Question: How can I track the status of a job in BullMQ?
Answer
In BullMQ, you can check the status of a job by using its getState
method. This will return the current state of the job in the queue.
const Queue = require('bullmq').Queue;
const queue = new Queue('my-queue');
async function getJobStatus(jobId) {
const job = await queue.getJob(jobId);
if (job === null) {
console.log('No job found with id ' + jobId);
} else {
const state = await job.getState();
console.log('Job state is: ' + state);
}
}
getJobStatus('some-job-id');
In this example, getJobStatus
function takes a jobId
as an argument and retrieves the job from the queue. If the job exists, it gets the job's state using getState()
. The potential states of a job in BullMQ are: completed
, failed
, delayed
, active
, waiting
, paused
, or stuck
.
Please note that getJobStatus
is an asynchronous operation because it needs to communicate with the Redis server where your jobs are stored. Therefore, it should be used inside an async function or handled with promise-based syntax.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common BullMQ Questions (and Answers)
- What are the differences between BullMQ and Amazon SQS?
- What are the key differences between BullMQ and Agenda?
- What is the difference between BullMQ and RabbitMQ?
- What are the differences between BullMQ and Bull in job queueing?
- What are the differences between BullMQ and Celery?
- How can I use multiple consumers with BullMQ?
- How can I monitor the health of my BullMQ queue?
- How can I use BullMQ for job queue management in Node.js?
- What is the architecture of BullMQ?
- How can you handle errors in BullMQ?
- What are the differences between BullMQ and Kafka?
- What are some best practices for using BullMQ?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
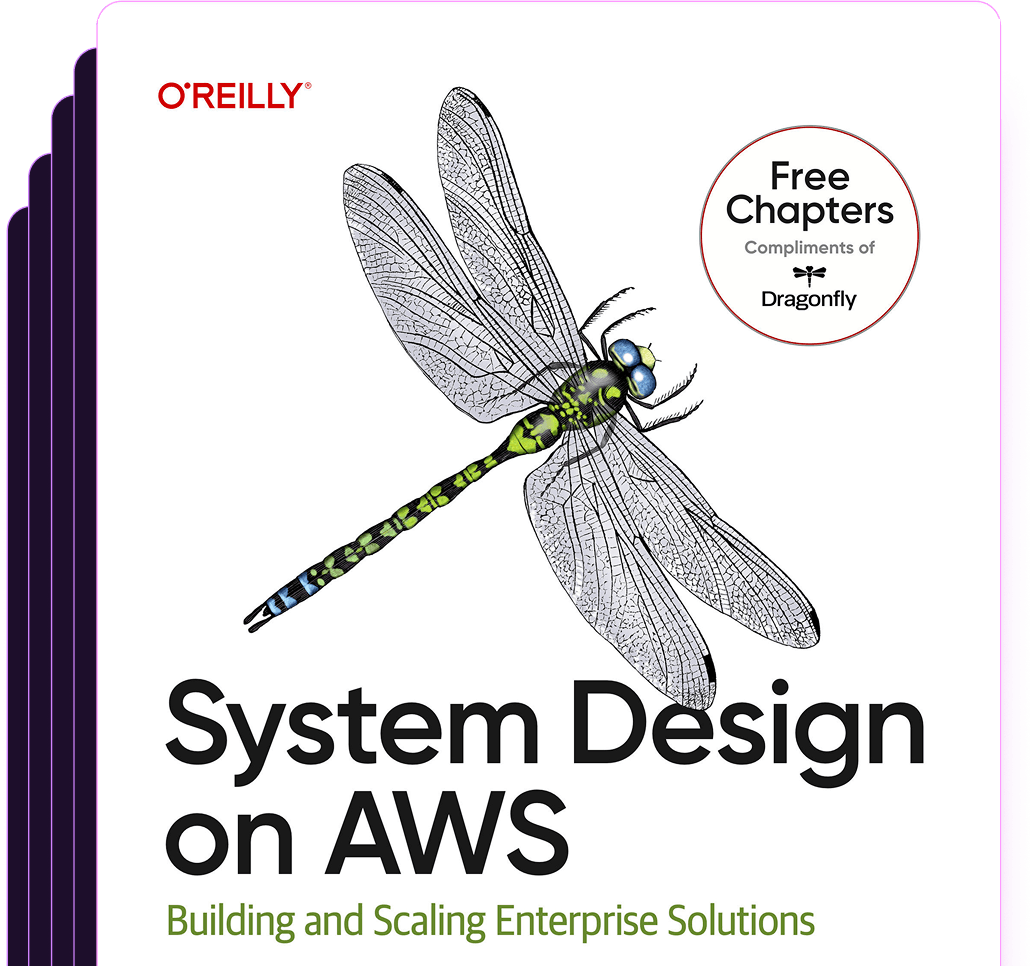
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost