hardware Power: Throughput can also be limited by your system's CPU, memory, storage speed, and network bandwidth. For maximum throughput, use more powerful hardware or scale out across multiple machines.
Remember, these are generic tips. For the best performance, you should benchmark different configurations and fine-tune based on your specific workload and environment.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common BullMQ Questions (and Answers)
- What are the differences between BullMQ and Amazon SQS?
- What are the key differences between BullMQ and Agenda?
- What is the difference between BullMQ and RabbitMQ?
- What are the differences between BullMQ and Bull in job queueing?
- What are the differences between BullMQ and Celery?
- How can I use multiple consumers with BullMQ?
- How can I monitor the health of my BullMQ queue?
- How can I use BullMQ for job queue management in Node.js?
- What is the architecture of BullMQ?
- How can you handle errors in BullMQ?
- What are the differences between BullMQ and Kafka?
- What are some best practices for using BullMQ?
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
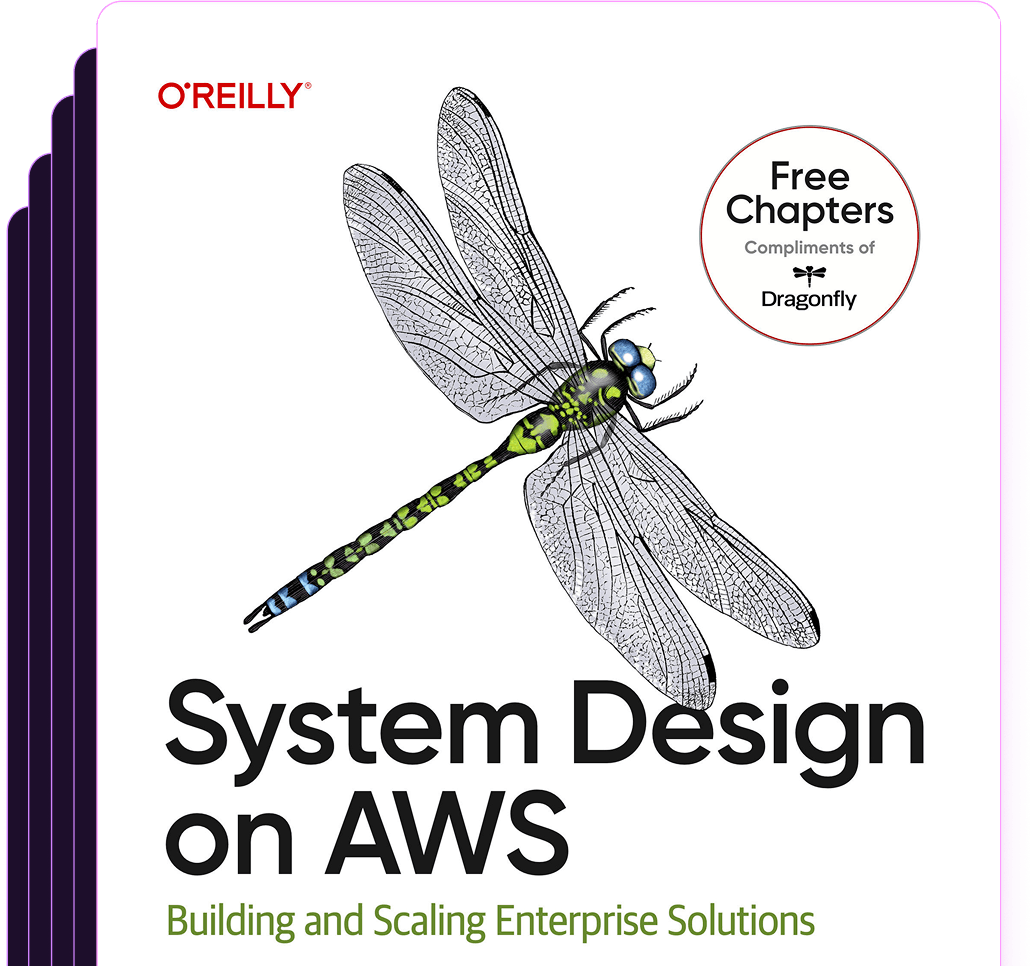
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost